Multiple Inheritance in C++
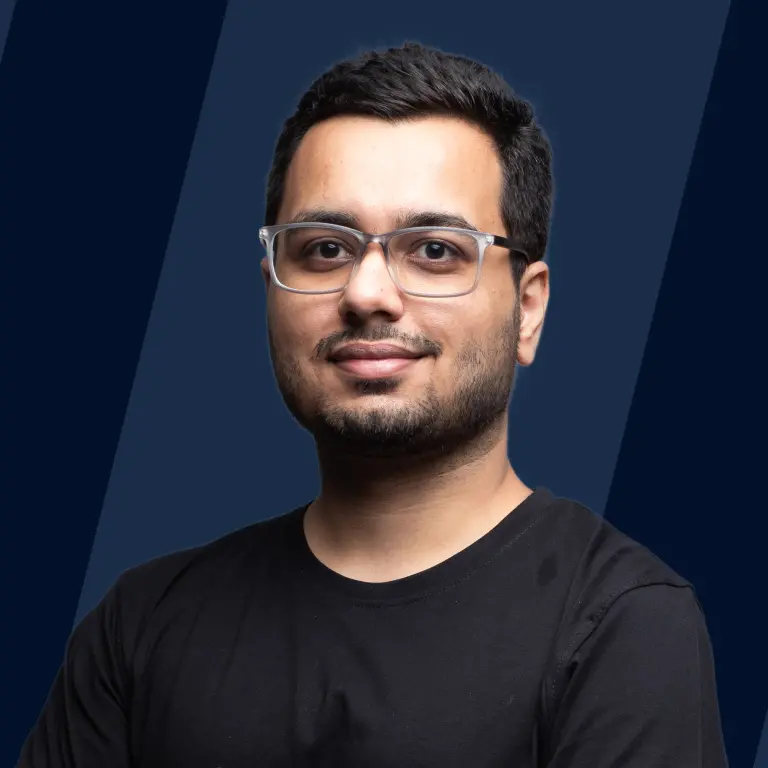
Overview
The capability of a class to derive properties and characteristics from another class is called Inheritance. This allows us to reuse and modify the attributes of the parent class using the object of the derived class.
The class whose member functions and data members are inherited is called the base class or parent class, and the class that inherits those members is called the derived class.
Introduction to Multiple Inheritance
Multiple Inheritance is the concept of inheritance in C++ by which we can inherit data members and member functions from multiple(more than one) base/parent class(es) so that the derived class can have properties from more than one parent class.
In the image above, the derived class inherits data members and member functions from two different parent classes named Base Class 1 and Base Class 2.
For an analogy, we can take an example of a flying car, a ground vehicle, or a flying vehicle. It can be seen that it has inherited the properties of both the ground vehicle and the flying vehicle.
Diagram of Multiple Inheritance in C++
Syntax of Multiple Inheritance in C++
Here, there are two base classes: BaseClass1 and BaseClass2. The DerivedClass inherits properties from both the base classes.
While we write the syntax for the multiple inheritance, we should first specify the derived class name and then the access specifier (public, private, or protected), and after that, the name of base classes.
We should also keep in mind the order of the base classes given while writing the multiple inheritance because the base class's constructor, which is written first, is called first.
Example
As the order while writing the multiple inheritance was BaseClass2 and then BaseClass1, the constructor will be called in that order only.
Output
Now, changing the order.
Output:
Ambiguity Problem in Multiple Inheritance
In multiple inheritance, we derive a single class from two or more base or parent classes.
So, it can be possible that both the parent classes have the same-named member functions. While calling via the object of the derived class(if the object of the derived class invokes one of the same-named member functions of the base class), it shows ambiguity.
The online C++ compiler gets confused in selecting the member function of a class for the execution of a program and gives a compilation error.
Program to demonstrate the Ambiguity Problem in Multiple Inheritance in C++
Output
Ambiguity Resolution in Multiple Inheritance
This problem can be solved using the scope resolution (::) operator. Using the scope resolution operator, we can specify the base class from which the function is called. We need to use the scope resolution (::) operator in the main() body of the code after we declare the object and while calling the member function by the object.
Syntax:
Ambiguity Resolved Code
Output
How Does Multiple Inheritance Differ from Multilevel Inheritance?
In multiple inheritance, the derived class inherits the properties from more than one base class.
But, in multilevel inheritance, the properties are derived to one class from another class, and then again, the class that was derived class for the previous level acts as the base class for another level.
Syntax of Multilevel Inheritance:
Syntax of Multiple Inheritance:
The Diamond Problem
A diamond pattern is created when the child class inherits from the two base/parent class, and these parent classes have inherited from one common grandparent class(superclass).
As shown in the figure, ClassC inherits the traits of SuperClass twice—once from ClassA and again from ClassB. This creates ambiguity since the compiler fails to understand which way to go.
Code example of the diamond problem:
Output
Here, you can see that the superclass is called two times because of the diamond problem.
Solution of the Diamond Problem: The solution is to use the keyword virtual on the two parent classes, ClassA and ClassB. Two-parent classes with a common base class will now inherit the base class virtually and avoid the occurrence of copies of the base class in the child class (ClassC here). This is called virtual inheritance. The virtual inheritance limits the passing of more than a single instance from the base class to the child class.
Fixed Code for the Diamond Problem
Output The result shows that ambiguity is removed using the virtual keyword:
Here in the output, we can see that the superclass's members are only inherited and have not formed copies.
In the image below, you can understand how the virtual keyword helps avoid duplicates in the base class when there is a diamond problem.
virtual keyword causes to call the only default constructor of the base class.
How to Call the Parameterized Constructor of the Base Class?
To call the parameterized constructor of the base class, we need to explicitly specify the base class’s parameterized constructor in the derived class when the derived class’s parameterized constructor is called.
The parameterized constructor of the base/parent class should only be called in the parameterized constructor of the subclass. It cannot be called in the default constructor of the subclass.
Code Syntax:
Visibility Modes in Multiple Inheritance in C++
The visibility mode specifies the control/ accessibility over the inherited members of the base class within the derived classes. In C++, there are three visibility modes- public, protected, and private. The default visibility mode is private.
- Private Visibility Mode: In Inheritance with private visibility mode, the public members(data members and member functions) and protected members of the parent/base classes become ‘private members' in the derived class.
The private members of the base class are not inherited. These members(of the base class) cannot be accessed in the derived class. And also, these members cannot be further inherited as the private members are not inherited.
Code:
Output
- Protected Visibility Mode: In inheritance with protected visibility mode, the public members(data members and member functions) and the protected members of the base/parent class become protected members of the derived class. As the private members are not inherited, they are not accessible directly by the object of the derived class. The difference between the private and the protected visibility mode is-
In private visibility mode, members cannot be accessed (or viewed) from outside the class as it cannot be inherited. Still, in protected visibility modes, members can be accessed outside the inherited class.
Code:
Output
- Public Visibility Mode: In inheritance with public visibility mode, the public members(data members and member functions) of the base class(es) are inherited as the public members in the derived class, and the protected members(data members and member functions) of the base class are inherited as protected members in the derived class.
In the derived class, the inherited members can be accessed directly using this visibility mode. The private members of the base class are still not directly accessible to the derived class as they are not inherited.
Code:
Output
Visibility Mode Table:
Advantages of Multiple Inheritance in C++
Multiple Inheritance is the inheritance process where a class can be derived from more than one parent class. The advantage of multiple inheritances is that they allow derived classes to inherit more properties and characteristics since they have multiple base/parent classes.
Example of Multiple Inheritance in C++
In the example of Multiple Inheritance, we will calculate the average of the two subjects.
The two subjects will have two different classes, and then we will make one class of Result which will be the derived class from the above two classes through multiple inheritance.
Now, we can access the member functions and data members directly when we inherit in public mode. We will use the data of both the parent classes, and then we will calculate the average marks.
Output
Conclusion
- The capability of a class to derive properties and characteristics from another class is called Inheritance.
- Base Class - A class whose members are inherited.
- Derived Class - A class that inherits the base class members.
- In Multiple Inheritance in C++, we can inherit data members and member functions from multiple(more than one) base/parent class(es).
- When the base classes have the same-named member functions and when we call them via derived class object. It shows ambiguity.
- To solve the ambiguity problem, we should use the scope resolution operator (::).
- A diamond pattern is created when the child class inherits values and functions from the two base/parent classes, and these parent classes have inherited their values from one common grandparent class(superclass), which leads to duplication of functions.
- To solve the diamond problem, we should use the virtual keyword, which restricts the duplicate inheritance of the same function.
- There are three visibility modes in C++ for inheritance - public, protected, and private. The default visibility mode is private.