Dynamic Members
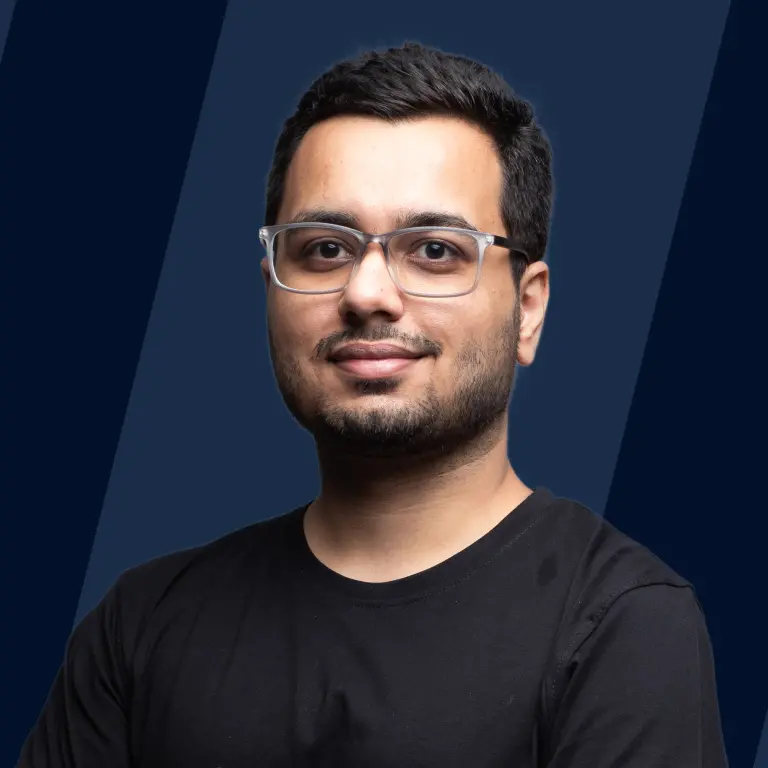
Overview
Dynamic Member is a concept of implementation of user-defined data types which can shrink or grow according to user needs at the runtime of the program. The core concept which allows us to create some data containers of variable length is the dynamic memory allocations in C++ programming. In general, a pointer to locate the memory blocks, a number count which can represent how much data is stored in the variable, and a maximum size that will indicate that these many elements can be inserted without making any changes to the data container is used in dynamic members.
Dynamic Member Class Template
The normal template of class on which we are going to work will look like this,
In the further article, the constructor, destructor, methods etc will be added in this class to make it functional Dynamic Member. The elaborated description of each is given in the article.
Constructor
When we start to work along with the objects, the constructor should be the first thing to think about because they are responsible for creating objects only after we can manipulate them. There can be majorly 4 types of the constructor,
1. No parameter is provided to constructor
Here the default constructor will execute to create an object with the maximum size and count as 0. Normally, the point to create this type of object is relying on the fact that later the program may increment the maximum size.
2. The number of elements are provided
The constructor will create an object with the maximum size as a number of elements provided as a parameter, to avoid the problem of garbage it will be filled via memset.
3. The number of elements along with the value for each block is provided
The constructor will not only create the data blocks but also fill them with a provided value.
4. The object of same class is provided
It will be a copy constructor to execute the functionality which can create the object as well as copy the data of provided object into the newly created one.
Destructor
Before we move on to further functionality, it is necessary to talk about the deletion of objects because it's a good software practice to maintain and delete all unnecessary data. If the object is going to be deleted, then somehow, we need to release all the memory pointed by our object.
You might know from the concepts of dynamic members allocation in C++, the delete keyword deletes the memory locations pointed by the pointer written after the delete keyword.
Access & Manipulation of Data Element
This is an interesting part of dynamic members because after all, we are supposed to access our data in an efficient manner. So here, we will create some functionality to insert, access, and manipulate our data.
Append
This method will be used to append the data at the end.
Pop
This method will pop and return the element corresponding to last data block.
Remove
This method will remove the element according to the given index and will shift the all data to the left after removal.
Operator overloading
[] operator
In general, for the primitive arrays, we access the data by providing an index inside the [] operator because the language constructs have some code written for this accessibility but for our user-defined data type, we must have to overload this operator.
= operator
This assignment operator overload will be used if an object of this user-defined data type is being assigned to another so that it can copy the data of the assigned object to the one which is on the left side. Although the assignment functionality of shallow copy is injected in the code automatically by the compiler but in this case, both objects will reference the same data locations and changes made by any of the objects will appear in both. That's why in the overload, we are making a slight change by creating a new data set of elements i.e deep copy.
Varying Size of Container
Now, after this discussion, the point is how to vary the size of the container i.e. decrease or increase according to user need at runtime. The concept here is to create a new container of more size than the existing one. This number "more" could be anything according to the program author i.e. vector implementation doubles the size each time when the user requests to enter more data and in a similar way reduces the size by half if the block of elements becomes empty due to removal of elements.
In our example, we are going to increase or decrease the size by 10.
Let's re-write the append method by including the case of full data container.
Similarly we will have to write the functionality to decrease the maximum size in remove and pop method.
The same kind of procedures will be inserted in the pop method so that if the number of empty blocks are greater than 10 we can release that space.
Examples
When we combine all methods, constructors and destructors, we can use this class to create our user defined variables which is nothing but a dynamic integer array, in that integer array we can perform several operations according to class definition:
- Create an object with the help of various constructors.
- Append some data from the end of object's dynamic array via append method.
- Access any index of the object's dynamic array with the help of operator overload.
- Remove any index value, also the last index value by using remove and pop method.
- One thing which is different from array is, here it will grow and shrink at runtime according to the functionality written in the append, remove and pop method.
Here we'll explore some examples to test each method written above.
1. Object Creation
Output:-
Explanation:- The A1, A2, and A3 objects will be created according to the constructor defined in the class definition. The getMaxSize() method returns the maximum size of the container, and getCount() returns the number of actual data blocks in the container.
2. Use of Operator[] and Append Method
Output:-
Explanation:- Initially, we have A1 as an empty object means it doesn't contain any memory blocks. So the getMaxSize() and getCount() both method will return 0. When we call the append method on A1, the append method will investigate the number of remaining memory blocks, which is zero now. Conditionally, it will create ten more memory blocks according to the function definition. After the allocation of sufficient memory blocks, the append method will store 10, 20, and 30. Concurrently the count will also increase at each successful append method call.
3. Working of Remove & Pop
Output:-
Explanation:- In the starting, we have created an object A4 of size 15, all initialized with value 3. The getCount() and getMaxSize() both will return 15. When we try to pop more than 10(let's say 12) data blocks from the dynamic array, it will shrink according to function definition, i.e. size will be decreased by 10. Now, the total element will become 3, and the maximum size will become 5.
Output:-
Explanation:- Initially, we have an empty object A5; the append method inserted the 4 data elements. Consequently, its element count will become 4, and the maximum size will become 10. The functionality of the remove method is the same as the pop method, the difference is it can remove the element of any index and shift the remaining ones to the left. After removing the element of the 3rd index the count will be decremented by 1.
4.Use of Assignment Operator Overload
Output:-
Explanation:- At the starting of the code snippet, we have created two empty objects, and then the append method is used four times to insert some data to A5. Later, A5 is being assigned to A6, due to assignment operator overload A5 object is being copied to A6.
Conclusion
- When the size of data is not constant at the time of creation of data container, The dynamic members comes into the picture.
- They provide a nicer way to cope with this problem of size prediction, So that you need not to worry about the increasing size of data.
- If the size of container is less to accommodate incoming data, we can somehow allocate some extra data blocks to insert this new data i.e discussed in the append method.
- If the size of container is much more than the actual data, we can delete the allocation of those extra data blocks to for memory optimization i.e discussed in the pop and remove method.
- The Dynamic Members are better in comparison to the static members i.e. normal array because they provide flexibility to increase or decrease the size according to needed memory blocks.