Event Handling in Java
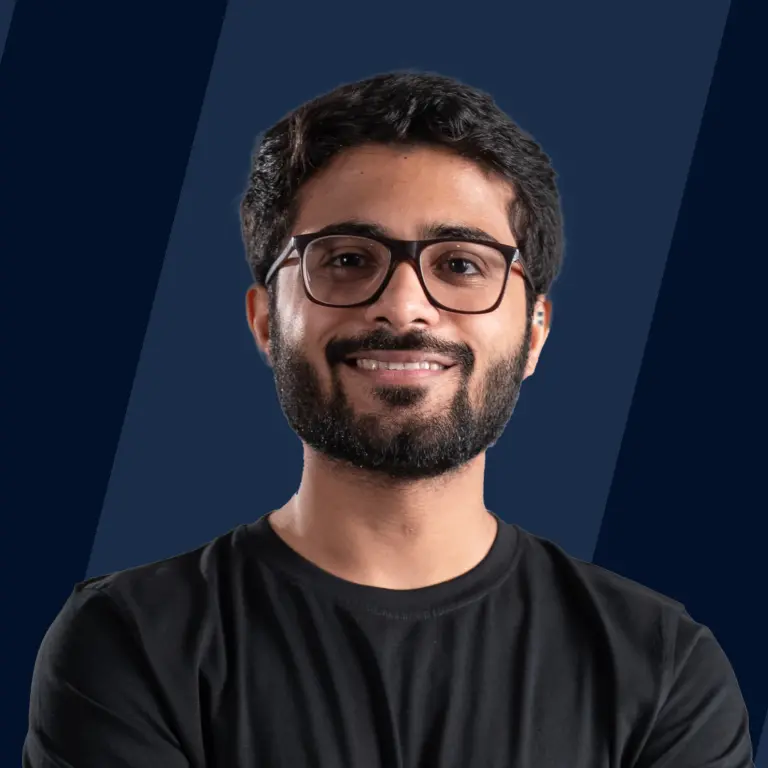
Overview
Event handling in Java is the procedure that controls an event and performs appropriate action if it occurs. The code or set of instructions used to implement it is known as the Event handler.
It consists of two major components:
- The event source and
- The event listener.
The source is the object where the event occurs, and the event listener is responsible for taking appropriate actions when an event occurs. These Listeners must be registered with the source object in order for the listener to receive event notifications.
What is Event Handling in Java?
Many event listeners are frequently used; recall the click of a button that takes you to another website or the mouse scroll? All of this is accomplished through the use of various event handlers, and the mechanism is known as event handling.
Events in Java
Events in Java represent the change in the state of any object. Events occur when the user interacts with the interface. Clicking a button, moving the mouse, typing a character, selecting an item from a list, and scrolling the page are all examples of behaviors that cause an event to occur.
Types of Events in Java:
- Foreground Events: These events necessitate the user's direct participation. They are produced as a result of a user interacting with graphical components in a Graphical User Interface.
- Background Events: Background events are those that require end-user interaction. Operating system interrupts and hardware or software failures are examples of background events.
Event handling in Java is the process of controlling an event and taking appropriate action if one occurs.
How are Events Handled?
The modern approach to event processing is based on the Delegation Model. It defines a standardized and compatible mechanism for generating and processing events. In this approach, a source generates an event and sends it to one or more listeners. The listener sits and waits for an event to occur. When it gets an event, it is processed by the listener and returned. The user interface elements can delegate the processing of an event to a different function.
The image below shows the flow chart of the event delegation model.
Event Handling in Java Example
The example below shows how you can handle events in Java. In this example, we will create a frame using Java and add a button. Once the interface is created, we will add one event listener to the button, which will change the background and foreground (text) color on click. Another event listener is activating dispose of the frame, that is, the x button on the top-right of the frame.
Output:
The initial output of the program is the user interface that we discussed above.
Now, once you click on the click me button, the font, font color, and background color for the button are shown in the image below.
Components of Event Handling in Java
An Event Model is fundamentally composed of the three elements listed below:
- Events Handler
- Event Sources
- Event Listeners
In the subsequent sections, we will go over each one in-depth.
1. Event Handler
An event handler is a function or method that executes program statements in response to an event. A software program that processes activities such as keystrokes and mouse movements is what an event handler is. Event handlers in Web sites make Web content dynamic.
2. Event Sources
An object on which an event occurs is referred to as a source. The source is in charge of informing the handler about the event that occurred. There are various sources like buttons, checkboxes, lists, menu-item, choices, scrollbars, text components, windows, etc.
3. Event Listeners
When an event occurs, an object named an event listener is called. The listeners need two things: first, they must be registered with a source; however, they can be registered with multiple sources to receive event notifications.
Second, it must put in place the methods for receiving and processing notifications. A set of interfaces defines the methods for dealing with events. The Java.awt.event package contains the following event classes and interfaces.
Event Classes and Listener Interfaces in Java
Event Classes | Description | Listener Interface |
---|---|---|
ActionEvent | When a button is clicked or a list item is double-clicked, an ActionEvent is triggered. | ActionListener |
MouseEvent | This event indicates a mouse action occurred in a component | MouseListener |
KeyEvent | The Key event is triggered when the character is entered using the keyboard. | KeyListener |
ItemEvent | An event that indicates whether an item was selected or not. | ItemListener |
TextEvent | when the value of a textarea or text field is changed | TextListener |
MouseWheelEvent | generated when the mouse wheel is rotated | MouseWheelListener |
WindowEvent | The object of this class represents the change in the state of a window and are generated when the window is activated, deactivated, deiconified, iconified, opened or closed | WindowListener |
ComponentEvent | when a component is hidden, moved, resized, or made visible | ComponentEventListener |
ContainerEvent | when a component is added or removed from a container | ContainerListener |
AdjustmentEvent | when the scroll bar is manipulated | AdjustmentListener |
FocusEvent | when a component gains or loses keyboard focus | FocusListener |
Steps to Perform Event Handling in Java
The following steps are required to perform event handling in Java:
Implement appropriate interface in the class: The first step is to implement an appropriate interface in the class. For the example we used above, this step can be the implementation of frame and mybtn objects, i.e. simply creating them.
This code shows how we created frames and buttons and added buttons to the frame.
Register the component with the listener: Once we are done with the implementation of the interface in the class, the second step is to register the created components with listeners, which can be done using inbuilt functions. In our example, we added two event listeners.
- One was to make the button responsive to click operation, see the code below.
- We registered our frame object with the windows listener and commanded it to dispose of the frame on interaction.
Java Event Handling Code
Within Class
The code below demonstrates how event handling can be implemented in a single class. In this example, we'll make a user interface with a button and a text field inside a frame, and we'll handle the button's click event so that the text inside the text field appears when you click the button.
- Firstly we created a class named MyClass that extends the inbuilt Frame class in Java and implements the ActionListner interface.
- Then created a text field object and inside the constructor of the class initialize it to a new empty textfield and set the bounds for it.
- Then the button is created and added and ActionListner passes the current instance of it.
- Then both of them are added to the extended frame and our UI is ready.
- Now we manipulate the abstract actionPerformed method, to make a response for the ActionListner added above, we command it to display "Hello" in the text field.
- Then we created an object of MyClass and the output is performed accordingly.
Output
Other Class
The same example of event handling in Java can be implemented using another class, the code for the same is given below.
- We created the same UI, we used in the previous example using the same approach.
- The only difference is that we used another class named Outer class which creates the object of MyClass and overrides the actionPerformed function for this object.
- The output of this is exactly the same as that of the previous example
Anonymous Class
Another way of creating an event handling programme in Java is using anonymous class implementation. The code below shows how you can implement using an anonymous class.
The benefit of using anonymous class implementation is that we don't have to explicitly create another class to implement the actionPerformed method as we did in the previous example.
- Firstly we created the UI similar to the previous two examples, inside the class named MyClass which extends the Frame class in Java.
- Once we have created the text field and button objects, we added the same ActionListner to the button object using anonymous class implementation.
- Here we can override the actionPerfomed method, instead of manipulating it separately inside the same class or in another class.
- The output is the same as that of the previous two examples.
Conclusion
- We have covered the basics of event handling in Java along with its implementation techniques.
- Event handling in Java is controlling an event and taking appropriate action if one occurs. Events handling makes web pages and mobile applications live.