Dynamic Binding in Java
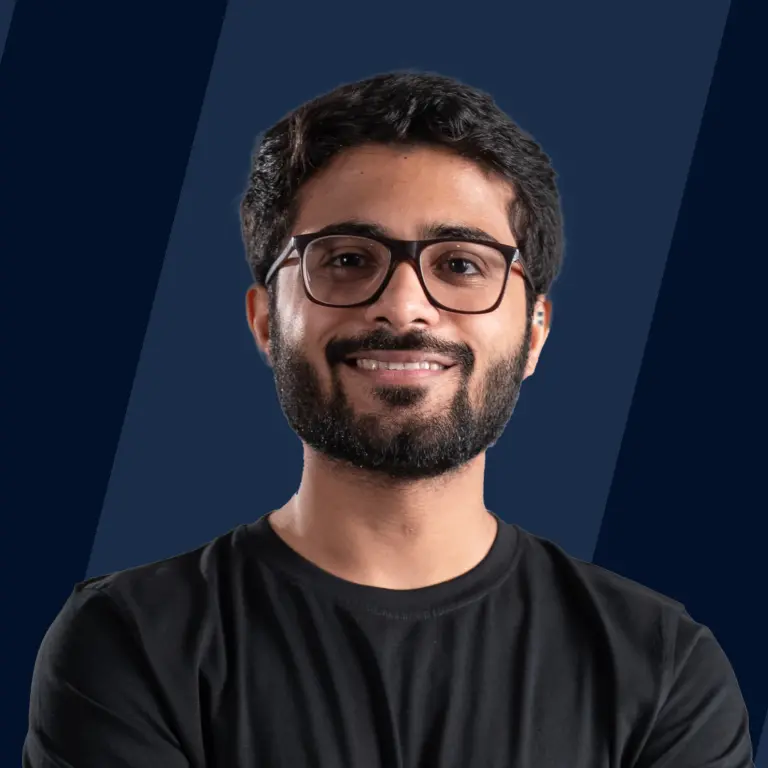
If the body of a method is bonded to a method call at runtime then it is called dynamic binding or late binding. This is because the method’s functionality is decided at runtime by the Java Virtual Machine.
Dynamic binding is also referred to as a run-time polymorphism. In this type of binding, the functionality of the method call is not decided at compile-time. In other words, it is not possible to decide which piece of code will be executed as a result of a method call at compile-time.
Overriding a method is a perfect example of dynamic binding in java.
How does Dynamic Binding Work in Java?
Consider two classes A and B such that A is the superclass of B. Assume B implements a method already implemented in its parent class, thus overriding it. Now, this means when a method is called on an object of the subclass type B, the implementation of the method in the subclass will be used, even if the method is invoked through a reference of the superclass type A.
We will discuss examples of this mechanism below. To implement Dynamic Binding or Runtime Polymorphism, following are the conditions:
Conditions:
- Inheritance: There must be a superclass and one or more subclasses that inherit from the superclass. The superclass should define the method that is overridden in the subclasses.
- Method Overriding: The subclass must override the method defined in the superclass. The method signature (i.e., the name and parameter types) must be the same in both the superclass and the subclass.
- Upcasting: The object of the subclass must be upcast to the type of the superclass. This is necessary because the reference variable used to call the method must be of the same type as the superclass.
- Method Invocation: The method must be called on the object of the subclass through the reference variable of the superclass. The implementation of the method that is invoked will be determined at runtime based on the actual type of the object being used.
Limitations :
- Private methods of the parent class cannot be overridden.
- Final methods cannot be overridden.
- Static methods cannot be overridden.
Example of Dynamic Binding
Java program to implement dynamic binding by overriding methods.
Output :
Explanation :
- In this code, there is a superclass called India that has a method called locate(), which simply prints "We are in India". The subclass SubIndia extends India and overrides the locate() method to print "We are in Delhi".
- In the main() method, an object of India is created and its locate() method is called, which prints "We are in India".
- Then, an object of SubIndia is created and upcast to the type of India. When the locate() method is called on this object using the India reference variable, dynamic binding comes into play and the overridden locate() method in the SubIndia class is called, printing "We are in Delhi". This is because the actual type of the object is SubIndia, and so its implementation of the locate() method is used.
- Finally, an object of SubIndia is created directly and its locate() method is called, which again prints "We are in Delhi".
Difference between Static and Dynamic Binding
Static binding | Dynamic binding |
---|---|
It is known as early binding as it takes place during compilation time. | It is known as late binding as it takes place during runtime. |
Static binding occurs during Method Overloading | Dynamic binding occurs during Method Overriding |
Static binding never uses real objects. | Dynamic binding always uses real objects. |
Conclusion
- If a body of the method is bonded to a method call at runtime then it is called dynamic binding or late binding.
- Overriding a method is a perfect example of dynamic binding in java.
- Dynamic binding or runtime polymorphism allows the JVM to decide the method's functionality at runtime.
- Dynamic binding is used when a subclass overrides a method defined in its superclass. In this scenario, the implementation of the method in the subclass is used, even if the method is invoked through a reference of the superclass type.
- On the other hand, static binding occurs during method overloading and takes place during compile-time. Unlike dynamic binding, it never uses real objects and is known as early binding.