Difference between Collection and Collections in Java with Examples
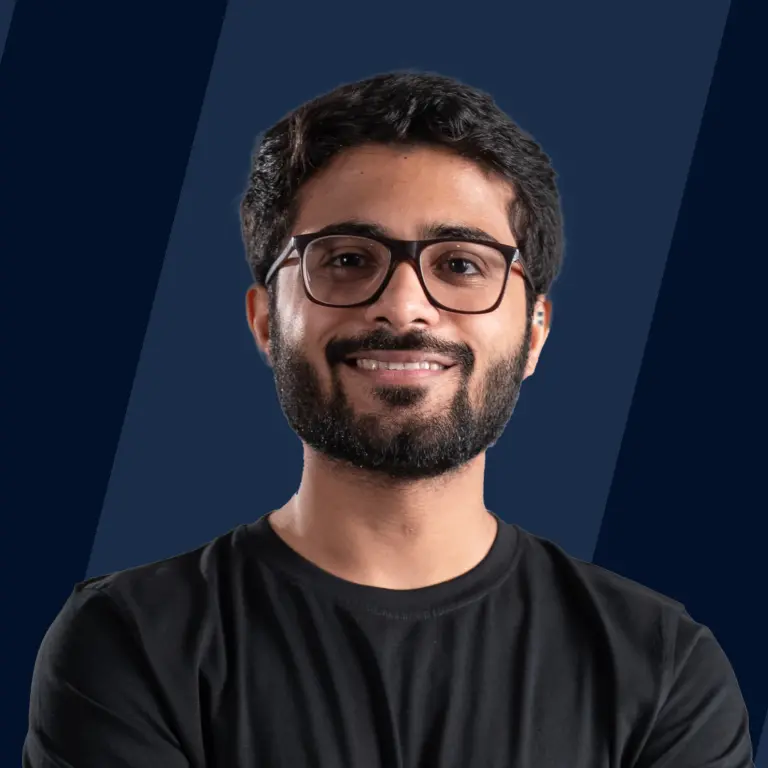
Overview
Java's Collections Framework provides a robust set of classes and interfaces for managing and manipulating groups of objects. However, newcomers to the framework might need help to ascertain the difference between Collection and Collections in Java due to their similar names but distinct roles. This article aims to clarify the difference between Collection and Collections in Java by providing a comprehensive overview along with illustrative examples.
Collection
The Collection interface serves as the foundation of the entire Java Collections Framework. It is an interface defined in the java.util package. It is synonymous with the container in the C++ language. It depicts a standard set of methods that all collection types must implement, such as lists, sets, and queues. This interface abstractly represents a group of elements and provides fundamental operations like adding, removing, and querying elements through functions like add(), remove(), clear(), size(), and contains(). Examples of classes that implement the Collection interface include ArrayList, HashSet, and LinkedList.
Declaration
Example: Using the Collection Interface
Code:
Output:
Explanation:
The code snippet above uses the Java Collection interface. It creates a Collection of strings (colours), adds elements, and showcases various collection operations. The program then prints the colours, displays the collection's size, and checks if the collection contains the colour Green.
Now let's discuss what are Iterators:
An iterator is an object that facilitates the traversal of elements within a collection (like a list, set, or other data structures) one element at a time. It provides methods to check for more elements to be traversed and retrieve the next element in the sequence. Iterators are a fundamental concept in programming, mainly when working with collections, as they enable efficient and consistent element access without exposing the underlying data structure.
Here's a simple example showcasing the use of an iterator to iterate through elements in a collection:
Output:
Explanation:
We create an ArrayList named numbers containing integer values from 1 to 5. We obtain an iterator for the numbers list using the iterator() method provided by the Collection interface. We use a while loop along with the hasNext() method of the iterator to check if more elements need to be traversed. Inside the loop, we retrieve the next element using the iterator's next() method and print it.
Collections
Contrary to the Collection interface, the Collections class is a utility class that provides various static methods to operate on or manipulate collections. It is defined in the java.util package. These methods include sorting, searching, reversing, and creating synchronized versions of collections. It acts as a helper class to perform everyday tasks on collections, making the development process more efficient.
The Collections class in Java's Collections Framework provides static methods that offer utility operations for working with collections. Since these methods are defined as static, they can be used without instantiating the Collections class. This design allows for a convenient and straightforward way to perform joint operations on collections without the need to manage instances of the utility class.
For instance, it has a method sort() to sort the elements in the collection according to the default sorting order, and it has a method max(), and min() to find the maximum and minimum value respectively in the collection of elements.
Declaration
Example: Using the Collections Class
Code:
Output:
Explanation:: This code demonstrates the use of the Java Collections Framework. It creates an ArrayList to hold integers, adds numbers to it, and then sorts the list using the Collections.sort() method. It prints the list before and after sorting. We then print the list using the Collections.reverse() method.
Difference between Collection and Collections
By now, you must have a good idea of collections and collections in Java. Now, let us look at the differences between them.
Collection | Collections |
---|---|
Collection in Java is an interface. | Collections in Java is a utility class. |
It represents a collection of distinct objects combined into a singular entity. | It defines various utility methods utilized for performing operations on collections. |
The Collection interface features a static method since Java 8. This interface can also house abstract and default methods. | It contains only static methods. |
FAQs
Q. What is the fundamental difference between Collection and Collections in Java?
A. The fundamental difference between Collection and Collections in Java is that Collection is an interface representing a group of objects as a unified entity. In contrast Collections is a utility class offering static methods to manipulate and operate on collections.
Q. Concerning role, what is the difference between Collection and Collections in Java?
A. The Collection interface defines the structure and methods for collections, while the Collections class provides utility methods for various collection-related tasks.
Q. Can you provide an example of Collection in action?
A. Certainly, an instance of Collection could be a list of student names, and methods like add(), remove(), and size() could be used to manage and query this collection.
Q. What functionalities can be achieved using the Collections class?
A. The Collections class offers methods like sort(), min(), and max() for sorting, finding minimum and maximum values, and other collection operations, enhancing development efficiency.
Conclusion
Here are the key takeaways from the article on the difference Between Collection and Collections:
- The collection interface serves as a blueprint for creating cohesive groups of objects, while the Collections class provides a toolbox of utility methods to work with these collections.
- Collection abstracts the concept of a group of elements, while Collections encapsulates utility methods to perform operations on these groups effectively.
- Collection is an interface forming the base of collection hierarchies, including sub-interfaces like List, Set, and Queue. On the other hand, Collections is a class containing utility methods.
- Collection doesn't contain static methods and mainly focuses on defining the structure of collections. In contrast, Collections exclusively comprise static utility methods like sort() and max().
- Collection enhances the understanding of how collections function, while Collections expedite development by offering pre-built methods to address common collection manipulation tasks.
- In essence, the Collection interface concentrates on the functionality and behaviour of collections, while the Collections class emphasizes operational methods to perform actions on these collections.