What is Destructor in Python?
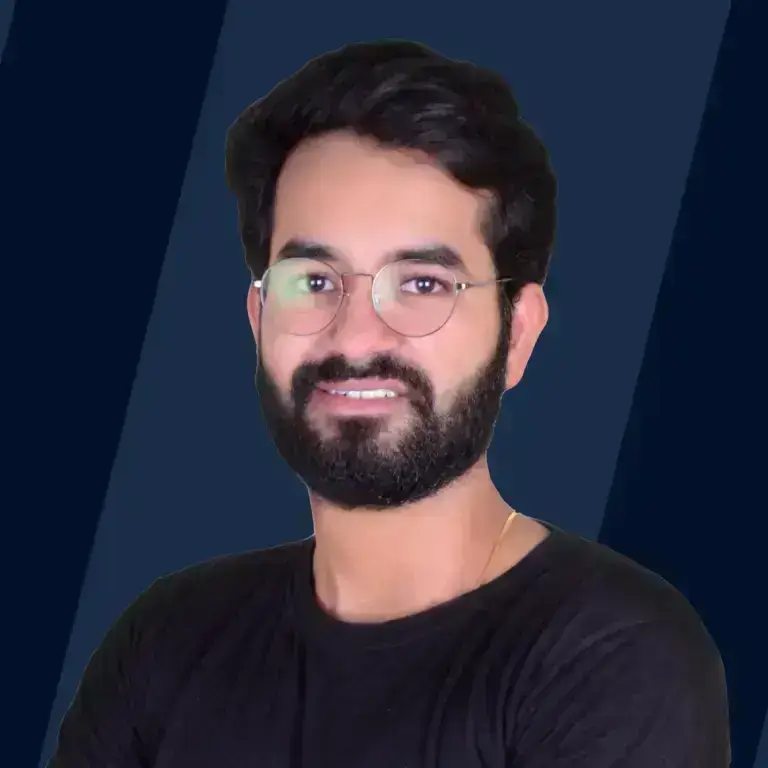
What is Destructor in Python?
When an object is erased or destroyed in object-oriented programming, a destructor is invoked. Before deleting an object, the destructor in python executes clean-up operations such as memory management. Destructor and constructor in python are quite diametric in nature. Constructor is automatically called when an object is created, whereas destructor is called when an object is destroyed.
Syntax of destructor in Python
Here,
- def is a keyword used to define a method in python.
- __del __() Method: In Python, the __del __() is referred to as a destructor method. When all references to an object have been erased, i.e., once an object's garbage is collected, this method is invoked.
- self: The self-argument reflects one of the given class's instances (objects).
Use of destructor in python
When a class object and its members are destroyed, destructors are typically used to deallocate memory and perform various cleaning operations. The destructor is called when a class object goes out of existence or is removed.
Although python contains a built-in garbage collector that takes care of memory management, when an object is destroyed, it is not simply memory that must be released. Other assets that the object was utilizing, sometimes including open files, database connections, and clearing up the buffer or cache, must be released or closed. In Python, we use a destructor to handle all of these cleaning chores. For instance, consider an E-scooter whose code destroys the "battery" object. But before destroying the battery, one would have to turn off the ignition and ensure that the scooter is resting on the stand. And the latter is the job of the destructor.
How to use the __del __() method
Example 1: Using Destructor
Destructor was automatically invoked because we used the del keyword to delete all references to the object.
Code:
Output:
Explanation:
Despite the fact that no methods were called, the output is shown. That is because in python the constructor and destructor are automatically called.
Example 2: Invoking destructor at the end of the program
The destructor is invoked when the program is finished or when all references to the object are erased, not when the object is removed from scope. This is demonstrated in the following example.
Code:
Output:
Example 3: Using destructor in circular reference
So in the next example, when fun() is invoked, it will create an instance of class Name, which will then pass to class Register, which will update the reference to the class name, resulting in a circular reference.
Output
Normally, Python's garbage collection would destroy this item if it was detected as cyclic, but in this case, the usage of a custom destructor identifies it as "uncollectable". Basically, it doesn't know how to destroy the items in the proper order. As a result, if your instances are engaged in circular references, they will remain in memory for the duration of the application's execution.
Important Points about Python Destructor
- When the reference count for any object drops to zero, the del function is invoked.
- When the program is closed, the reference count for that object drops to zero, unless we explicitly erase all references with the del keyword.
- When we delete an object reference, the destructor is not called. It will only run when all references to the objects have been removed.
- A scenario in which two objects refer to each other is known as circular referencing. When both of these objects lose their references, the destructor gets unsure which should be destroyed first, so it doesn't delete any of them to avoid an error.
Conclusion
- In object-oriented programming, when an object is erased or destroyed, a destructor is invoked.
- The destructor executes cleanup operations before deleting an object, such as memory management, open files, database connections, and releasing the buffer or cache.
- Before removing an object in Python, we utilize the del() function to tidy it up.
- When we delete an object reference, the destructor is not called. It will only run when all references to the objects have been removed.