Difference Between For Loop and While Loop in Python
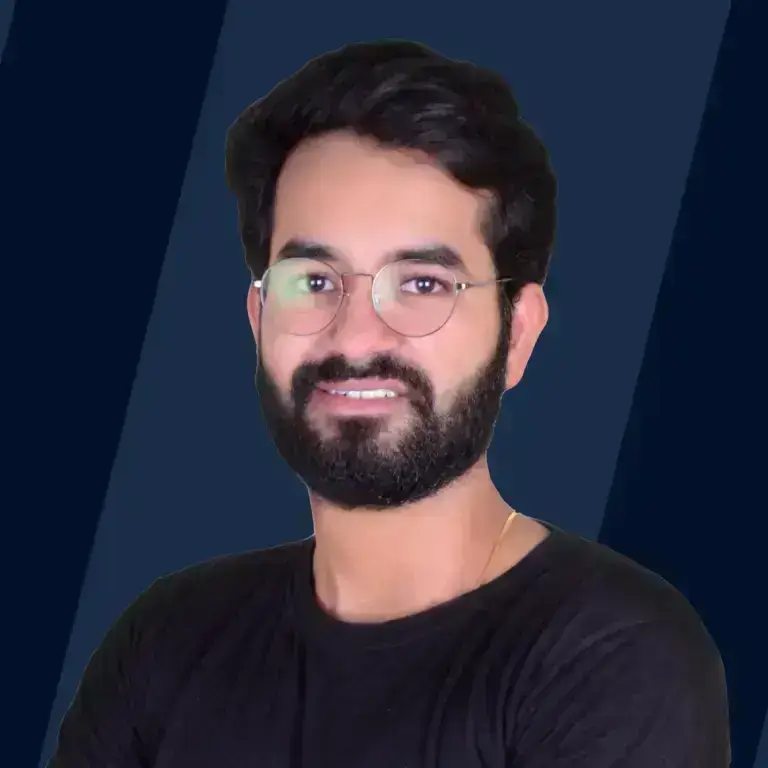
The loops are used to repeatedly execute the instructions till the condition is true. The difference between for loop and while loop is that for allows initialization, condition checking and iteration statements on the top of the loop, whereas while only allows initialization and condition checking at the top of the loop.
What are Loops?
Loops are the most powerful and basic concept in computer programming. A loop is an instruction that executes a statement until a specific condition is reached. The number of times the loop repeats itself is known as iteration. Some loop controlling statements are break and continue. Various types of loops are for, while, do while, etc. Every programming language, including C, C++, Java, Python, etc., has the concept of a loop.
For Loop in Python
A for loop is a control flow statement that executes code repeatedly for a particular number of iterations. In this control flow statement, the keyword used is for. The for loop is used when the number of iterations is already known.
The for loop has two parts:
- Header: The header part specifies the iteration of the loop. In this part, the loop variable is also declared, which tells the body which iteration is executed.
- Body: This part contains the statement executed per iteration.
Let's see the flow of the for loop:
- Initialise the starting value
- Check that the starting value is less than the stopping value.
- Execute the statement.
- Increment the starting value.
Let's see how the for loop works.
Syntax of for loop:
Example of for loop:
Output
While Loop in Python
A loop that executes a single statement or a group of statements for the given true condition. The keyword used to represent this loop is while. A while loop is used when the number of iterations is unknown. The statement repeats itself till the boolean value becomes false. In a while loop, the condition is tested at the start, also known as the pre-test loop.
Let's see the flow of the while loop:
- Initialise the starting value
- Check that the starting value is less than the stopping value.
- Execute the statement.
- Increment the starting value.
Let’s see how the while loop works.
Syntax of while loop:
Example of while loop:
Output:
Difference Between For Loop and While Loop in Python
The main difference between For and While Loops in Python lies in their iteration control. A For loop is used when the number of iterations is predetermined, often iterating over elements using functions like “range” or directly over generators. In contrast, a While loop operates without prior knowledge of iterations and allows for flexible initialization within its body. Performance-wise, For loops are generally faster than While loops.
Let's see the difference between the for loop and the while loop:
Parameter | For Loop | While Loop |
---|---|---|
Keyword | For Keyword is used. | While Keyword is used. |
Use | Number of iterations already known. | No prior information on the number of iterations. |
In absence of condition | Loop runs infinite times. | Display the compile time error. |
Initialization Nature | Once done cannot be repeated. | Repeat at every iteration. |
Initialization in accordance with iteration | To be done at starting of the loop. | Can be done anywhere in the loop body. |
Function used | Range or xrange function is used to iterate. | No such function is used in the while loop. |
Generator Support | For loop can be iterated on generators in Python. | While loop cannot be iterated on Generators directly. |
Efficiency | More efficient when iterating over sequences due to predetermined iterations. | May be efficient in situations where the condition can be evaluated quickly. |
Loop Nature | Used to iterate over a fixed sequence of items. | Used for more dynamic scenarios where conditions dictate loop continuation. |
Speed | For loop is faster than while loop. | While loop is slower as compared to for loop. |
So these were the main difference between the for loop and the while loop.
Initialization in Accordance to Iteration
In the case of the for loop, the syntax gets executed when the initialization is at the top of the syntax. On the other hand, in the case of the while loop, the position of the initialization statement does not matter for the syntax of the while loop to get executed.
When to Use?
The for loop is used when we already know the number of iterations, which means when we know how many times a statement has to be executed. That is why we have to specify the ending point in the for loop initialization.
When we need to end the loop on a condition other than the number of times, we use a while loop. In this case, it is not necessary to know the condition beforehand. That is why we can give a boolean expression in the initialization of the loop.
Absence of Condition
When no condition is given in the for and while loop, the for loop will iterate infinite times.. The difference between for loop and while loop in the absence of condition:
- For loop: The below loop will run infinite times.
- While loop: The below loop will run infinite times.
Initialization Nature
In the case of a for loop, the initialization is done once at the start, so there is no need to initialize it again. But in the case of a while loop, we require to initialize the loop manually by taking a variable that is further modified (incremented, decremented, multiplied, etc.) as per our requirement.
Learn More
Conclusion
Let's conclude our topic, "difference between for loop and while loop," by mentioning some of the important points.
- In a for loop, the set of declarations, including iterations and conditions, sits at the top of the body.
- In a while loop, initialization is always outside the loop. Before or after the execution of the statement(s), update can be performed.
- A for loop is a control flow statement that executes code repeatedly for a particular number of iterations.
- A loop that executes a single statement or a group of statements for the given true condition. The keyword used to represent this loop is while.
- When no condition is given in the for loop, the loop will iterate infinite times, while in the case of the while loop, it will give an error (compile time error).