Date Validation in JavaScript
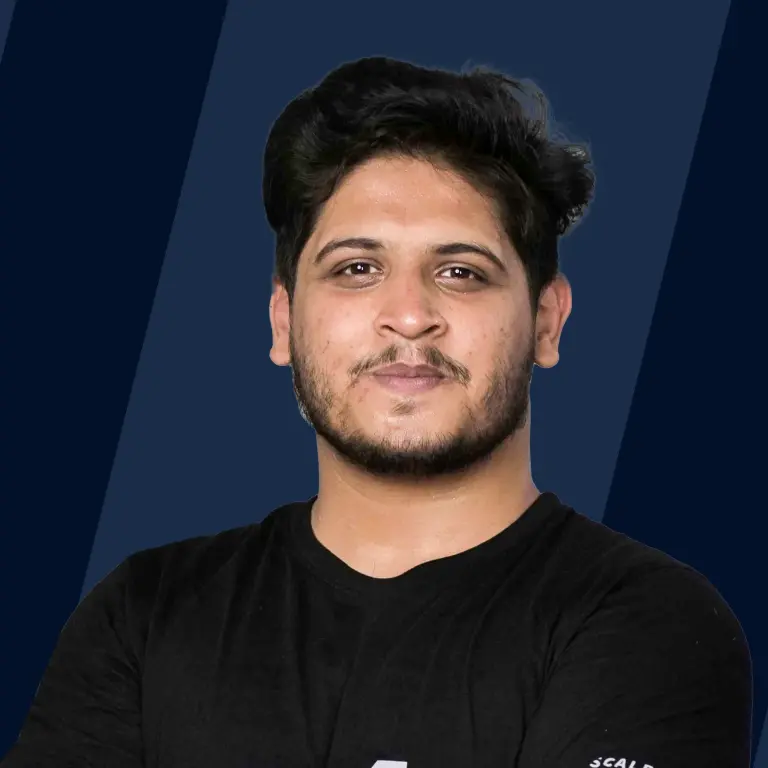
Date validation in JavaScript plays a crucial role as people around the globe follow various formats while entering dates. Date validation in JavaScript means checking whether the date entered by the user is valid or not. Whichever format the user has entered will be validated so that it is easy for developers to work with it. The various formats of dates are DD/MM/YYYY, MM/DD/YYYY, and YYYY/MM/DD.
Validate Dates in the Format: MM/DD/YYYY
First, we are going to check whether the date is valid or not. We are going to write a regex expression to match the date. If the date matches the format, we check whether it is valid or not. We will split the date into 3 parts by using the split() function.
If the month and date are out of range, i.e., if the day part exceeds the total number of days in that month or for a leap year if the month does not match the day, we return false.
In the end, if all the conditions are true, we return true that the format is correct.
Code-
**Explanation- **
First, the regex expression is matched and then checked. An array of date is created and the month part is extracted first, the 0th index will have the month, the 1st index will have the day and the 2nd index will have the year which is according to the given format. Now we will check if the month is out of range by checking if the date of the day part is greater than the total days in that month. The next validation will be to check whether the given year is a leap year or not. Otherwise, return false.
Now if we call the function,
The program will yield the following output-
If we use an invalid date,
The program will yield the following output-
Validate Dates in the Format of DD/MM/YYYY
The code is similar to the above where we have validated the MM/DD/YYYY format. For validation of DD/MM/YYYY, the change that we do is the regex expression and the sequence of year, month, and date.
Code-
Explanation-
First, the regex expression is matched and then checked.
An array of date is created and the day part is extracted first, the 0th index will have the day, the 1st index will have the month and the 2nd index will have the year which is according to the given format. Now we will check if the month is out of range by checking if the date of the day part is greater than the total days in that month. The next validation will be to check whether the given year is a leap year or not. Otherwise, return false.
Now if we call the function,
The program will yield the following output-
If we use a valid date,
The program will yield the following output-
Validate Dates in the Format of YYYY/MM/DD
The code is similar to the above where we have validated the MM/DD/YYYY and the DD/MM/YYYY format. For validation of YYYY/MM/DD, the change that we do is the regex expression and the sequence of year, month, and date.
Code-
Explanation-
First, the regex expression is matched and then checked. An array of date is created and the day part is extracted first, the 0th index will have the year, the 1st index will have the month and the 2nd index will have the day which is according to the given format. Now we will check if the month is out of range by checking if the date of the day part is greater than the total days in that month. The next validation will be to check whether the given year is a leap year or not. Otherwise, return false.
So, while we call the function,
Output-
Validate Date with the moment.js Library in JavaScript
There is a JavaScript library called moment.js which is used to validate the dates easily. In order to use this library, first, we need to download it. The moment() method present is used to check whether the date is valid or not.
The moment() function takes three parameters as input, the first parameter is the date that we want to validate the second one is the format i.e., DD/MM/YYYY, etc, and the last parameter is optional, if it is set as true then it will use the strict parsing. In strict parsing, the format and input should match correctly. At last, we use the isValid() function to check whether the input date is valid.
Example 1-
Output-
Example 2-
Output-
Validate Date with Date.parse() Method in JavaScript
The JavaScript Date.parse() method is used to check whether the given date is valid or invalid. The function returns NaN if the given date is invalid or else it returns the number of seconds elapsed since January 1, 1970. By this, we can check the validation of the date given.
Example-
Output-
Conclusion
- Date validation in JavaScript means checking whether the date entered by the user is valid or not. Whichever format in which the user has entered, it will be validated so that it is easy for us to work with it.
- The various formats of dates are DD/MM/YYYY, MM/DD/YYYY, and YYYY/MM/DD.
- Here we use regular expressions to separate the input date entered.
- There is a JavaScript library called moment.js which is used to validate the dates easily. The moment() method present is used to check whether the date is valid or not.
- The JavaScript Date.parse() method is used to check whether the given date is valid or invalid. The function returns NaN if the given date is invalid or else it returns the number of seconds elapsed since January 1, 1970.