What is Do While Loop in C++?
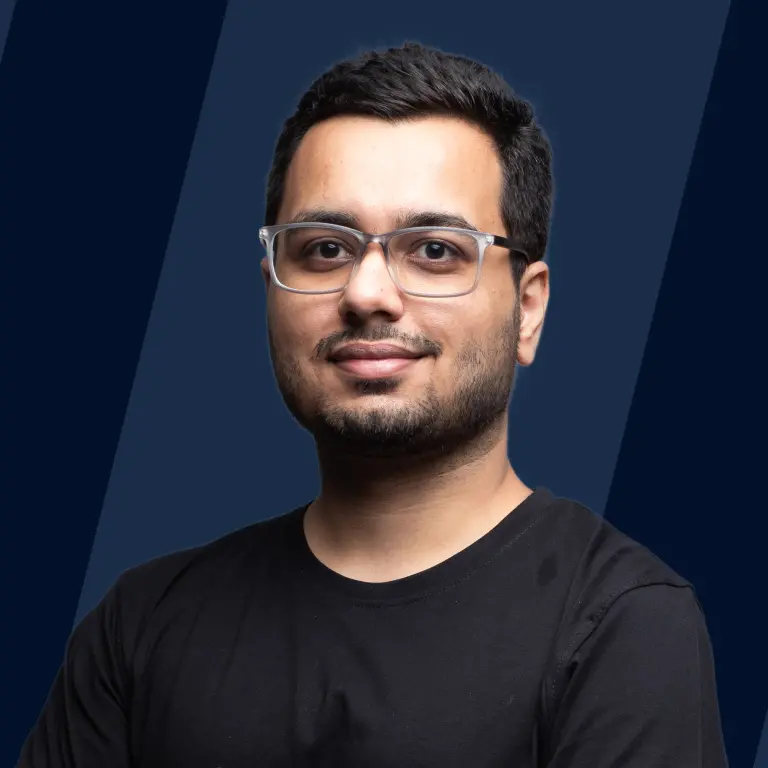
Before discussing the do-while loop, let's discuss control statements and loops in detail.
A control statement in C++ is a type of statement that controls the program's flow to execute a particular piece of code after checking a condition. In other words, a control statement redirects the flow of the program to execute a block of code. They are used for decision-making inside a program.
Every control statement depends upon a boolean value, true or false. If the condition returns true, the piece of code will be executed, and if the condition returns false, then the piece of code will not be executed.
Control statements are basically of two types:
- Conditional Statements
- Loop Statements
Conditional statements run a block of code if the condition inside the parenthesis returns true.
Conditional statements are of three types:
- if
- if else
- switch
Looping statements are used when we want to execute a piece of code for a specified number of repetitions or until a particular condition is fulfilled.
Loops are of three types:
- While Loop
- Do While Loop
- For Loop
Now, let's get back to the topic, i.e., do while loop in C++.
The Do while loop is an exit control statement used to iterate a block of code for a specified number of times according to the given condition. The condition is checked before the block of code gets executed in while and for loops, whereas in do-while loops, it first executes the block of code and then checks the condition at the end of the loop.
In simple words, while and for loop are controlled at the entry of the loop(also called entry-controlled loops), whereas the do-while loop is controlled at the end of the loop(also called an end-controlled loop). Hence, even if the condition returns false in the very beginning itself still, the loop will be executed once.
For example, if we want to check students' grades in a class, then instead of writing the same code for every student, we loop from 1 to the last student in the class.
Do while loop executes the block of code at least a single time without even checking the condition.
Syntax
The syntax for do-while loop in C++:
According to the syntax, the body will be executed first at least once without checking whether it is true or false.
How Does a Do While Loop Execute?
Following are the steps which will explain the working of do while loop and how they are executed in a program:
- Step 1: When the program's control reaches the do while loop, then unlike other loops, it does not check the condition.
- Step 2: The body of the loop gets executed first and updation(update_expression) takes place.
- Step 3: The loop's body will be executed at least once without even bothering about the given condition.
- Step 4: Then the condition(test_expression) is validated. If it returns true, the body of the loop will be executed again.
- Step 5: If the condition returns false, then the program flow exits the loop, and then the piece of code written outside the loop will be executed.
The above execution and working can be easily understood using a flowchart. Refer to the 'Flowchart' section of the article to understand more.
Flowchart
The above flowchart diagram thoroughly explains the execution cycle of the do-while loop.
Example
Let's take some examples to understand more about the working of do while loop inside a C++ program.
Example 1:
The following program will describe working the do while loop and printing a message for some specific number of times based on the condition.
Code:
Output:
Example Explanation:
The above program will print the message "Scaler Topics" till the test_expression or condition returns false. Every time the body of the loop gets executed, the expression gets updated, and the value of num increases by 1.
Example 2:
The following program is again a simple implementation of the do-while loop, but here, it is shown that even when the condition is not validated, the do-while loop will execute the body of the loop at least once.
Code:
Output:
Example Explanation:
The program prints the message only once, even after the condition given returns false. This is because inside a do while, the body of the loop executes once as the condition is validated after the execution.
Various Parts of the Do While Loop
There are two important parts of the do-while loop used in its execution as follows: a) Test Expression b) Update Expression
Test Expression:
The condition inside the program is known as the test expression. The test expression validates; if it yields true, the loop's body gets executed and updates the expression inside it. Else the flow of the program exits the loop.
According to the above examples, num < 1 and num < 5 are test expressions.
Update Expression:
If the loop's body gets executed, then the statement that updates the value in a program is known as an update expression. It increases or decreases the variable inside the body, so the test condition can be false at a certain value. If we don't have an update expression inside the body of the loop, then it will eventually lead to an infinite loop.
According to the above examples, num++ is an updated expression.
When Should You Use the Do While Loop?
A Do while loop is used when we want to execute the block of code inside the loop at least once, regardless of the condition. Whenever we want to repeat the execution of a block of code a specific number of times, we use a do-while loop. We mainly use a do while where we have to ensure that the code block must be executed at least once.
For example, When we do not initialize the value of a variable but rather take it as input from the user. So when we write the condition for the do-while loop, we cannot guarantee that the user's input is satisfying the condition, but still, we want to execute the body of the loop at least once and print the required statement in the output. In these kinds of cases, we have to use a do-while loop.
Let's see the implementation of the example above to understand it more clearly:
Code:
Output:
C++ Nested Do-While Loop
When a do-while loop is present inside another do-while loop, it is called a nested do-while loop. The inner do while loop is written as the body of the outer does while loop.
Now, let's see how the flow of nested do-while loop works, its syntax, and an example to understand it more clearly.
How Does a Nested Do While Loop in C++ Work?
Following is the working and flow of nested do while loop inside a program:
- Step 1: The program flow enters the first do-while loop, i.e., the outer loop.
- Step 2: When the execution of statements of outer loops is completed, the iterator enters the inner loop.
- Step 3: The body of the inner loops gets executed and then validates the test condition whether it returns true or false.
- Step 4: The inner loop execution continues until the test condition returns false.
- Step 5: After exiting the inner loop, the iterator validates the test condition of the outer loop.
- Step 6: If the test condition of the outer loop returns true, the iterator again goes back and starts the iteration from step 2.
- Step 7: If the test condition returns false, the flow of the program jumps out of the nested do while loop.
Syntax
Example
Let's take a simple example to implement a nested do-while loop in C++ where we will increment the value of variables and then print them with their incremented values.
Code:
Output:
Example Explanation:
In the above example, we have initialized two variables a and b. Now, the flow goes inside the do while loop, then the body of the loop gets executed, which prints the value of both a and b, and then the value of b gets incremented.
After updating the value of b, the condition is checked, and if it yields true, then the loop's body gets executed again until the condition returns false and the flow comes out of the inner loop.
Then the value of a gets incremented, and the condition of the outer loop is checked if it returns true. Then, the previous iteration process takes place until the condition returns false.
C++ Infinitive Do-While Loop
When a do-while loop executes an infinite number of times due to its test expression or condition, it is known as an infinitive do-while loop. Here the condition of the loop always returns true, which is why the body of the loop will be executed an infinite number of times. A do-while loop can be converted into an infinitive by using true as the test expression in a while ('while(true)').
Let's see the syntax used for infinitive do while loop in C++ with some basic examples.
Syntax
Example:
Let's see a simple implementation of infinitive do-while; a statement will be printed an infinite number of times.
Code:
Output:
We can use one more approach to implement an infinitive do while loop by not having an update expression which may increment/decrement expression in the body of the loop.
Code:
Output:
More Examples
Let's take some more examples to understand a bit more about do while loop in C++ and it's working:
Example 1:
The following example will print the total number of elements inside an array using the do-while loop in C++.
Code:
Output:
Example Explanation:
In the above program, we have initialized an array arr of size=6 and a variable i to access every element of the array initialized to 0. Inside the do while loop, we are printing the elements of the array, and every time after printing one element, the value i is increased by 1. After the increment, we check whether the test expression returns true. If it yields true, the loop's body is executed again.
Example 2:
The following program will add the entered number by the user until the user enters the number 0. After the user enters 0, the program will show the total sum of elements.
Code:
Output:
Example Explanation:
The above program will add the elements entered by the user. We have initialized two variables, i.e., num and totalSum of integer type. Inside the do while loop, we perform the sum of elements entered by the user and then check the condition that whether the number entered by the user is 0 or not. If the number is 0, the program flow exits from the loop and displays the total sum as seen in the output.
Difference Between While Loop Vs. Do While Loop in C++
Let's see some of the basic differences between the While loop and the Do while loop:
While Loop | Do While Loop |
---|---|
In a while loop, it first checks the condition or test expression, and then the loop's body will be executed. | In a do-while loop, it executes the body of the loop and then validates the condition or test expression. |
It is also called an entry-controlled loop. | It is also called an exit-controlled loop. |
If the condition returns false, then the body of the code will not be executed even a single time. | Even if the condition returns false, the loop's body will be executed at least once. |
The semicolon is not required when writing conditions in a while statement. | The semicolon is required when writing the condition in the while statement. |
Brackets(Curly braces) are not required if the loop's body contains a single statement. | Brackets(Curly braces) is required even if the loop's body contains a single statement. |
Learn More
- While Loop in C++:- While loop is a control statement used to control the flow of a program that allows a piece of code to be executed based on a particular condition.
Syntax of while loop:
Explore Scaler Topics C++ Tutorial and enhance your C++ skills with Reading Tracks and Challenges.
Conclusion
- Looping statements are used when we want to execute a piece of code for a specified number of repetitions or until a particular condition is fulfilled.
- Loops are of three types:
- While Loop
- Do While Loop
- For Loop
- Do while loop is a control statement used to iterate a block of code for a specified number of times according to the given condition.
- Do while loop executes the block of code at least a single time without even checking the condition.
- while and for loop are controlled at the entry of the loop( also called entry-controlled loops), whereas the do-while loop is controlled at the end of the loop(also called an end-controlled loop).
- When a do-while loop is present inside another do while loop, it is called a nested do-while loop.
- When a do-while loop executes an infinite number of times due to its test expression or condition, it is known as an infinitive do-while loop.
- We mainly use a do while where we have to ensure that the block of code must be executed at least once.