Dynamic Memory Allocation in C
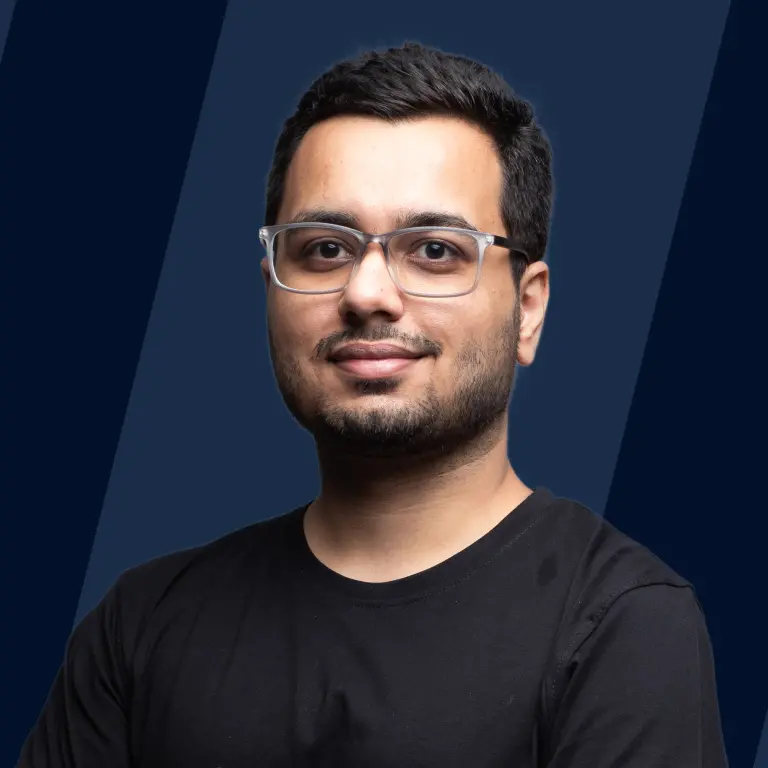
Comparing Static and Dynamic Memory Allocation, the former reserves memory at compile-time, which cannot be altered during execution. In contrast, the latter allows adjusting memory size at runtime via functions like malloc(), calloc(), and realloc() in C. Static allocation uses stack space, limiting flexibility. In contrast, dynamic allocation uses heap space, enhancing efficiency and adaptability for varying data sizes, exemplified in dynamic data structures.
Now, let us examine some of the differences between static memory allocation and dynamic memory allocation.
Aspect | Static Memory Allocation | Dynamic Memory Allocation |
---|---|---|
Memory Reservation Time | At compile-time, cannot be modified. | At runtime, can be modified. |
Usage | Known as compile-time memory allocation. | Known as runtime memory allocation. |
Allocation/Deallocation | Cannot allocate or deallocate memory during runtime. | Can allocate and deallocate memory during runtime. |
Memory Space Used | Uses stack space. | Uses heap space. |
Efficiency and Flexibility | Less efficient due to no reusability of memory while running. | More efficient due to reusability and flexibility of memory. |
Methods Used for Dynamic Memory Allocation in C
Dynamic Memory Allocation in C provides several functions to allocate, reallocate, and free memory at runtime, enabling flexible management of memory according to a program's needs. These methods are essential for creating data structures that can grow or shrink dynamically, such as linked lists or dynamic arrays.
There are four methods of Dynamic Memory Allocation in C.
- malloc()
- calloc()
- free()
- realloc()
Let's see each of them one by one.
Malloc Method in C
The malloc() function in C, crucial for dynamic memory allocation, allocates a specified byte size on the heap and returns a void pointer to this space. Found in <stdlib.h>, it does not initialize the allocated memory, leaving it with garbage values. Cast the void pointer to the desired data type to use the allocated memory.
Syntax
The general syntax for malloc() in C involves casting the void pointer to the desired data type:
For instance, to allocate memory for an integer:
This approach ensures compatibility across different compilers and operating systems by automatically adjusting to the size of data types.
Example
Output:
Explanation:
- The program dynamically allocates 1 byte of memory sufficient for a char type and assigns it to ptr.
- It checks if the memory allocation was successful (i.e., ptr is not NULL). If not, it prints an error message.
- If successful, the program assigns the character 'S' to the allocated space and prints it.
- This simple example illustrates dynamic memory allocation's power, enabling efficient and flexible memory management.
Calloc Method in C
calloc() in C is also a method that is used to allocate memory blocks in the heap section. Still, it is generally used to allocate a sequence of memory blocks (contiguous memory) like an array of elements. It is also present in <stdlib.h> header file.
Syntax of calloc()
- General Syntax:
calloc() in C is a function that takes two arguments, first is the size of the array (number of elements) and second is the sizeof() data type (in bytes) of which we have to make an array.
- Defined in header <stdlib.h> as :
- num : number of elements
- size : size of each element
- Example Syntax: int *arr = (int *)calloc(5, sizeof(int));
In the above statement, we are allocating an integer array of size 5 at runtime and assigning it to an integer pointer arr, arr will store the address of the very first element of the allocated array.
Example of calloc()
We will see an example of allocating a character array of size n using calloc() in C method.
C Program :
Input:
Output:
Malloc Vs Calloc
Now let's see the differnce between malloc and calloc in c:
malloc() method | calloc() method |
---|---|
It is generally used to allocate a single memory block of the given size (in bytes) during the runtime of a program. | It is generally used to allocate contiguous (multiple) blocks of memory of given size (in bytes) during the runtime of a program. |
It doesn't initializes the allocated memory block and contains some garbage value. | It initializes all the allocated memory blocks with 0 (zero) value. |
malloc() takes a single arugment i.e. the size of memory block that is to be allocated. | calloc() takes two arguments i.e. the number of elements and the size of one element that are to be allocated. |
syntax: (cast-data-type *)malloc(size-in-bytes) | syntax: (cast-data-type *)calloc(num, size-in-bytes) |
Example : float *ptr = (float *)malloc(sizeof(float)); | Example : float *ptr = (float *)calloc(10, sizeof(float)); |
free() Method in C
The free() function in C plays a critical role in memory management by deallocating memory blocks previously allocated with malloc() or calloc(). This helps prevent memory leaks and ensures efficient use of memory.
Syntax and Usage
Defined in <stdlib.h>, the syntax for free() is straightforward:
where ptr is a pointer to the memory block to be freed. Example usage is as simple as free(ptr);.
Examples
Output:
Explanation:
- Initially, memory for a single character is allocated using malloc(), used to store the character 'S', and then deallocated with free(). The pointer is set to NULL afterwards to prevent it from becoming a dangling pointer.
- Next, memory for an array of characters is allocated using calloc(), which initializes the allocated memory to zero. The array is used to store and print the string "Hello". After use, the memory is deallocated using free(), and the pointer is set to NULL to avoid dangling pointers.
realloc() Method in
The realloc() function in C is utilized for resizing a memory block previously allocated with malloc() or calloc(). This versatile function can either increase or decrease the allocated memory size and even allocate or deallocate memory.
Syntax
- ptr is a pointer to the memory block to resize.
- new_size is the new memory size in bytes.
- Example: int *arr = (int *)realloc(arr, new_size * sizeof(int));
Simplified Example
Output:
Explanation:
- An array of integers is initially allocated with malloc() for 5 elements.
- The realloc() function is then used to double the array's size.
- The newly allocated half of the array is filled with consecutive integers.
- The entire resized array is printed, showcasing the successful resize.
- Finally, the dynamically allocated memory is freed using free().
Conclusion
- Discussing what is Dynamic memory allocation in C (dma in C) is crucial for efficient memory management, allowing runtime allocation and deallocation.
- Functions like malloc() and calloc() in C allocate memory, realloc() adjusts the size of an allocated memory block, and free() deallocates memory.
- malloc() allocates uninitialized memory, whereas calloc() allocates zero-initialized memory blocks, making dynamic data structures like linked lists and arrays flexible.
- realloc() demonstrates the ability to resize memory allocations dynamically, which is particularly useful for data structures that need to grow or shrink during runtime.
- Proper use of these functions prevents memory leaks and ensures the efficient use of memory in C programs, exemplified by practical of dynamic memory allocation in c programming examples.