Difference between Arrays and Pointers in C++
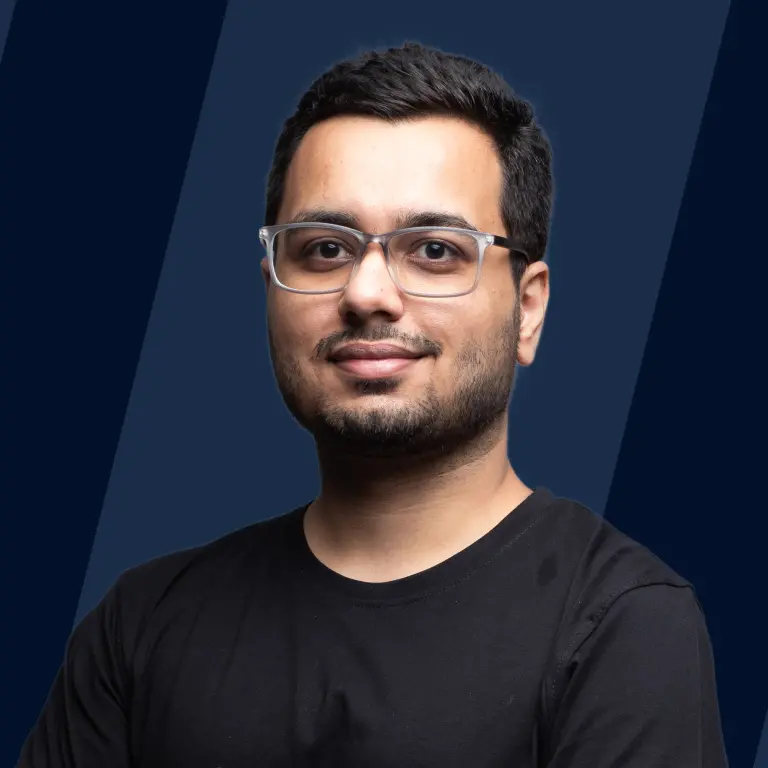
Overview
Arrays and pointers are essential data types in C++ frequently used to manage data collections. Pointers are variables that record memory addresses and enable flexibility but need careful memory management. Arrays are fixed-size containers that hold pieces of the same data type. Understanding these differences is essential for good programming.
Array
An array is a fundamental data structure in computer programming that allows you to store a group of items under a single variable name. Consider it a storage box with compartments, each containing a different value. These values can be of any data type, including integers, texts, and arrays. Arrays can be multidimensional as well. They are essentially arrays of arrays.
Syntax
Here's a simplified example in C++:
In this case, my_array is the variable name containing five integer values.
Example
Let's explore a practical example to illustrate the use of arrays. Consider a scenario where you need to store the daily temperatures for a week:
Example of Multi-dimensional Array
Now, you have a single variable, weekly_temperatures, with a collection of temperature values. You can easily access and manipulate these values using their positions within the array. For instance, to retrieve the temperature on the third day (68 degrees), you would use weekly_temperatures[2], as arrays typically start counting from 0.
Arrays are important in programming because they allow you to handle and modify data collections effectively, making them a vital idea to understand in computing.
Pointer
Pointers are important features in C++ programming that allow you to control memory locations and handle data effectively. They are essential when working with complicated data structures or optimizing code for performance. In this quick lecture, we'll go through the syntax of pointers in C++ and show how to use them with a simple example. Dynamic memory allocation in C/C++ allows programs to request and manage memory at runtime. new and delete are C++ operators that allocate and deallocate memory for objects, while malloc() and free() are C functions for generic memory allocation.
Syntax
Pointers are declared in C++ using an asterisk (*) symbol followed by the data type. For example, to define a reference to an integer, you might write:
This declaration creates a pointer named myPointer that can hold the memory address of an integer variable.
Example
Here's a straightforward example to illustrate the concept of pointers:
Explanation:
In this example, we define an integer variable myVar and a pointer ptr that contains myVar's address. We may indirectly access and alter the value contained in myVar by using *ptr.
Pointers in C++ are a powerful tool, but they must be used carefully to avoid memory-related difficulties such as segmentation errors. Understanding their grammar and use is critical for producing error-free code.
Difference between Array and Pointer in C++
Aspect | Array | Pointer |
---|---|---|
Definition | Arrays are collections of elements of the same data type stored in contiguous memory locations. | Pointers are variables that store memory addresses, allowing access to values indirectly. |
Declaration | Declared with a fixed size during compile-time. | Declared as variables that can be assigned dynamically during runtime. |
Size | Size is fixed and determined at declaration. | Size can vary, depending on what it points to. |
Initialization | Elements can be initialized individually or with initial values for the entire array. | Pointers can be initialized to point to specific memory locations or other variables. |
Memory Allocation | Memory is allocated statically or on the stack. | Memory allocation can be dynamic, using new or malloc, or static-like arrays. |
Accessing Elements | Elements are accessed using indices, e.g., myArray[3]. | Values are accessed indirectly by dereferencing the pointer, e.g., *myPointer. |
Address Manipulation | Address manipulation is not applicable. | Pointers allow for direct Manipulation of memory addresses. |
Reassignment | Array size cannot be changed once declared. | Pointers can be reassigned to point to different memory locations. |
Multidimensional | Multidimensional arrays can be created. | Pointers can point to arrays, making them flexible for multidimensional data structures. |
Nullability | Arrays cannot be set to null. | Pointers can be set to null, indicating they don't point to anything. |
Conclusion
- Arrays are static, fixed-size data structures, whereas pointers provide dynamic memory allocation and manipulation flexibility.
- Indexes are used to access arrays directly, whereas pointers allow indirect data access via memory locations.
- Pointers provide reassignment and nullability, making them more adaptable than arrays in some scenarios.
- Both arrays and pointers may be used to generate multidimensional data structures, but pointers provide more flexibility when working with complicated data arrangements.
- In C++ programming, knowing when to utilize arrays for simplicity and predictability and when to use pointers for flexibility and advanced memory management is critical.
- Pointers in C++ are a powerful tool, but they must be used carefully to avoid memory-related difficulties such as segmentation errors.