Difference Between HashMap and HashTable
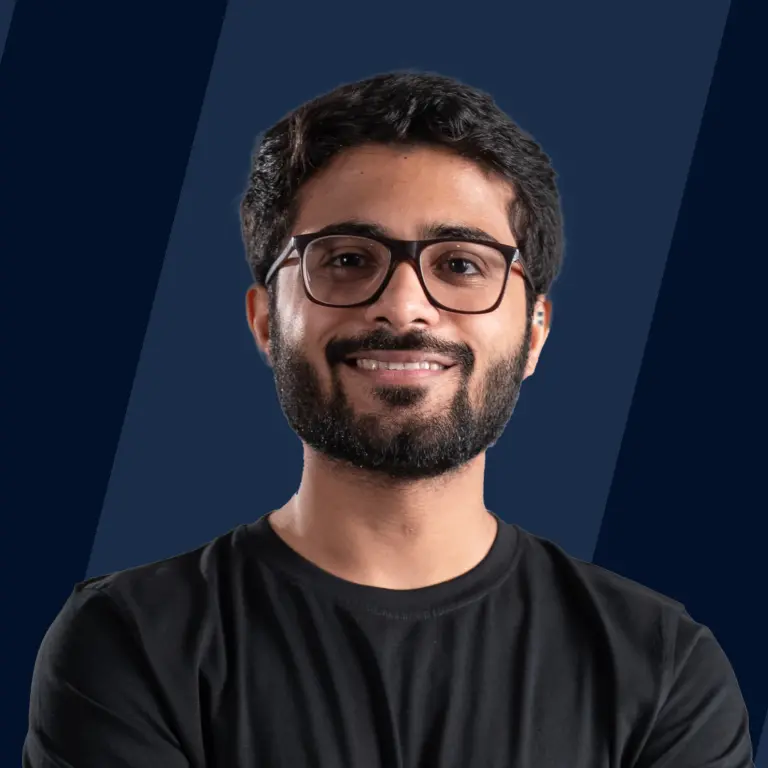
HashMap and HashTable are both key-value storage classes in Java. HashMap is non-synchronized, making it faster for single-threaded tasks, while HashTable is inherently synchronized, providing thread safety. HashTable doesn’t allow any null keys or values, but HashMap lets you have one null key and several null values. Additionally, HashMap can be molded to retain insertion order or sorted order using its various implementations, whereas HashTable doesn’t guarantee any particular order for its entries.
HashMap
A hashmap is a class that is a part of the Java collection framework since Java 1.2. This class belongs to java.util package. Java's Map interface can be implemented using this hashmap class. It usually stores the data in key, value pair (key, value), and you can access the value with the help of key in constant time, for example, an Integer. Inside a hashmap, one object is used as a key (index) to another object (value). If there is a key already existing in the hashmap and we again try to insert the same key, this time with a different value, then the value will be replaced with the new one of the corresponding key.
Syntax :
Example :
Output :
HashTable
A hashtable is a class that is a part of the Java collection framework. It implements a hash table, and it stores the data in key value pair (key, value). Inside a hashtable, we can store any non null object like a key or a value, that is, null values and keys are not allowed in hashtable. To store and retrieve objects successfully from a hashtable, the objects used as a key must implement the hashCode method and the equals method, that is the object which is used as a key is hashed, and the resulting hashCode which we get after hashing the object key is used as an index to get the value which is associated with the object key.
Although HashMap and HashTable are similar, there are certainly notable differences between them, let us discuss them.
Syntax :
Example :
Output :
Key Difference Between HashMap and HashTable
The main difference between HashMap and HashTable is their approach to synchronization and thread safety. While HashTable is inherently synchronized, ensuring that only one thread can access it at a time, HashMap is non-synchronized, allowing simultaneous access by multiple threads. This makes HashMap generally faster in single-threaded scenarios, due to the absence of synchronization overhead.
HashMap vs HashTable
Parameter | HashMap | HashTable |
---|---|---|
Synchronization | Non-synchronized. Multiple threads can access simultaneously, but synchronization must be externally handled for safe concurrent modification. | Synchronized. Only one thread can access at a time, ensuring thread safety. |
Null Values and Keys | Allows one null key and multiple null values. | Does not permit null keys or values. Inserting null can lead to a NullPointerException. |
Ordering | Can be used to implement LinkedHashMap (maintains insertion order) and TreeMap (sorted order). | Does not guarantee any specific order for its entries. |
Framework Membership | Part of the collection framework from its introduction. Implements the Map interface. | Initially not part of the collection framework. Later integrated after implementing the Map interface. |
Iterator Behavior | The iterator is fail-fast. Throws ConcurrentModificationException if modified by another thread during iteration. | Enumerator is not fail-fast. Due to internal synchronization, concurrent modification risks are minimized during enumeration. |
Thread Waiting and Performance | Multiple threads can operate without waiting. Generally offers better performance in single-threaded scenarios due to lack of synchronization overhead. | Threads may need to wait due to synchronization. This can lead to performance overhead in multi-threaded scenarios. |
Version Introduced | Introduced in version 1.2. | Introduced in version 1.0. |
Legacy Status | Non-legacy. | Considered legacy; newer implementations like HashMap are generally recommended. |
Why HashTable Doesn’t Allow null and HashMap Do?
In the above differences between HashTable and HashMap, there is a point : A HashMap can allow null keys and values, whereas a HashTable does not allow null.
You must be wondering, why a HashMap can store a null whereas HashTable cannot store a null. Let us understand the reason in detail.
A HashTable stores the object in the key, value pair. In order to store and retrieve the object successfully, the object which is used as a key must implement the hashCode method and the equals method. Since null is not an object, it cannot implement these hashCode method and the equals method. So if we store null inside the HashTable, it will not work and throw a null pointer exception error. However, HashMap is a modern version of HashTable and it was created later. It will allow one null key and any number of null values.
Let us now understand the above points with an example.
Code in Java :
Output :
Explanation :
In the above code, we are implementing both the HashMap and HashTable inside the public class MyClass. To demonstrate our above point, we have added null inside both the HashMap and HashTable to check whether it is working. We can clearly see, that the HashMap is working completely fine after storing the null as its key, and value, and it is also printing its data. However, the HashTable throws an error null pointer exception after we added null as data inside it. Hence, we can conclude that the HashMap allows null, whereas the HashTable does not allow null.
Let us now don't add null inside the HashTable and check if it is working or not.
Code in Java :
Output :
Explanation :
We can clearly see, that if we are not adding null inside the HashTable, it is not throwing any error, and the code is working completely fine.
Conclusion
- HashMap is a class within the Java collection framework introduced in Java 1.2. It implements the Map interface and stores data as key-value pairs.
- HashTable implements a hash table and similarly stores data as key-value pairs.
- For successful storage and retrieval in a HashTable, keys must implement both the hashCode and equals methods. The key’s hashCode, derived post-hashing, serves as an index to retrieve its associated value. Only non-null objects can be stored as keys or values.
- We highlighted some distinctions between HashMap and HashTable.
- HashMap is non-synchronized, while HashTable is synchronized.
- HashMap can accommodate one null key and multiple null values. In contrast, HashTable prohibits both null keys and values, leading to a NullPointerException upon attempting such insertions.
- Altering a HashMap during iteration can result in a ConcurrentModificationException. However, modifying a HashTable under the same conditions doesn’t raise any exceptions.
- HashTable disallows null keys and values because, to function correctly, keys must implement the hashCode and equals methods. Since null isn’t an object, it doesn’t implement these methods, and attempting to store null in a HashTable results in a NullPointerException.