Date Format in Java
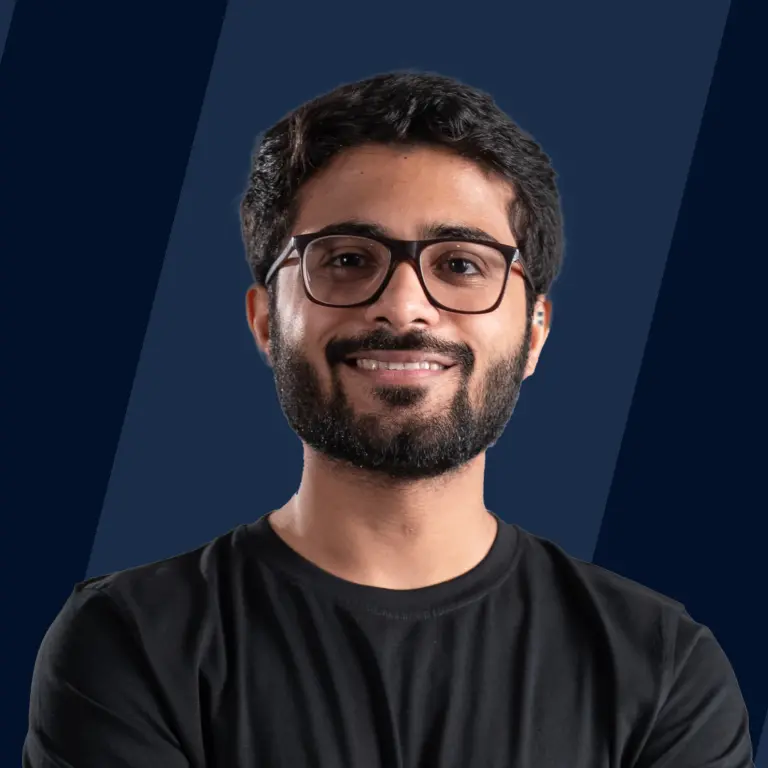
Discover the essentials of Java's DateFormat class in this article, a powerful method for managing date and time formatting within the Java programming environment. Whether it's coordinating transaction times or ensuring accurate scheduling, such as preventing double bookings in railway systems, mastering Java's date and time formatting is crucial. Let's delve into the DateFormat class and its practical applications in time-sensitive operations.
Create Simple Date Format
SimpleDateFormat is created using the SimpleDateFormat constructor, which is a parametrized constructor and requires a String pattern as a parameter.
According to the example above, the String pattern will be used to format dates, and the output will be shown as "yyyy-MM-dd".
Formatting Dates
Java's DateFormat class offers the format() method for formatting a given date to a Date/Time string. This method is used to convert this date and time into an appropriate format, i.e., mm/dd/yyyy.
Syntax:
A simple example of how to format dates using the format() method is shown below.
This formatted date string begins with the month, then the day, then the year. SimpleDateFormat determines the order of the date fields based on the pattern passed to it in the constructor.
Output:
Convert Date Into String
Date is converted to String using the format() method of the DateFormat class. The DateFormat class is abstract. SimpleDateFormat is a child class of DateFormat. It implements the DateFormat class. The approach we follow is listed below
- Simply define the SimpleDateFormat class to create the date format in the first step
- Call the format() method of SimpleDateFormat without going through the Date object; this method returns a String representation of the specified date
Syntax:
Let us look at an example
Output
Parsing Dates
Parsing is the process of converting Strings into Java.util.Date instances. By using the parse() method of the SimpleDateFormat class, we can parse a string into a date instance.
Strings can be converted to dates using the SimpleDateFormat class, which takes a string pattern as input and applies it to the class constructor. An example is shown below
Syntax:
Example
Output:
Creating a SimpleDateFormat For a Specific Locale
SimpleDateFormat instances can be created for specific Java Locales. If this is done, the dates will be formatted according to that locale. If you use a formatting pattern that includes the name of a weekday, the weekday will be written in the locales language.
Syntax:
Consider the case where we wish to use SimpleDateFormat in your specified locale . How would we do this? By following the code below we can do it
You can display the date in different countries as you wish by having the appropriate locales. You can see the different list of locales here
Output:
Pattern Syntax
Formatting dates and times is done by specifying pattern strings. The unquoted letters from 'A' to 'Z' and 'a' to 'z' within pattern strings representing date and time factors are interpreted as pattern letters.
If necessary, single quotes can be used to avoid ambiguity. As for all other characters, they will simply be copied into the output string during formatting or will be compared to the input string during parsing.
Let us look at the symbols we can use in date format
Symbol | Meaning | Presentation | Example |
---|---|---|---|
G | Era Designation | Text | AD |
Y | Year | Number | 2022 |
M | Month In A Year | Text & Number | March; Mar; 03 |
d | Day in a Month | Number | 5 |
h | hour in am/pm (1-12) | Number | 10 |
H | hour in day (0-23) | Text & Number | March; Mar; 03 |
G | Era Designation | Text | AD |
Y | Year | Number | 2022 |
M | Month In A Year | Number | 2 |
m | Minute in hour | Number | 40 |
s | Second in minute | Number | 50 |
S | Millisecond | Number | 968 |
E | Day in week | Text | Monday |
D | Day in year | Number | 180 |
F | Days of week in month | Number | 2 (2nd Mon in March) |
w | Week in year | Number | 25 |
W | Week in month | Number | 3 |
a | Am/Pm marker | Text | PM |
k | Hour in day (1-24) | Number | 20 |
K | Hour in am/pm (0-11) | Number | 2 |
z | Time zone | Text | India Standard Time |
Z | Time zone | RFC 822 time zone | -0800 |
X | Time zone | ISO 8601 time zone | -08; -0800; -08:00 |
The rest of the characters will be treated as normal text to be inserted into patterns and formatted dates.
See the JavaDoc for SimpleDateFormat to learn more about the patterns accepted
Java Date Time Format Example
We discussed different aspects of DateFormat and SimpleDateFormat. The following are some examples of time and date formats.
Symbol | Meaning |
---|---|
dd-MM-yy | 06-03-22 |
dd-MM-yyyy | 03-06-2022 |
MM-dd-yyyy | 03-06-2022 |
yyyy-MM-dd | 2022-03-06 |
yyyy-MM-dd HH:mm | 2022-03-06 22:00:00 |
yyyy-MM-dd HH:mm | 2022-03-06 22:00:00.888 |
yyyy-MM-dd HH:mm | 2022-03-06 22:00:00.888+0300 |
EEEEE MMMMM yyyy HH:mm | Saturday March 2022 10:00:00.760+0300 |
DateFormatSymbols
The DateFormatSymbols class contains localized date and time formatting information, like the days of the week and the months. DateFormatSymbols do not have to be instantiated directly. A DateFormat instance is automatically created when you call one of its factory methods. By calling SimpleDateFormat's getDateFormatSymbols() method, you can retrieve a DateFormatSymbols object.
Syntax:
Using the setWeekdays(String[] newWeekds) method of the DateFormatSymbols class in Java, we can set the weekdays of the calendar into some different strings. For eg., “Sunday” can be changed to "Pluviophile Wednesday”, “Monday” can be changed to "Petrichor Thursday” or into some other random strings.
Let us look at implementation of it
In the first step, the UK Locale instance of DateFormatSymbols is created. Second, a new set of names has been assigned to weekdays and then they are displayed with the original names to see the difference.
Output:
The DateFormatSymbols object allows you to set more date formatting symbols. The following methods can be used to set additional symbols:
See the JavaDoc for DateFormatSymbols to get more details about it
Set Time Zone of SimpleDateFormat
Java's Simpledateformat class can be used to display a date in multiple time zones. Quite often, Java applications are designed to display times in different time zones, for example, when a server runs on PST or GMT time and clients are global or at the very least located in global trading hubs such as Hong-Kong, Mumbai, Tokyo, London, etc.
Syntax:
A SimpleDateFormat's setTimeZone() method lets you set its time zone. Java's setTimeZone() method takes an instance of Java TimeZone as a parameter (java.util.TimeZone). Let us look at an example showing how to set its time zone.
Output
ISO Date Format
A numeric date format defined by the International Organization for Standardization (ISO) eliminates ambiguity in numeric calendar dates. North Americans, for instance, writing the month before the date. In Europe, the date comes before the month, such as "30.3.1998" for March 30, 1998. Different countries also use different separators between numbers. It might also be useful to know how to express a date that precedes "1/1/1" (how to express a date in "BC") ISO 8601 establishes a standard that comprises the following elements:
- It is easier to process a date that is made up of a general to specific order. For example, the year is first, then The month, and then the day
- Hyphens (-) are used as separators
- when numbers less than 10 are preceded by a leading zero
- The year prior to year 1 should be expressed as "0" and the year before that as "-1" (and so on)
Use the following date pattern to create a Java SimpleDateFormat object when creating a date in ISO 8601 format using a time zone other than UTC
Syntax:
Let us know look at how to display ISO date format in java
Output:
Conclusion
- Date/time classes in JDK 8 as well as related formatting and parsing classes are much more easy to use than their predecessors.
- Using the DateFormat function, one can format a date into a String, depending on the locale provided.
- Using the Format and Parse function we can format and parse the dates
- The locale specifies the language and region of the code, making it more relevant to the user.
- Since Date Format classes are not synchronized, it's recommended that each thread has its own instance.