Pair in C++
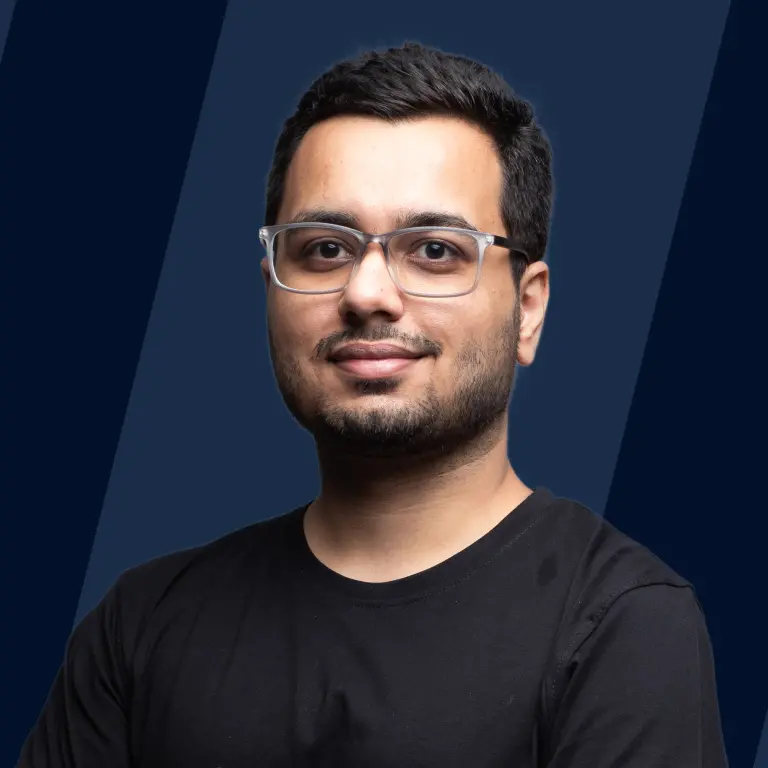
Overview
A pair in C++ is described as a container that combines two elements of the same or different data types in C++. Pair in C++ consists of two elements, first and second (must be in this order), and they are accessed using the dot (.) operator and the keyword first or second.
What Is A Pair In C++?
Pair in C++ behaves similarly to tuple in python. It consists of two elements {first, second}. The first element is referred to as first while the second element is referred to as second. This order must be fixed (first, second). The header file for Pair in C++ is <utility>, and it is also a container class in STL (standard template library).
Basic Syntax
The syntax to declare pair in C++ is as follows.
Parameters:
- data_type1 : data type of first element.
- data_type2 : data type of second element.
C++ Pair STL Functions
Function | Description | Syntax |
---|---|---|
make_pair() | It makes it possible to create a value pair without explicitly writing the data types. | pair1 = make_pair(data1, data2); |
swap() | It swaps the contents of one pair object with the contents of another pair object. The pairs have to be of the same kind. | pair1.swap(pair2) |
tie() | It unpacks the pair values into independent variables by creating a pair of lvalue references to its arguments. | tie(int &, int &) = pair1; |
Note: Actually, tie() is not a pair STL function. This is for tuples but can be used with pairs as well. Also, it requires a tuple header file to be used.
Example Explaining Pair STL functions
Output:
We can observe that we can access the pair elements using the (.) operator, and we have also seen how the swap and tie function works in pairs.
Nested Pairs
We can use nested pairs, also. The syntax to declare nested pairs in C++ is as follows :
Parameters:
Here we have a nested pair, i.e., the first or second element of a pair is itself a pair.
- dt1: data type of first element.
- dt2: data type of second element.
- dt3: data type of third element.
Let's understand nested pairs with an example :
Output:
We can observe that we can create nested pairs that can be accessed using first and second with the (.) operator.
Conclusion
- A pair in C++ is described as a container that combines two elements of the same or different data types.
- The header file for pair in C++ is <utility>.
- There are various pair STL functions, such as make_pair(), tie(), swap().
- We can use nested pair, i.e., the first or second element of a pair can itself be a pair.