What is Event Delegation in JavaScript?
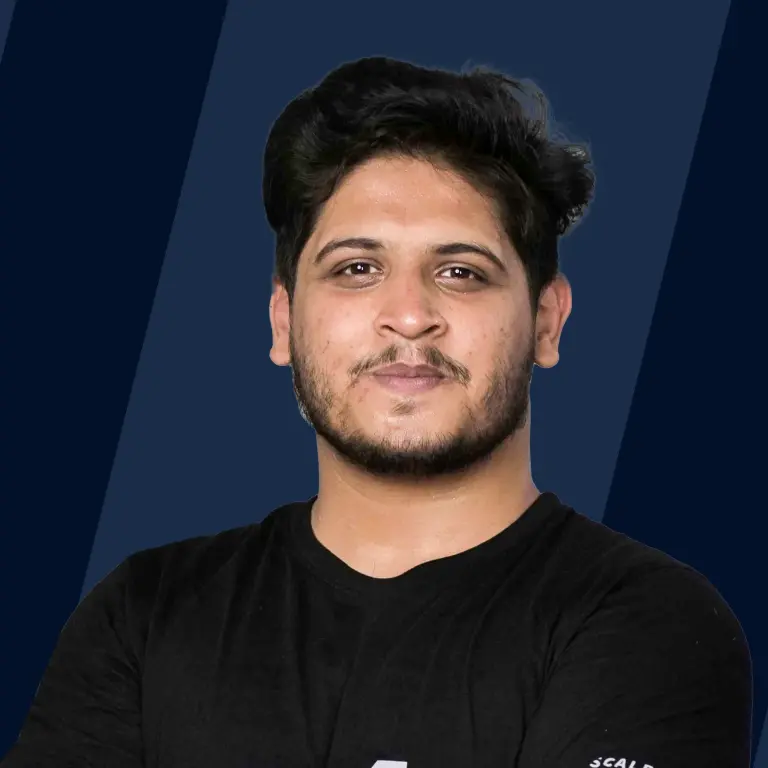
Event delegation in JavaScript is a pattern that efficiently handles events. Events can be added to a parent element instead of adding to every single element. It refers to the process of using event propagation (bubbling) to handle events at a higher level in the DOM than the element on which the event originated. This can be done on a particular target element using the .target property of an event object.
Some of the Common JavaScript Events
- change- the event handler used is onchange and when clicked, it changes the value of the form element.
- click- the event handler used is onclick and it performs an event when the mouse clicks on an element.
- mouseover- the event handler is called onmouseover, which performs an event when the mouse cursor comes over the element.
- mouseout- the event handler is called onmouseout and it performs an event when the mouse cursor leaves the element.
- keydown- the event handler is called onkeydown, which releases the key.
- load- the event handler is known as onload and it executes an event when the page gets loaded.
Example
Let's take an example and understand event delegation in JavaScript.
Steps to create event delegation:
- Create an HTML file
- Create a JavaScript file and use the function document.getElementById and give the id of the element where event delegation is to be applied.
- Use the function addEventListener by passing the parameter onclick and function(e). As soon as the events take place, the function is called which is passed to the listener.
JavaScript code
HTML code
CSS code
Output:
Why Event Delegation?
It is useful because the event can be listened to on multiple elements by using just one event handler. It also uses less memory and gives better performance. Apart from this, it also requires less time for setting up the event handler on the page.
The 3 Steps for Using Event Delegation.
Following are the steps for using event delegation:
- Determine the parent of elements to watch for events- In the example given, <table id = "mynewtable"> is the parent element of the table.
- Parent element is the one from which the feature is inherited in the child elements.
- Attach the event listener to the parent element- document.getElementById() addEventListener('click', handler), is used for attaching the event listeners to the parent element. This button when clicked reacts because of the event listener.
- Use event.target to select the target element- When the element is clicked, the handler function in JS is invoked with the event object as an argument. The event.target property is the element on which the event is dispatched.
JavaScript Event Delegation Benefits
It is possible to have a single event handler on the document for handling an event of a particular type. Using event delegation has the following benefits:
- Better performance and less usage of memory
- The time required for setting up an event handler on the page is less
- The document object is available immediately and the element is rendered perfectly without any delay.
- Easy syntax and usage
Browser Compatibility
It is flexible and has cross-browser compatibility. Event delegation is a better technique to handle events in web applications. Chrome on Windows and macOS- 104.0.5112.101 is compatible, however, do update your browser.
Conclusion
- Event delegation in JavaScript is a pattern that efficiently handles events. Events can be added to a parent element instead of adding to every single element.
- It is useful because the event can be listened to on multiple elements by using just one vent handler. It also uses less memory and gives better performance.
- The 3 steps to using event delegation
- Determine the parent of elements to watch for events
- Attach the event listener to the parent element
- Use event.target to select the target element
- It is flexible and has cross-browser compatibility. Event delegation is a better technique to handle events in web applications.