What is Data Hiding in C++?
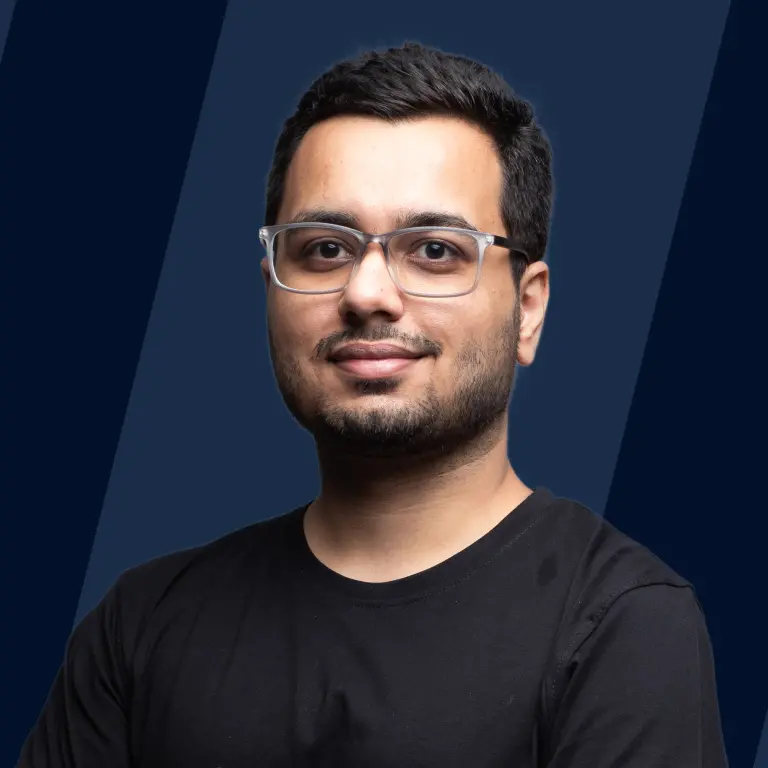
Data is the most confidential and sensitive information that must be kept safe and protected against unwarranted disclosure from all outsiders unless they have permission to access it.
Data hiding is an object-oriented programming technique for protecting the data within a class from unwanted access and preventing unneeded intrusion from outside the class. It helps hide internal object details, i.e., data members within the class, to prevent its direct access from outside the class. Data hiding is also known as Information hiding.
Data hiding guarantees the restricted access of data from unwanted sources and maintains data integrity by preventing unintended or intended changes. We will look into data hiding in this article's upcoming sections.
Introduction to Data Hiding
The term data hiding refers to the process of concealing or hiding data from program components that don't need to be retrieved. Data hiding helps in the protection of a class's members.
The data members and the member functions in a class are made private using data hiding so that they cannot be accessed falsely by functions outside the class and are protected from accidental modifications and changes. This helps in providing object integrity and in preventing unintended or intended changes.
Let's take one example to understand the concept of data hiding:
Suppose a class A has a variable var and two functions, one to input the number from the user and the other to display the variable entered by the user on the screen. Here, we will declare the variable var as private so that it can be accessed only by the same class members and can't be accessed anywhere else. Hence, we cannot access this variable outside the class, called data hiding.
The output of the above code is:
If we try to access the private variable var directly from outside the class, it will give an error.
The output of the above code will be a compile time error, as we can't access the private variable from outside the class.
Now, you would have understood the usage of data hiding and how to implement it in C++. Now, we will see two object-oriented programming concepts closely related to data hiding: abstraction and encapsulation.
We will look at each of these OOPs properties in the upcoming sections of this article.
Data Abstraction
Data Abstraction is a mechanism or technique of showing the users only the necessary interface details and hiding the complex and complicated implementation details from the user. It's the process of providing only the essential details to the outside world and hiding the complex internal details.
We can understand the concept of data abstraction with the help of an example: Let's suppose you want to sort a list of numbers in your C++ program, so for that, you can make a call to the sort() function without knowing what algorithm the function uses to sort the given values. Similarly, we use the pow() function to calculate the power of a number without knowing the algorithm the function follows.
This is done to hide the unnecessary internal details from the end-users and to make their task easier.
Data abstraction can be achieved by two types:
- Using classes.
- Using header files.
- Abstraction Using Classes: An abstraction can be achieved with the help of classes. As we know, a class is a group of all the data members and member functions that binds them all into a single unit. A class is responsible for determining which data member is to be visible and accessible outside the class and which is not with the help of the access specifiers.
Let's see a simple example to understand abstraction using classes:
The output of the above code is:
In the above example, abstraction is achieved using classes. Class Base contains the private members num1, num2, and subtract and are only accessible by the member functions of the class.
- Abstraction in Header Files: Abstraction can also be achieved using header files. As we have seen above, the pow() function is used to calculate a number's power without knowing which algorithm function uses to calculate the power. We can use the pow() function in our code after importing the necessary header files. Thus, header files hide all the implementation details from the user.
Let's take one simple example to understand abstraction in header files:
The output of the above code is:
As we can see from the above code, we can use the pow() function in our code after importing the necessary header file, i.e., math.h, even without knowing which algorithm the pow() function uses to calculate the power.
Data Encapsulation
Data encapsulation is one of the key features of object-oriented programming. It is the process of grouping or bundling the data members and member functions into a single unit known as a class.
Wrapping similar data members and functions inside a class help in data hiding. With this, you can hide sensitive information and limit access to the internal state only.
NOTE: Don't get confused between encapsulation and data hiding.
Encapsulation refers to bundling related fields and methods together, which can be used to achieve data hiding. Encapsulation is not itself data hiding.
The general form of encapsulation looks like this:
The code above shows that a class acts like a single unit consisting of member variables and member functions.
Let's take one example to understand the concept of data encapsulation:
The output of the above code is:
In the above example, as we can see, class A acts like a single unit consisting of member variables and member functions operating on those member variables. Here, the variable var is private. Hence, this variable can be accessed only by the same class members and is not accessible anywhere else. Hence outside the classes will be unable to access this variable which will help achieve data hiding.
Difference between Data Hiding and Data Encapsulation
Data Hiding | Data Encapsulation |
---|---|
The data hiding in c++ is an object-oriented programming technique that protects the data within a class from unwanted access and prevents unneeded intrusion from outside the class. | Data encapsulation is wrapping the data members and member functions into a single unit known as a class. |
Data hiding is the process or the technique itself. | Data encapsulation is the sub-process or method used to achieve data hiding. |
The data under data hiding is always under the private access specifier and is inaccessible. | The data under encapsulation may be private, public, or protected. |
Data Hiding focuses on restricting the access to data used in a program to assure data security. | Data Encapsulation focuses on enveloping or wrapping the complex data within a single unit to give a simplified perspective to the end user. |
Data hiding is data protection and ensuring security process. | Data Encapsulation is a method or a process of achieving data hiding. |
Access Specifiers
We have access specifiers to support the features of data hiding in C++, abstraction, and encapsulation in object-oriented programming. Access modifiers specify the access restriction to the class member functions. Access modifiers are also known as visibility modes in C++, and there are three types of visibility modes: public, private, and protected.
Access specifiers are mainly used for data hiding in c++ and encapsulation, as they can help us to control what part of a program can access the members of a class. So that misuse of data can be prevented.
-
Public Visibility Mode:
In public visibility mode, when we inherit a child class from the parent class, then the public, private, and protected members of the base class remain public, private, and protected members, respectively, in the derived class. The public visibility mode retains the accessibility of all the parent class members.
The public members of the parent class are accessible by the child's class and all other classes. The protected members of the base class are accessible only inside the derived class and its inherited classes. However, the private members are not accessible to the child class.
The syntax of using Visibility Modes is:
The visibility_mode can be public, private, or protected.
Let's see an example to understand the concept of public visibility mode:
The protected variable x2 will be inherited from the Parent class and will be accessible in the Child class. Similarly, the public variable x3 will be inherited from the Parent class. It will be accessible in the Child class, but the private variable x1 will not be accessible in the Child class.
-
Private Visibility Mode:
In the private visibility mode, when we inherit a child class from the parent class, all the base class members (public, private, and protected members) will become private in the derived class.
The access of these members outside the derived class is restricted, and the member functions of the derived class can only access them.
Let's see an example to understand the concept of private visibility mode:
As the visibility mode is private, so, all the members of the base class have become private in the derived class. The error is thrown when the object of the derived class tries to access these members outside the derived class.
-
Protected Visibility Mode:
In the protected visibility mode, when we inherit a child class from the parent class, all the base class members will become the derived class's protected members.
Because of this, these members are now only accessible by the derived class and its member functions. These members can also be inherited and accessible to the inherited subclasses of this derived class.
Let's see an example to understand the concept of protected visibility mode:
As the visibility mode is protected, the protected and the public members of the Parent class become the protected members of the Child class.
The protected variable x2 will be inherited from the Parent class and accessible in the Child class. Similarly, the public variable x3 will be inherited from the parent class as the protected member. It will be accessible in the Child class, but the private variable x1 will not be accessible in the Child class.
Given table will summarize the above three modes of inheritance in C++:
NOTE: If no visibility mode is specified, then, by default, the private mode is considered.
Applications of Data Hiding
-
It helps protect sensitive information against unwarranted disclosure from all outsiders unless they have permission to access it.
-
It helps in isolating the objects as the basic concept of object-oriented programming, which means that objects within the class are disconnected from irrelevant data.
-
It helps in increasing data security against hackers, which helps in ensuring that they are unable to access confidential data.
-
It helps to prevent damage to volatile and sensitive data by hiding it from the public.
Benefits of Data Hiding
Benefits of data hiding include:
-
Increased data protection and security against hackers.
-
It ensures data assurance from corruption and unwarranted access.
-
It is used to minimize data complexity.
-
It increases the program’s reusability.
-
It helps in defining the interface clearly and improves readability and comprehensibility.
-
It reduces system complexity and increases robustness by limiting interdependencies between software components.
Disadvantages of Data Hiding
-
The disadvantage of data hiding in c++ is that it requires additional coding to achieve the desired effect in hidden data. This makes the code lengthier. So, data hiding can be a harder process for a programmer, and he may need to write lengthy codes to create effects in the hidden data.
-
The link between the visible and invisible data makes the objects work faster, but data hiding prevents this linkage, making the process slow.
Conclusion
- Data hiding is an object-oriented programming technique for protecting the data within a class from unwanted access and preventing unneeded intrusion from outside the class.
- Data abstraction only provides essential details to the outside world and hides complex internal details.
- Data encapsulation is the process of wrapping or bundling the data members and member functions into a single unit as a capsule known as a class.
- Access specifiers provide access restrictions to the class member functions. There are three access specifiers: public, private, and protected.
- When the class members are declared public, they are accessible from anywhere.
- When the members in the class are declared private, then they are only accessible by the members of the base class.
- When the members in the class are declared protected, they are accessible in the base and derived classes.
- Private access specifier provided more security to its data members than the public and protected access specifier.
- Access modifiers are mainly used for data hiding in c++ and encapsulation as they can help us to control what part of a program can access the members of a class. So that misuse of data can be prevented.