Jump Statement in C++
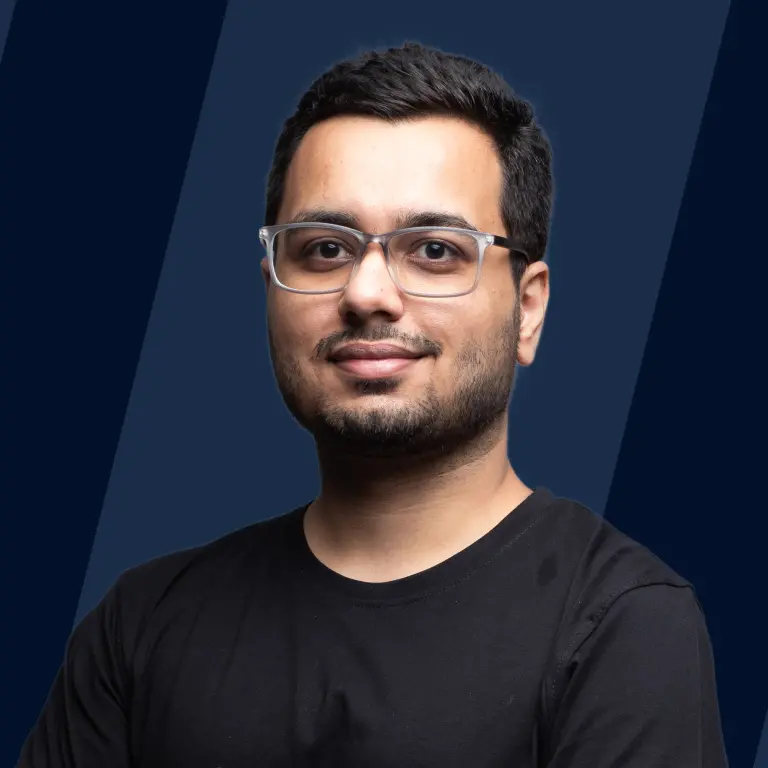
Overview
A C++ control statement diverts the flow of a program to compile a supplementary code. Decision-making, iterative, and jump statements are the basic types of control statements in C++.
Jump statements are used to shift the program control from one part to any other part of the program unconditionally when encountered. continue, break, return, and goto statements are used to implement the jump statements in C++.
What are Control Statements in C++
A C++ control statement diverts the flow of a program to compile a supplementary code. These statements consist of conditional statements such as if-else and switch and loop statements such as for, while, and do-while. Each of them executes a logical condition and returns a boolean value to check for the execution of a piece of code.
Types Of Control Statements In C++
There are three types of control statements in C++ :
- Decision Making Statements
- Iterative Statements
- Jump Statements
1. Decision-making Statements
Decision-making statements use conditional statements on which the execution of a particular code is dependent. If the conditional statement returns true, the code will get executed, and the cursor will skip the code if false is returned. In C++, the decision-making statements are implemented using if-else, if-else ladder, nested if, switch statements, etc.
2. Iterative Statements
Iterative statements run a piece of code recurrently until a base condition is met. These statements contain a variable upon which the condition depends.
The variable's value gets changed after every iteration, and the condition is checked corresponding to the new value. In C++, the iterative statements are implemented using for, while, and do-while loops.
3. Jump Statements
Jump statements are used to obstruct the normal flow of execution of a program. It shifts the program control from one part to any other part of the program unconditionally when encountered. In C++, the jump statements are implemented using break, continue, goto, and return. break and continue are used in loops or iterative statements, while, goto, and return can be used anywhere in the program.
A standard library function exit() is also provided in C++ to stop the further execution of the program.
Types Of Jump Statements In C++
Jump statements in C++ mainly have four types :
- Continue statements
- Break statements
- Goto statements
- Return statements
1. Continue Statements
The continue statements are used within loops or iterative statements. The objective of the continue statement is to skip the remaining statements of the current iteration of the loop and move on to the next iteration.
The flow chart of the continue statement is illustrated in the following diagram:
Steps involved in the execution of the continue statement:
- The program control executes every statement of the loop sequentially.
- A conditional statement containing a continue statement is encountered.
- If the conditional statement returns true, then the control enters its body.
- In the body of the condition statement, the continue statement occurs, which immediately transfers the control to the starting point of the loop for the next iteration.
- If the conditional statement returns false, then the remaining loop statements for the current iteration will be executed sequentially.
A program to show the implementation of the continue statement in C++ :
Output:
Explanation:
The above program aims to provide odd numbers between 1 to 10. The code consists of a while loop having a variable i initialized with 0. The variable i gets incremented and checks whether it is even or not by equating the remainder, which comes up when the current i is divided by 2, with 0. If i turns out to be even, then the continue statement will be executed, which skips the current iteration and starts the next one. If the variable i is odd, then it will get printed.
2. Break Statements
The break statements, like continue statements, are also used within loops or iterative statements. The objective of the break statement is to stop the execution of the loop and transfers the program control to the statement just after the loop.
It not only skips the execution of the remaining statements of the current iteration but all other remaining iterations of the loop also remain untouched by the program control.
The flow chart of the break statement is illustrated in the following diagram:
Steps involved in the execution of the break statement:
- The program control executes every statement of the loop sequentially.
- A conditional statement containing a break statement is encountered.
- If the conditional statement returns true, then the control enters its body.
- In the body of the condition statement, the break statement occurs, which immediately throws the control outside the loop.
- If the conditional statement returns false, then the remaining statements and iterations of the loop will be executed sequentially.
A program to show the implementation of the break statement in C++ :
Output:
Explanation:
The code consists of a while loop that runs from 0 to 9. A conditional statement in the loop checks whether the current value of i is greater than 5. If the conditional statement returns true, then the break statement will be executed, which ceases the further execution of the loop, and the control comes out of the loop. If the conditional statement returns false, all the remaining statements and iterations of the loop will be executed sequentially.
3. Return Statements
The return statements can be used in any part of the function. The objective of the return statement is to stop the execution of the current function and transfers the program control to the point from where it has been called.
If a return statement is used in the main function, it will stop the compilation process of the program. The return statement is also used to transfer a computed value of the function that belongs to a predefined return type to the variable used to call the function.
The flow chart of the return statement is illustrated in the following diagram:
Steps involved in the execution of return statement:
- The program control executes every statement of the function sequentially.
- A return statement is encountered with or without a variable of the predefined return type.
- The return statement is executed with the transfer of the program control to the point from where it has been called.
- If the return type of the function is not void, then a variable is used to call the function and stores the resultant value returned from the function.
A program to show the implementation of the return statement in C++ :
Output :
Explanation:
The code initializes a variable that calls a function with two arguments of integer type. The function adds the two values and stores them in a variable. The return statement is then executed, which transfers the program control along with the resultant value to the variable from where it has been called.
4. Goto Statements
Like the return statements, the goto statements can be used in any part of a function. The objective of the goto statement is to jump directly to any part of the function. The goto statement hits the program control to the part of the program defined by a label.
A label acts as an argument to the goto statement and an identifier, initiating with a colon(:), defining a piece of code. The goto statement and the label should be in the same function in a C++ program.
A label can be placed anywhere in the program, irrespective of the position of the goto statement. If the label is placed after the goto statement, it is said to be a forward reference. If the label is placed before the goto statement, it is said to be a backward reference.
The flow chart of the goto statement is illustrated in the following diagram:
Diagram to show forward and backward reference in the goto statement:
Steps involved in the execution of the goto statement:
- The program control executes every statement of the function sequentially.
- A goto statement is encountered with a label pointing to another code.
- The goto statement is executed with the transfer of the program control to the label.
- The program control starts executing the statements under the provided label.
A program to show the implementation of the goto statement in C++ :
Output :
Explanation:
The code prints numbers from 1 to 5 with the help of 2 goto statements. One of the goto statements calls label1 if the value of i is less than 10. The label1 is present above the goto statement, so backward reference occurs. The other goto statement calls label2 if the value of i is greater than 4. The label2 is placed below the goto statement, and henceforward reference occurs.
Should the Goto Statement Be Used?
The goto statements disturb the flow of a program and hence convert it into a complicated code to interpret and modify. It threatens a programmer while repairing the code by removing bugs and adding required features.
It also provokes problems related to scope accessibility. There are many cases where we have options in place of using the goto statement. The Goto statement can be best used to get out from the nested loops where the break statement only leaves the inner loop.
Conclusion
- A C++ control statement diverts the flow of a program to compile a supplementary code.
- Jump statements are used to shift the program control from one part to any other part of the program unconditionally when encountered.
- continue, break, return, and goto statements are used to implement the jump statements in C++.
- A continue statement skips an iteration of the loop, while a break statement stops the execution of the loop when encountered.
- A return statement stops the execution of the current function in a program while goto shifts the program control to a part of the program defined by a label.