How to Declare an Array in Java?
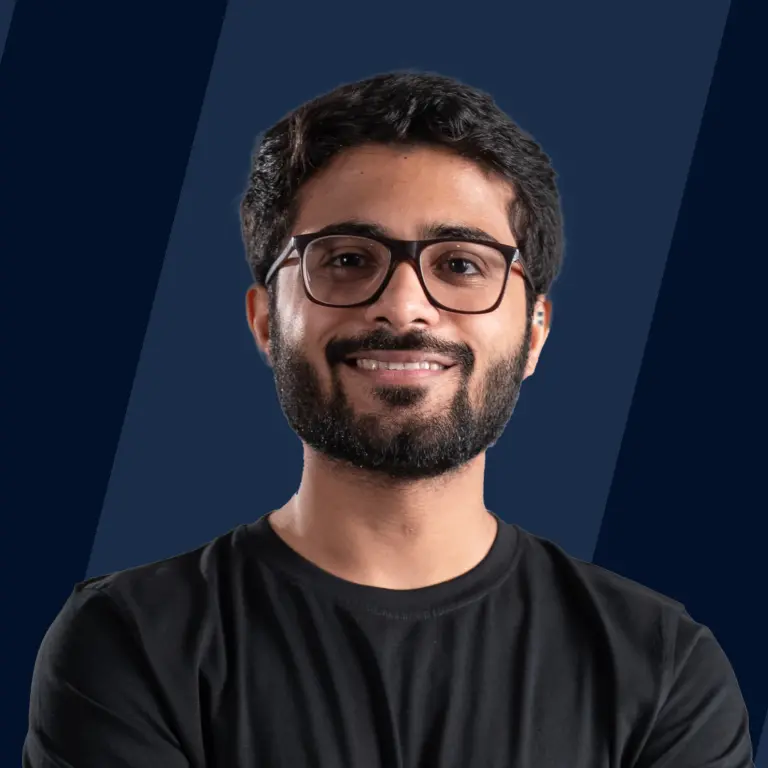
In Java, we can declare an array by specifying the type of the elements it will contain, followed by square brackets [], and then providing name of the array (there is variation too). You can also optionally specify the initial size of the array using a number in square brackets. We will discuss it all, the array declaration followed by their intialization and other relevant details in this article.
Introduction
The array is one of the essential concepts in java programming and it is used extensively to store similar types of data elements in contiguous memory locations.
As shown in the above representation an array contains similar types of data elements in the contiguous memory locations and each element is having some index number associated with it. The index of an array starts from 0 as you can see above.
The declaration of array in java is similar to the way we declare the variables in java. First of all, we need to know which type of data we want to store in the array, for example - int, float or string, etc.
First, specify the datatype and then write the name for an array element at the time of declaration.
Following are the two valid ways to declare an array -
In the above examples, we saw the declaration of array in java with type integer and the name of an array is myArray, [] denotes it as an array. In these examples, we only declared the array and they are not initialized with memory.
Array Initialization in Java
There are various ways to initialize and allocate the memory to an array, with the declaration of array in java.
We can use the new keyword to directly specify the size of the array as follows -
In the above example, the array with size 5 gets initialized with the default value of 0 at all locations.
Java provides the default values to an array if the values are not provided at the start depending on a datatype. 0 for integers, null for strings, false for booleans, etc.
We can initialize the elements in array by using the index position of an array. The index of an array starts from 0.
In this above example, we done the declaration of array in java having size of 5 consecutive memory locations and we initialized the elements at the 0th and 4th index numbers. But this way of initialization is a little slow because we are initializing values one by one.
To initialize multiple values at once we can use the {} as follows -
In the above example, we created an array of 4 elements and assigned the multiple values at once. Here the size of an array is 4 because we specified the 4 elements inside the {}.
We can use this same technique for strings and booleans as well.
In java we can initialize the array using new keyword as follows -
Note: Once the size of array is specified it would not change.
Can We Declare an Array Without Size?
Declaration of array in java without specifying its size is not possible. If we declared an array and never initialized it then it gives the NullPointerException.
Initializing And Accessing Array Elements
There are multiple ways for initializing and accessing the elements from an array as follows -
1. Using For Loop
We can use the for loop to iterate over an array by taking advantage of index numbers. We can loop through the array to initialize and access the values as demonstrated in the following code example
Output:
In the above code example we first did the declaration of array in java and then for loop is used to initialize and access the values to myArray, variable i is used to represent the index number in each iteration.
2. Using Arrays.fill()
Suppose we want to initialize the same value at each position of an array, then fill() method in java comes in very handy.
fill() method is present in the arrays class which is part of java.util package. This method is useful to initialize the same value at all indices of an array, as shown in the following code example
Output:
In the above example, we did the declaration of array in java with type String and initialized the value "empty" to all the positions of myArray using Arrays.fill() method.
3. Using Arrays.copyOf()
To copy all the values from one array to another array we use copyOf() method. This method comes from Arrays class which is the part of java.utils package. Following is an example of code to copy values from one array to another array.
Output:
Conclusion
- An array in java is used to store the data elements of similar type in contiguous memory locations.
- We can do a declaration of array in java in a similar way we declare the variables.
- It is possible to initialize an array with or without the new keyword. We can make use of {} to directly initialize multiple values.
- We can use the index numbers of the memory location to access the elements of an array.
- There are multiple methods provided in an arrays class which is a part of java.utils package to work with arrays.
- If we just declared the array and never initialized it then the compiler gives the NullPointerException.