‘this’ Pointer in C++
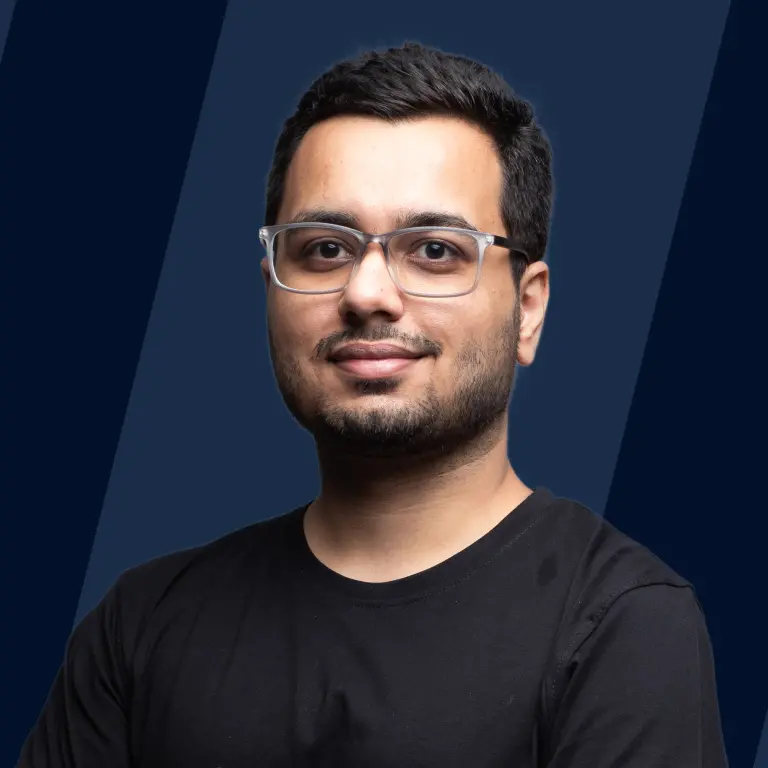
In C++ programming, "this" is a keyword representing the current class instance. It serves three primary purposes: passing the current object as a parameter to another method, referring to current class instance variables, and declaring indexers. The "this" pointer is automatically passed as a hidden argument in non-static member function calls.
Example
Suppose we create an object named objectA of class A. The class A has a non-static member function foo(). When we call the function using objectA as objectA.foo(), the pointer this is passed to the function by the compiler implicitly. this pointer can be accessed from inside the function body to access data members of objectA because it stores the address of objectA.
So, when we call the function foo() we are calling foo(&objectA) because C++ adds a new parameter to the function. Since a new parameter is passed to the function, C++ converts the function definition from
internally to
This new parameter is consistently named this and is a hidden pointer inside every class method (functions) that points to the class object.
Note: Friend functions don't have access to the this pointer because such functions are not class members.
Constness of this Pointer
It's important to note that this is a const pointer. We can change the value of the object it points to, but we cannot make it a point to any other object. This is why we can not declare and make assignments to the this pointer.
If a class has a member function declared with const, the type of this pointer for such class is of type const className* const. this pointer, in this case, can be used only with const member functions. Data members of the class will be constant within the function body, and to change their values inside the function; we will need to use const_cast as shown below:
Argument Matching
Whether class member functions are static, these functions are treated differently. Since non-static functions of a class have one extra implicit parameter (this), they are considered different from static functions, but they are declared identically. The non-static functions in class require an extra argument that should match the object type through which they are called. No temporary variable or conversion is attempted when trying to pass the this pointer argument in the function.
When an arrow operator is used on the class object to access its member function, then the this pointer argument has the type className * const. Similarly, when . is used to access object members compiler implicitly adds a prefix & (address-of) to the object name. For example,
compiler internally treats it as
Using this Pointer in the Constructor
We can use the this pointer inside the constructor body, and the reason this requires a special mention is that we think the object is not created entirely at the time of using the this pointer inside the constructor.
this pointer can reliably access the class data members inside the constructor body because by the time constructor is called, all the data members of a class are guaranteed to have been fully constructed.
Let us see an example of using the this pointer in the constructor.
Output
Here, we are using the this pointer in the constructor to initialize the data members of the respective object. The fact that the values of data members are initialized successfully (as seen from the Output) means the data members were declared for the objects pointA and pointB before the constructors started executing.
Deleting this Pointer
Delete is the operation used in C++ to de-allocate the storage space of the variable and can be used only on objects created using the new keyword. Generally, the delete operator should not be used with the this pointer to de-allocate it from memory.
Trying to delete the this pointer inside the member function is wrong and must be avoided, but if we try deleting the this pointer following things can happen.
- If the object is created on stack memory, then deleting the this pointer from the objects member function can either result in the program crashing or undefined behaviour.
- If the object is created in heap memory (using the new operator), then deleting objects from the this pointer will destroy the object from the program's memory. It will not crash the program, but later, if any object member function tries to access the this pointer, the program will crash.
Example
Output
In the above example, once we have called the function destroy on an object pointed by pointer ptr, it is safe to call the function displayText() because it is not accessing any data member inside the function body. But, if function bar() is called, the program will crash because we are trying to access values from a dangling pointer(pointer not pointing to a valid memory location).
Conclusion
- this Pointer in C++ stores the address of the class instance, which is called from the member function, to enable functions to access the correct object data members.
- Object reference is passed to a non-static function when a call is created implicitly by the compiler. The compiler automatically adds an extra argument to the class function definition before executing the program.
- This is a const pointer, and we can change the value of the object it points to, but we can not point it to any other object, and it can also be accessed in the object's constructor.
- Deleting this pointer can lead to the program crashing, especially if the object is created in stack memory, i.e., with the new keyword, and is generally not recommended.