eval() in JavaScript
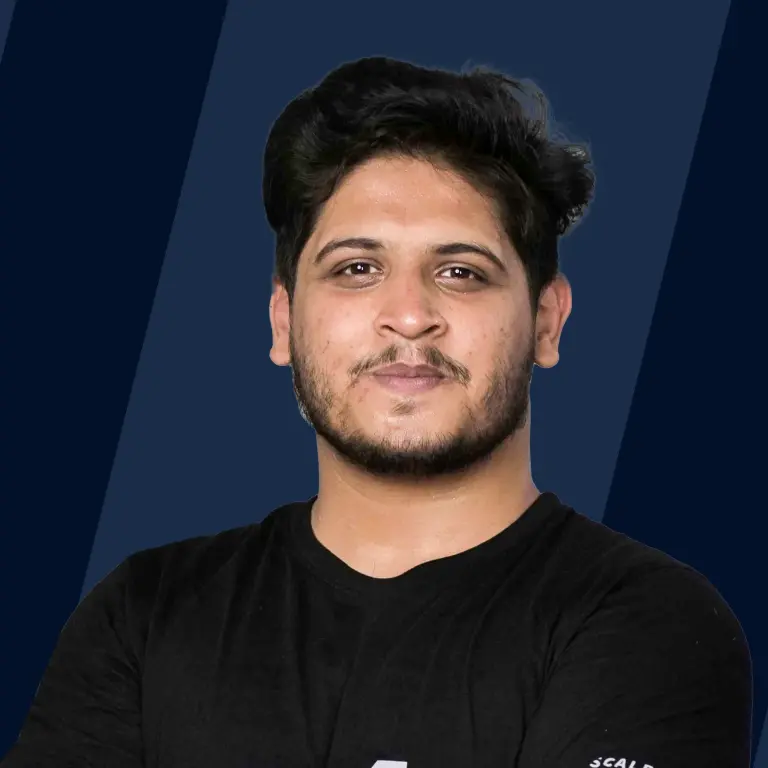
Overview
The eval() function in JavaScript is a global function (global functions are those functions that can be called directly without using an object) that is used to evaluate a string containing a JavaScript expression, a statement, or a sequence of statements. This function can help evaluate expressions that come as string inputs from the users.
Syntax of eval() in JavaScript
The following is the syntax of eval() in javascript:
Here expression is a string containing an expression or statement(s). For example, an expression could be "4 + 11" or alert("Hello").
Parameters of eval() in JavaScript
The function eval in JavaScript takes only one parameter as its argument:
- string - A string containing an expression, a statement, or a sequence of statements.
The expression/statement can contain variables, functions, or even objects.
Return Value of eval() in JavaScript
If the argument passed is a string, the value calculated after the evaluation of the given code is returned by eval in JavaScript. If there is no calculated value, undefined is returned.
Exceptions of eval() in JavaScript
If we pass a non-string argument to eval in JavaScript, it returns the same argument without any change.
For Example:
Output:
In the above example, we passed an array into the eval() function. Because it is a non-string argument, the function returned the same array without any changes to it.
Example
Output:
In the above example, we wrote a simple expression (a * 7) in a string. We used this string as an argument for the eval() function. Once the eval() function was executed, it multiplied the value stored in variable a with the number 7 and finally returned the product between the two numbers. Then, we stored this product in the output variable and printed it on the webpage.
How Does eval() in JavaScript Work?
The function eval in JavaScript takes a string argument. If the string contains an expression, eval() evaluates the expression. If the string contains a statement, eval() evaluates the statement. Even though the eval() function can be useful, it is usually recommended to avoid this function. Let us look at the reasons.
Why Should We Not Use the eval() in JavaScript ?
Following are the reasons why we should avoid eval():
- The eval() function is slow to execute because it invokes the JavaScript interpreter.
- If the string passed as the eval() function's argument contains malicious code, it could lead to malware attacks on the programmer's system/server.
- It makes code difficult to read.
More Examples of eval() in JavaScript
Let us look at a few more examples of eval() in JavaScript to get a complete understanding of the topic.
Example 1 - Using eval() to Call a Function
Output:
In this example, we passed a function with two arguments into the eval() function (as a string). We stored the value returned by eval() in the ans variable and finally displayed the result.
Example 2 - Using eval() with JavaScript Statements
Output:
In the above example, we wrote a conditional statement in a string. We passed this string as the eval() function's argument. Because the variable time was equal to 7, we got the output "Time to wake up!".
Example 3 - Passing a String Object as an Argument
Output:
In this example, instead of passing a string, we passed a string object into eval(). This string object contained the string "4 + 11". Now, because we passed a string object, the eval() function did not evaluate anything and returned the argument as it was. That is why we got 4 + 11 as the output and not 15.
Example 4 - Using eval() with a JSON Object
Output:
In this example, the string object_str contained data in the form of a JSON object. We used the eval() function to convert the string into a JSON object. We stored this JSON object in a variable job_desc. Hence, we were able to access the object properties by using job_desc.Company, or job_desc.Profile, etc.
Supported Browsers
The eval() function is supported by all major browsers, including -
- Google Chrome (version 1 and above)
- Mozilla Firefox (version 1 and above)
- Safari (version 1 and above)
- Opera (version 3 and above)
- Microsoft Edge (version 12 and above)
- Internet Explorer (version 3 and above)
Conclusion
- The function eval in JavaScript evaluates a string containing a statement or an expression.
- If a string parameter containing an expression/statement is passed as the argument, the eval() function returns the calculated value of the expression/statement. If there is no calculated value, the function returns undefined.
- If a non-string parameter is passed as the argument, the eval() function returns the parameter without any change.
- It is usually recommended to avoid the eval() function because it is slow and can even cause security threats in a system.
- The eval() function can be used to call a function, and it can even be used with JavaScript objects.