JavaScript DOM - Document Object Model
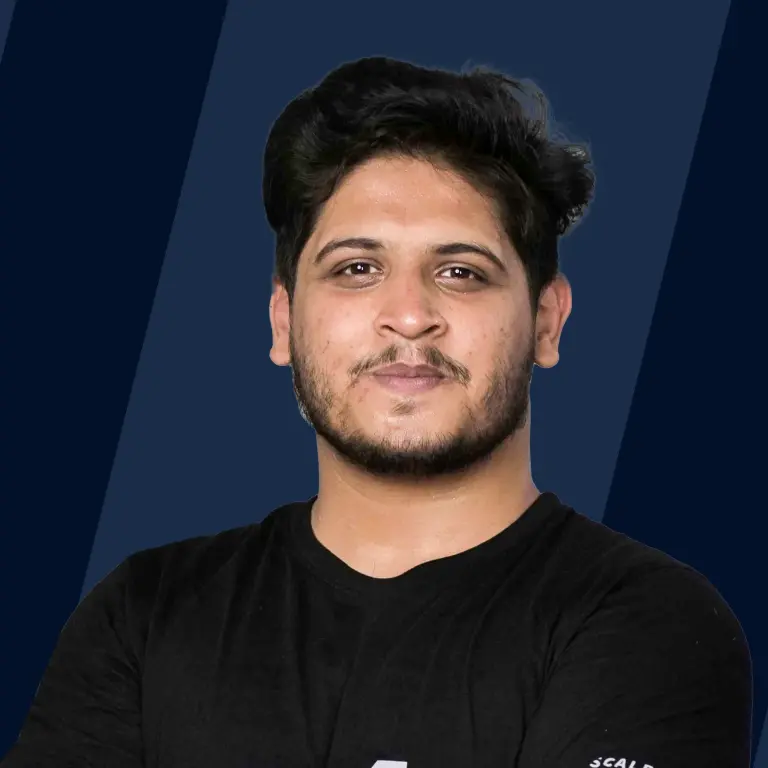
Overview
Each web page resides inside a browser window that can be considered an object, and the DOM lets you access and manipulates web page content using JavaScript. This guide will introduce the Document Object Model in Javascript and share detailed information about its hierarchy, properties, methods, DOM levels, and interfaces.
What is the Document Object Model in Javascript?
DOM stands for the Document Object Model in javascript. It works as a application programming interface for web documents (HTML and XML documents). Moreover, it is a "tree structure" representation built by the browser that allows the HTML structure to be easily accessed by programming languages such as javascript to change or manipulate the document structure, style, and content. It represents the document as nodes and objects in a tree structure.
Run the below code on your browser console and see the output.
The document object represent the whole HTML document. When the web page is loaded in the browser, it is converted into the document object. And the window object is the top element of the object hierarchy. So document and window.document both give the same result.
The output will show the document object with all the properties, methods, and events available for manipulating and creating web pages. For example, it helps to work with changing the content of HTML tags, dynamically styling the element or changing the color, creating or deleting a node, etc.
By manipulating the document object model in Javascript, you have endless possibilities. You can create applications that update the web page's data without needing a refresh. Also, you can create web pages that are customizable by the user and then change the page's layout without a refresh. You can drag, move, and delete elements.
Why DOM is Required?
HTML is used to create structured web pages, and javascript adds functionality and interaction to those web pages. However, when the HTML file is loaded into the browser, there is no way to modify it directly with javascript because javascript can not understand the HTML document. On the other hand, DOM is the same HTML document, but in the object form, javascript easily accesses the object, and we can easily dynamically manipulate the web page's content.
For example, JavaScript cannot understand HTML tags like p, h1 in HTML document, on the other hand DOM is the same HTML document but in object form, that's why JavaScript can easily understand objept p in DOM. It can easily access all those objects by using different properties and methods.
Structure of DOM
When the web document is loaded into the browser, it is converted into the document object, which will be much like a tree structure representation or, more precisely, like a "forest" or "grove." One important property of DOM structure models is a structural isomorphism.
Structural isomorphism:- If any two Document Object models in javascript implementations are used to represent the same document, they will create the same structure model with the same relationship and the objects. Let's look at this HTML code to understand the DOM tree structure better:-
Our document is called the root node, and it contains the one child node, which is the <html> element, and the element contains two more children, which are the <head> and <body> elements, even our element contains two more children element with the text content. Even a document can be viewed as a node tree or a collection of nodes, and nodes symbolize the branches and leaves of the document tree. There are many nodes, but the three main types are element nodes, text nodes, and attribute nodes.
Graphical Visualization of the HTML document:-
Key components of the document node tree structure are as follows:-
- Document node:- The whole web document is a document node.
- Element node:- Elements are the basic building blocks of the document, and they represent an element that exists in the DOM, such as , , are the element nodes.
- Text node:- The text node is contained by the element node, representing the text content of that element node such as title element contains "DOM" text content.
- Attribute node:- attribute node provides more information about the element node, such as a tag containing a href attribute with the external link.
And all the nodes of the DOM tree can be easily accessed and manipulated by javascript with the help of DOM properties. We will talk about this later in this article.
Why is it called an Object Model?
The name "document object model" was preferred because it is an "object model" in the traditional object-oriented design sense: documents are modeled using objects, and the model includes not only the structure of a document but also the behavior of a document and objects of which it is composed. In other words, the nodes in the above DOM structure do not represent a data structure; they represent objects which have functions and identities.
DOM and JavaScript
DOM was designed to be independent of any particular programming language and for making a structural representation of any web document. Even though we use javascript to access the document and manipulate its content, DOM is not part of the javascript language itself. Instead, it is a web API to build and manipulate the website. We can Easily access DOM with any programming language such as python because it's not only limited to javascript.
Properties of Document Object
Access to document object properties is essential to manipulate the content of web pages. Let's look at the properties of the document object that can be accessed and modified by the document object.
- Window Object:- The window object is the top-level object in the hierarchy. It includes property methods like document, location, etc.
- Document Object:- When the web page is loaded into the browser window, it becomes the document object. it is a tree-like structure that includes all the web page content. it includes,methods like getElementById , getElementByName to access the web content via the DOM.
- Link Object:- Link objects are objects or attributes set using the link tag.
- Anchor Object:- anchor objects are the objects that are represented by the href tag for reference of the links.
- Form object:- A Form object is enclosed between <form></form> tags that represent a form on a web page. Examples of form tags include a checkbox, radio, etc.
- Form Control Elements:- The form can have many elements to control the text input field, button, radio button, etc.
Methods of Document Object
We know that the document object provides many methods and properties to access and manipulate the document's contents. DOM methods are actions you can perform on ` objects (elements). The DOM methods include selecting an element, creating a new element, changing the element's content, deleting an element, etc. JavaScript is used to change or manipulate HTML elements, but before changing any element, we must select that element by some DOM methods.
Let's have a look at the essential methods of document object:-
Method | Description | Syntax |
---|---|---|
getElementById() | This method returns the first element with the specified ID. | document.getElementById(id) |
getElementsByClassName() | This method returns an object containing all the elements with the specified class names. | document.getElementsByClassName(name) |
getElementsByTagName() | This method returns all elements having the given tagname. | document.getElementsByTagName(tagname) |
querySelector() | This method returns the first element that matches a CSS selector. | document.querySelector("Name") |
querySelectorAll() | This method returns all elements in an array form that match the given CSS selector. | document.querySelectorAll("Name") |
createElement() | This method is used to create a new element. | document.createElement("tagName") |
Accessing the DOM
Accessing the various elements on a Webpage via DOM is a common way easily accomplished with JavaScript and the DOM API. You can use DOM API directly in javascript from within the script, a program run by the browser.
You can create a script, whether inline in an element or included in the web page. You can immediately use the API for the document or window objects to access or manipulate the document or any other elements in the web page. DOM programming is sometimes as simple just like the below console log example:-
We're printing a message "Welcome Back" to the browser's console every time the page loads. Still, it's generally not recommended to mix web page structure and DOM manipulation. But instead, the JavaScript part with HTML structure should be different.
Let's take a simple example for accessing the DOM:-
The simplest way to access a single element in the DOM is by its unique ID. We can grab an element by the given ID with the getElementById() method of the document object.
For example, we have a div HTML element with the "demo" ID.
Let's get the element and assign it to the demoID variable in the Console.
We have accessed the demo element and stored it into a variable. use the console method to print the variable:-
Fundamental Data Types
Various data types and objects are available in the document object model in javascript. The most commonly used data type in the DOM is "element", and most of the code within an HTML document is derived from "HTML node elements".
The vast majority of code that uses the DOMrevolves around manipulating HTML documents. It's common to refer to the nodes in the DOM as elements, although strictly speaking, not every node is an element.
The following table describes these data types in brief.
Data Types | Description |
---|---|
Document | The document is the root object of a DOM, and the document is used to represent which DOM element belongs to which document. The document refers to all web pages loaded in the web browser and includes sections such as body and tables in the DOM tree. |
Node | Every object located within a document is some type of node. In an HTML web document, an object can be an element node and a text node or attribute node. Accordingly, "document", "element", "attr", "characterdata", "document type" are examples of nodes. "Node.childNodes" and "Node.firstChild" are some of the methods one can use with the "node" data type. |
Element | The element data type is based on node. Element is the most commonly used data type in the DOM API. There are several types of elements in the DOM API. For example, HTMLElement, SVGElement. Each element in the DOM has specific methods and properties. The "document.createElement" allows the creation of an "HTML element" in a document. Various element methods such as "Element.classList" and "Element.attributes" manipulate the element. |
NodeList | A NodeList is a collection of multiple HTML elements of the same type. When HTML elements of the same type are selected with "document.querySelectorAll()", a "NodeList" is obtained. NodeList indexes start at 0 and items are accessed in two ways "list[1]" and list.item(1). |
Attr | Attribute properties in the DOM API can be called from the "createAttribute()" method. Properties are also nodes in the DOM API. "getAttribute", "setAttribute", and "removeAttribute" are other methods one can use within the DOM. For example, "attribute", "src", "alt", "width" and "height" are some of the attributes for img HTML tag. |
NamedNodeMap | The NamedNodeMap interface represents a collection of Attr objects.the objects inside a NamedNodeMap are in no particular order, unlike a NodeList, although an index can access them as an array. It has various methods like "NamedNodeMap.getNamedItems()", "NamedNodeMap.removeNamedItem()". |
There are also some general terminology considerations to keep in mind. It is common to refer to any Attr node as an attribute, for example, and an array of DOM nodes as a nodeList.
DOM Interfaces
This guide is about the document object model in javascript and the way you can use the DOM to manipulate web documents. There are multiple points where understanding how these works can be complicated. For example, the HTMLFormElement interface provides the Name property of an object representing an HTML form element, while the HTMLElement interface provides the ClassName property. In both cases, the property you are looking for is in the form object.
However, because the relationship between objects and the DOM interfaces they implement can be confusing, this section attempts to describe the actual interfaces of the DOM specification and how they are made available.
Interfaces and Objects
Many objects use interfaces from different sources. For example, the Table object implements the HTMLTableElement interface with methods such as createCaption and insertRow . Table, on the other hand, implements the Element interface as it is also an HTML element.
Ultimately, the Table object implements the more fundamental Node interface from which the element is derived. An HTML element is a node in a tree of nodes that composes the object model for an HTML or XML web page as far as the DOM is concerned.
Core Interfaces in the DOM
This section contains a list of some of the most frequently used DOM interfaces. The goal here is not to explain what these APIs do but to give you a concept of the methods and attributes you will often encounter when working with the DOM.
In DOM programming, the document and window objects are the objects whose interface you use the most. In simple words, the window object represents a browser, while the document object represents the document's root.
Elements inherit the generic Node interface, and these two interfaces together provide many of the methods and properties you can use on specific elements. These elements may also have unique interfaces for dealing with the type of data they maintain.
A Brief List of some of the Common APIs in Web and XML Page Scripting Using the DOM.
API | Description | |
---|---|---|
document.querySelector(selector) | The Document querySelector method returns the first element within the document that matches the specified CSS selector. | |
document.querySelectorAll(name) | The querySelectorAll method selects all the matching elements from the document and returns a static nodeList, an array-like object. | |
document.createElement(name) | It accepts an HTML tag name and returns a new Node with the Element type. | |
parentNode.appendChild(node) | The appendChild() method inserts a new node as the last child of a particular parent node. | |
window.scrollTo() | This method is used to scroll the document to specific coordinates. | |
element.innerHTML | This property sets or returns the HTML content of an element. | |
element.setAttribute() | The setAttribute() method sets an attribute for a particular element and assigns a value to it. | |
element.getAttribute() | This method is used to get the value of element attributes. | |
element.addEventListener() | This method attaches the event handler to an element. | |
element.style.left | The left property is used to set or return the left position of an element. | |
GlobalEventHandlers.onclick | The onclick event invokes Javascript code when a button, link, or tag is clicked. | |
GlobalEventHandlers.onload | The onload property of the GlobalEventHandlers mixin is an event handler that processes load events on a Window, |
Example
Let's take a simple example of the queryselectorAll method to select all matching elements of the given CSS selector.
This method is used to access one or more DOM elements that match the given CSS selectors:
The below code selects all the p elements from the document and returns the nodelist. We are using the console.log method to print the "matches" variable on the browser console.
Output:-
You can clearly see that in the above output there is a Nodelist in the array form with all available P elements, in our case they are two.
What DOM is Not?
The DOM works behind the browser, which creates a lot of confusion for people or false information about the DOM itself. Let's talk about some things that are not true, or DOM is not.
- DOM programs written in the same language will be source code compatible across platforms, but the DOM does not define any form of binary interface.
- The Document Object Model is not a way of describing objects in XML or HTML. Instead, it specifies how XML and HTML documents are presented as objects to be used in object-oriented programs.
- The Document Object Model is not a set of data structures, and it is an object model that specifies interfaces. Although this document includes diagrams showing parent/child relationships, these are logical relationships described by programming interfaces, not representations of any particular internal data structure.
- The Document Object Model does not define the "the true inner semantics" of XML or HTML. The W3C Recommendations define the semantics of these languages, and the DOM is a programming model designed to respect these semantics. The DOM does have no consequence for the way you write the XML and HTML documents. Any document written in these languages can be represented in the DOM.
- The Document Object Model, regardless of its name, is not a competitor to the Component Object Model (COM). COM, like CORBA, is a language-independent way to specify interfaces and objects.
Levels of DOM
The W3C DOM specifications are separated into multiple levels, where each level includes some required and optional modules. An application can claim support of a level by enforcing all the level requirements.
- Level 0:- The DOM 0 level was never formally specified. Instead, DOM Level 0 describes the techniques used to access HTML documents using JavaScript. These were introduced by web browsers such as Internet Explorer and Netscape Navigator before the standardization of the DOM.
- Level 1:- The goal of the DOM Level 1 specification is to define a programmatic interface for XML and HTML documents. Therefore, the DOM Level 1 specification is separated into two parts Core and HTML.
- Core:- It provides a low-level set of fundamental interfaces that can represent any structured document and define extended interfaces for representing an XML document.
- HTML:- It provides additional, higher-level interfaces used with the fundamental interfaces to provide a more convenient view of an HTML document.
- Level 2:- The Level 2 specification includes the following six specifications: Core2, Views, Events, Style, Traversal and Range, and HTML.
- Core2:- It consists of a set of core interfaces for developing and manipulating the structure and content of the document.
- Views:- It permits programs to dynamically access and updates the contents of an HTML or XML document.
- Event:- Events are scripts executed by the browser when the user reacts to the web page. Some of the standard methods are included addEventListener and handleEvent.
- Style:- It allows the programs and scripts to access the content of style sheets dynamically. The level 2 Style Sheet interface is the base interface representing any style sheet.
- Traversal and Range:- It permits programs and scripts to dynamically traverse and identify a range of content in a document.
- HTML:- It allows programs and scripts to dynamically access and updates the content and structure of HTML documents. It extends the interfaces defined in the DOM1 HTML, using the DOM2 Core possibilities. In addition, it introduces the contentDocument property, a helpful way to access the document contained in a frame.
- Level 3:- it consists of five different specifications: CORE3, LOAD and SAVE, VALIDATION, EVENTS, and XPATH.
Specifications
This section provides an overview of the DOM specifications, a set of APIs divided into several levels and modules.
DOM (Document Object Model in javascript) is a set of specifications recommended by W3C (World Wide Web Consortium) to define an API (Application Programming Interface) valid HTML and XML documents.
Conclusion:-
- The Document Object Model (DOM) is a representation of all the elements that make up a web page, from top to bottom.
- An HTML or XML document can be represented as a structure tree of nodes, like a traditional family tree.
- Each markup can be represented as a node with a specific node type.
- The W3C DOM specifications are separated into Four levels, level 0, level 1, level 2, level 3.