Procedural Programming and Object Oriented Programming in C++
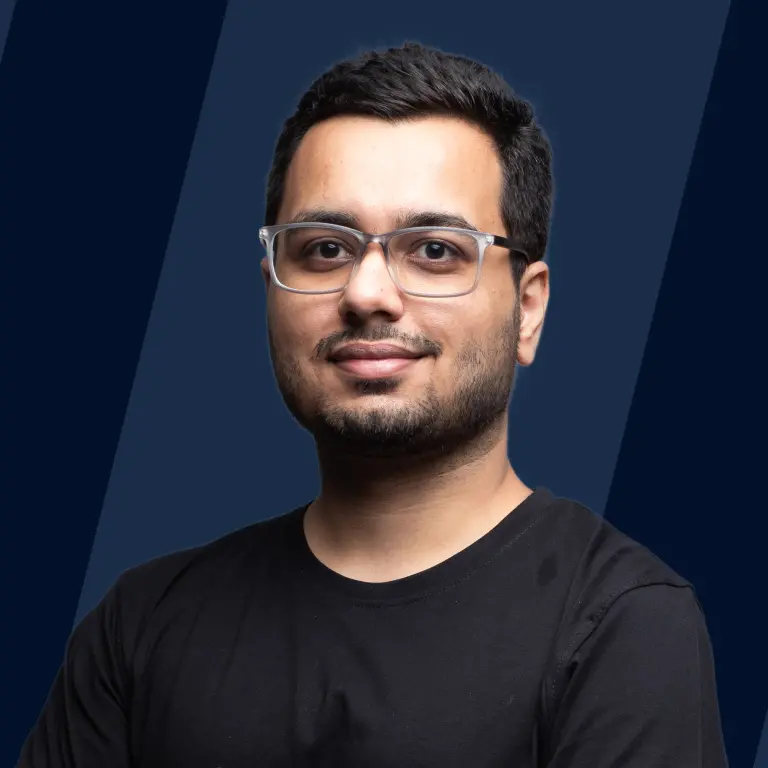
Overview
There are many differences between Procedural Programming and Object-oriented Programming. In procedural programming, we break down a task into variables and routines. After that, it is carried out every step individually, while in object-oriented programming, we try to see the whole world as an object.
What is Procedural Programming?
Procedural programming is a programming language derived from structure programming and based on the concept of calling procedure. The procedures are the functions, routines, or subroutines that consist series of steps to be carried out in the C++ OOPs concept.
The C++ OOPs concept breaks down a task into a set of variables and routines. After that, it carried out every step one by one. Procedural programming uses a linear top-down approach during the design of the program.
Key Features of Procedural Programming
OOPs concepts in C++ have some key features of procedural programming.
- Predefined Functions: The system libraries already define these functions. To build error-free code, a programmer will reuse the existing code in the system libraries. charAt() is an example of a pre-defined function that searches for a character position in a string.
- Local Variable: In programming, a variable is used only within the routine or function it is defined in. When the function is finished and control is returned to the part of the program that called it, the local variables no longer exist.
- Global Variable: A global variable is a variable that is declared in the global scope. In other words, a variable is declared outside every other function defined in the code. So, we can use global variables in all functions, unlike a local variable.
- Modularity: When two different systems have two distinct jobs at hand but are grouped to perform a bigger job first, this is referred to as modularity. Modularity is a powerful tool for reducing the complexity of systems.
- Parameter Passing: It’s a mechanism to pass parameters to procedures, functions, or routines. Parameter passing can be done through pass-by-value and pass-by-reference.
Languages Used in Procedural Programming
The languages used in Procedural Programming are FORTRAN, ALGOL, COBOL, BASIC, Pascal, and C. These languages use a list of instructions to tell the computer what to do step-by-step.
Advantages of Procedural Programming
- It is good for general-purpose programming.
- We can access the same code at different points in the software program without repeating it.
- The memory need is also reduced by using the Procedural Programming technique.
Disadvantages of Procedural Programming
- The code reusability feature is not present in procedural-oriented programming. We have to rewrite the same programming code many times.
- Difficult to relate to real-world objects.
- We can not perform operations like encapsulation, inheritance, etc.
What Is Object-Oriented Programming (OOP)?
As the name suggests, objects in programming. A programming model is based upon the concepts of objects, or where everything is represented as an object. In OOP, we try to see the whole world as an object. For example, dogs have states or data members like color, hungry, and breed, and the behaviors or methods (actions they can perform) are barking, wagging their tail, eating, sleeping, etc.
Basic Object-Oriented Programming (OOPS) Concept in C++ Class
Object-oriented programming aims to implement real-world entities in programming, such as inheritance, abstraction, polymorphism, and so on. Some C++ OOPs concepts that act as the basic building block of OOPs are:
- Object
- Class
- Encapsulation
- Abstraction
- Polymorphism
- Inheritance
- Dynamic Binding
- Message Passing
Let’s discuss them one by one.
Object:
OOPs concept in C++ has an object which is an entity with some characteristics and behavior or anything that exists physically in the world, like a dog, a pen, a pencil, etc. An object is an instance of the class. In Object-Oriented Programming, when a class is defined, no memory is allocated, but when an object is created, the memory is allocated.
Let’s see the sample code here:
Class:
In the C++ OOPs concept, a class is a blueprint of the object. When you define a class, you define a blueprint for an object. A class contains data members (variables) and member functions.
Consider the class of Person. There may be many people with different names and heights, but they all share some common properties. All of them will have a gender, age, occupation, etc. So here, the person is the class, and age, gender, and occupation are their properties.
In the C++ OOPs concept, data members are the data variables, and member functions are those used to manipulate these variables. Together, these data members and member functions define the properties and behavior of the objects in a class.
In the above example of a class Person, the data members would be age, gender, and occupation, and the member functions would be walking, eating, and sleeping.
Let’s see how we will define a class in C++.
OOPs concept in C++ has a class defined by the keyword class followed by the name of the class. The body of the class is defined inside the curly brackets and terminated by the semicolon at the end.
Output:
Encapsulation:
In the C++ OOPs concept, encapsulation means encapsulating (binding or wrapping) code and data into a single unit. More technically, encapsulation means placing the data and the functions that work on that data in a single place. Like, a capsule is wrapped with different medicines.
Let’s take a real-life example and try to understand it more clearly:
Assume you have an account in the bank. If you declare your balance variable as public in the bank software, your account balance will be public. In this scenario, anyone can know your account balance. So, do you want it? Clearly, no.
As a result, they define the balance variable as private to protect your account and ensure that no one can see your account balance.
The person who needs to check his account balance will only be able to access private members via methods defined inside that class, and this method will demand your account holder's name and password for authentication.
As a result, we can achieve security by using the principle of data hiding. This is known as encapsulation.
Abstraction:
In the C++ OOPs concept, abstraction is the most useful and necessary feature.
Abstraction means avoiding unnecessary and irrelevant information and only showing the specific details of what users want to see. Or in other words, abstraction refers to showing only the most relevant information while hiding the details. In C++, we use abstract classes and interfaces to achieve abstraction.
Let’s see some real-life examples to understand it more clearly:
Example:
Every time you log into your email account (Gmail, Yahoo, Hotmail, or official mail), many processes occur in the backend. You do not have any control over how the password is verified, which keeps it safe from misuse. Learn more about Abstraction in C++ here.
Polymorphism:
In the C++ OOPs concept, polymorphism means taking more than one form. Suppose you are in a classroom at that time. You behave like a student. When you are in the market at that time, you behave like a customer. When you are at your home at that time, you behave like a son or daughter. In this case, one person is exhibiting a variety of behaviors.
In the C++ OOPs concept, we use Operator overloading and Function overloading to achieve polymorphism.
- Operator Overloading: The process of making an operator exhibit different behaviors in different instances is known as Operator overloading.
- Function Overloading: When a single function name is used to execute many tasks, this is known as function overloading.
Suppose we have written a function that adds some integers. Sometimes there are two integers, and sometimes there are three. We can write the addition method with the same name but with different parameters, and we will call these methods according to the parameters. Learn more about Polymorphism in C++.
Inheritance:
When one class inherits the capabilities from another class or when one object acquires all the properties and behaviors of its parent object, then it is known as Inheritance. It helps to reduce the code size.
A real-life example of inheritance is a child and parents. All the properties of the father are inherited by his son.
Dynamic Binding:
In the C++ OOPs concept, binding is linking something to a thing, like the linking of objects. Dynamic binding, or late binding, is the mechanism used to select a particular function to run until the runtime. It is an alternative to early binding or static binding, where this process is performed at compile-time.
Dynamic binding is implemented with virtual function.
Message Passing:
Objects communicate with one another by sending and receiving information from each other. Message passing involves specifying the object's name, the function's name, and the information to be sent.
Web browsers and servers are examples of processes that communicate by message-passing.
Languages Used in Object-Oriented Programming
The languages used in object-oriented programming are Java, C++, C#, Python, PHP, JavaScript, Ruby, Perl, Objective-C, Dart, Swift, and Scala.
Advantages of Object-Oriented Programming (OOP)
- It helps in data hiding, keeping the data and information safe from leaking or exposure.
- Because OOPs provide reusability to the code, we can use a class many times.
- In OOPs, it is easy to maintain code as there are classes and objects, which help in making it easy to maintain rather than restructure.
Disadvantages of Object-Oriented Programming (OOP)
- As OOPs programs are large, they need more time to run, resulting in slower execution.
- OOP is not a universal language, so we can only use it in some places. It is only used when necessary. It is not appropriate for all sorts of issues.
- Everything is treated as an object in OOP, so before applying it, we need to think about objects well.
Procedural Programming vs. Object-Oriented Programming (Tabular structure)
On the basis of | Procedural Programming | Object-oriented programming |
---|---|---|
Definition | In procedural programming, we break down a task into variables and routines. After that, it is carried out every step one by one. | In Object-Oriented Programming, a programming model is based upon the concepts of objects, or where everything is represented as an object. |
Security | Procedural programming is less secure than Object-Oriented Programming. | Object-Oriented Programming is secure because data hiding is possible in OOPs due to abstraction. |
Approach | Procedural programming follow top-down approach | Object-Oriented Programming follow bottom-up approach |
Orientation | Procedural programming is structure/procedure-oriented | It is Object-oriented |
Access Modifiers | No access modifiers in Procedural programming. | Private, Public, and Protected are the access modifiers in Object-Oriented Programming. |
Code Reusability | No code reusability in Procedural programming. | Due to Inheritance, the concept of code reusability exists in Object-Oriented Programming. |
Virtual Class | In Procedural programming, there is no concept of virtual classes. | There exists a concept of virtual classes in Object-Oriented Programming. |
Overloading | In procedural programming, overloading is not possible. | Object-Oriented Programming has a concept of function overloading and operator overloading. |
Program Division | In Procedural programming, the program is divided into small functions. | In Object-Oriented Programming, a program is divided into small objects. |
Examples | Examples of procedural programming are FORTRAN, ALGOL, COBOL, BASIC, Pascal, and C. | Examples of object-oriented programming are Java, C++, C#, Python, PHP, JavaScript, Ruby, Perl, Objective-C, Dart, Swift, and Scala. |
Conclusion
- In procedural programming, we break down tasks into variables and routines. After that, it is carried out every step one by one.
- In object-oriented programming, we try to see the whole world as an object.
- Data members are the data variables and member functions are the functions used to manipulate these variables.
- The main difference between procedural and object-oriented programming is that procedural programming follows a top-down approach while Object-Oriented Programming follows a bottom-up approach.