Difference Between Structure and Class in C++
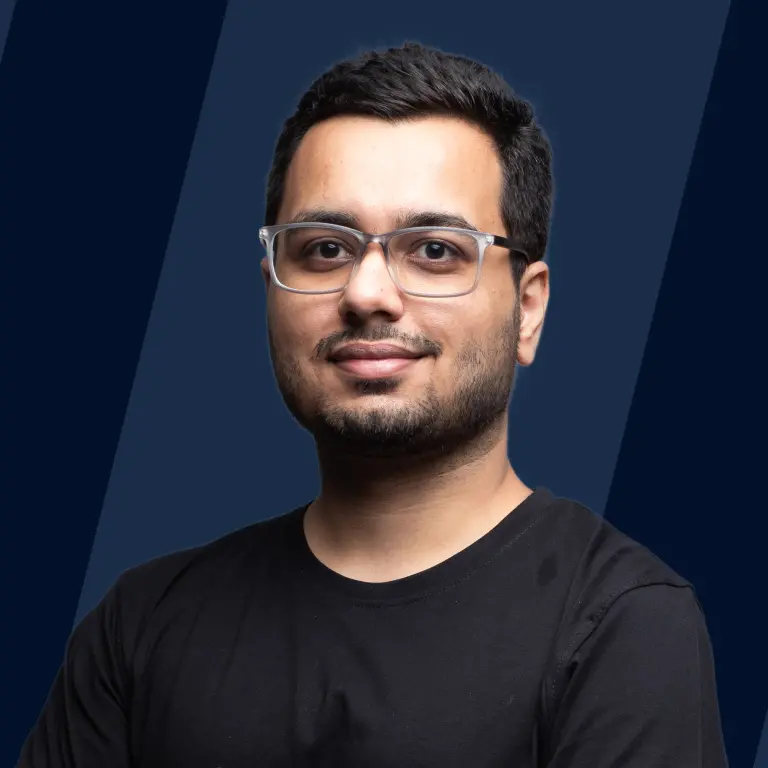
What is Structure in C++?
A structure in C++ is a user-defined data type that can be used to group items of possibly different types into a single type. The struct keyword is used to define a structure in C++. The items in the structure are called its members and they can be of any valid data type.
Here is the syntax for declaring a structure in C++:
For example, the following code declares a structure called Person with three members: name, age, and salary:
Once a structure has been declared, you can create a variable of that structure type. For example, the following code creates a variable of type Person and assigns it the values John Doe, 30, and 100000:
You can access the members of a structure using the dot notation. For example, the following code prints the name of the person:
What is Class in C++?
A class in C++ is a user-defined data type that can be used to group together data members and member functions. The class keyword is used to define a class in C++. The data members of a class are called its attributes, and the member functions are called its methods.
Here is the syntax for declaring a class in C++:
For example, the following code declares a class called Person with three attributes: name, age, and salary, and two methods: setName() and getSalary():
Once a class has been declared, you can create an object of that class type. For example, the following code creates an object of type Person and assigns it the values John Doe, 30, and 100000.
You can access the attributes and methods of a class object using the dot notation. For example, the following code prints the name of the person:
Key Difference Between Structure and Class in C++
- All structure members are by default public, whereas every class member is private.
- The members are automatically initialized by the structure, whereas constructors and destructors are used for initializing members of a class.
- The memory allocates on the stack when the structure is being implemented, whereas, for class, the memory gets allocated on the heap.
- In structure, the variables cannot be initialized during declaration, whereas in class, the variables can be initialized.
- No null values are allowed in structure members, whereas class values can have null values.
- A structure is usually a value type, whereas a class is usually of type reference.
Difference Between Structure and Class in C++
There are many differences between a structure and a class in C++. The features used to differentiate a structure and a class are their syntaxes, constructor, instance, data usage, memory allocation, etc. These basic features are used as a differentiating factor between the two. Here is the difference between structure and class in c++:
Parameters | Structure | Class |
---|---|---|
Basic definition | It is generally defined as the grouping of variables of various data types that are which is referenced by the same name. | A class is a collection of variables and functions which are related and contained within a single structure. |
Declaration | struct structure_name{ | class class_name{ |
Syntax | struct struct_name { | class class-name { |
Access specifier | All members are set to public if no access specifier is mentioned | All members are set to private if no access specifier is specified |
Inheritance | Inheritance is not supported | Inheritance is supported |
Instance | The instance of structure is allied as a ‘structure variable’. | The instance of the class is called an object. |
Allocation of memory | The allocation of memory is done on the stack. | The allocation of memory is done on the heap. |
Nature | Type value | Type reference |
Purpose | Grouping of data | Abstraction of data and inheritance is done further. |
Usage of data | It is mostly used for a small amount of data | It is used for a large amount of data |
constructor and destructor | It can only have a parameterized constructor | It can have all type of constructor and destructor |
Null values | Null values are not possible | It can have null values |
Examples Explaining the Difference Between Structure and Class
A class is generally declared using the class keyword, and the structure is declared using the struct keyword.
Example of class
Explanation:
In this example, the class car has private and public variables. The private variable includes the model number and name, whereas the public variable includes color and weight.
Example of structure
Explanation:
In this, the structure of the car is defined. Here, unlike in class, there are no private and public variables. Instead, all the variables are, by default, public.
Similarities Between Structure and Class in C++
Here are the following similarities between structure and a class:
- The members can be declared private in both class and the structure.
- The inheritance mechanism is supported by both class and structure.
- In C++, both the class and the structure are syntactically identical.
- The name of the class or structure can be used as a stand-alone type.
Extra Examples of Structures and Classes in C++ for More Understanding
Example:
Class members are private by default, whereas members of the structure are public.
C++ Program to Implement That Members of a Class are by Default Private
Output:
Explanation:
In this example, the class is made, and the variable a is initialized. Since the variable initialized in the class is private, it cannot be accessed outside the class. Hence when the variable is accessed by an object made in the main class, the compilation error is thrown.
C++ Program to Show That Members of a Structure are by Default Public.
Output:
Explanation:
In this example, the structure is declared, and the variable y is initialized. Since the variables initialized in the class are public, they can be accessed from outside the structure when called in the main function. Hence when an object in the main function accesses the variable y, the value is printed at the output.
Learn more:
Conclusion
- Structure is generally defined as the grouping of variables of various data types that are which is referenced by the same name.
- Syntax:
- A class is a collection of variables and functions that are related and contained within a single structure.
- Syntax: