What is Exception Handling in JavaScript?
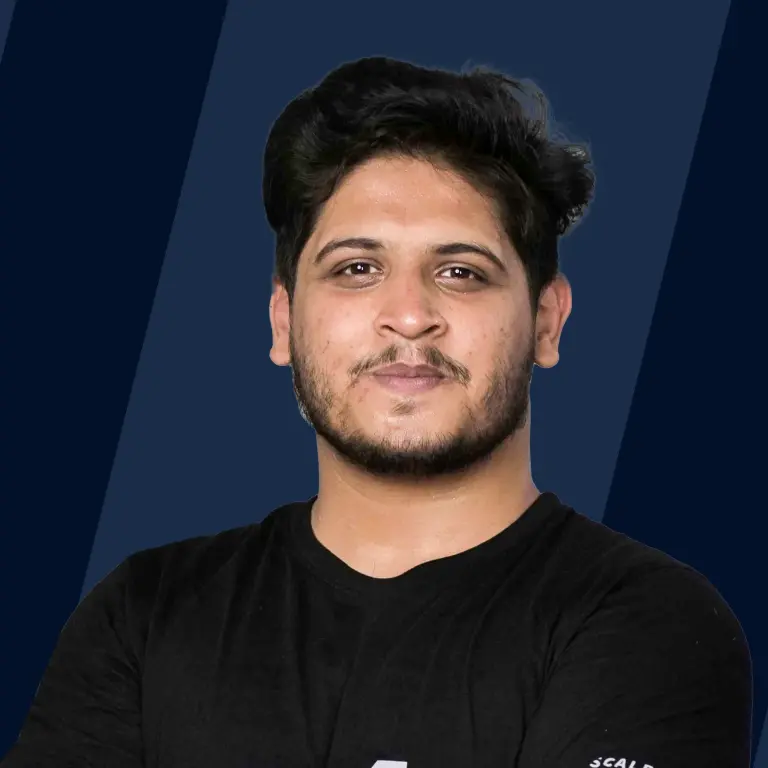
Exception handling in Javascript is one of the powerful features for handling errors and helps to avoid the sudden crashes of our programs and applications. Additionally, it helps to maintain the normal flow of our programs.
As the name suggests, Exception handling is made up of two words. The first word Exception is known as an unexpected or abnormal condition that occurs during the runtime or execution of a program. And second-word handling means how we can handle those unusual situations.
Exception handling in Javascript is the process of handling abnormal statements that occur during the execution of a program.
And it also helps in tracking errors, which means that the exception explains why the error occurred and when and where it happened in the code.
Example
For a better understanding, let me give you a simple example. Imagine that you have built a file management system that stores files provided by users in a database. Now the user stores a file named "important" in the database, and it gets accidentally deleted from the database. Unfortunately, you never thought about this situation because the user has no way to delete the stored file, and you ignored the problem and never handled it in your code.
When the user tries to access his file, your program crashes because you have never written the code to handle this situation. That is where the importance of Exception Handling in JavaScript comes in because you have to consider the constraints, and the code has to be written so that it never completely crashes the application. Instead, it throws an error, and the code continues the normal flow of the program.
The fundamental causes of exception errors are:
- Whenever we try to divide any numbers by 0, this leads to infinity, and as a result, the exceptions are shown.
- When we try to call any files that don’t exist within our system.
- When the input provided by the user is wrong.
- When our network connection is down.
As a software engineer or developer, you have to create a plan for failures or exceptions to make the project successful.
When the code execution raises an exception, it goes ahead, and the javascript translator looks for the exception handling code where the exception will be evaluated.
Then, based on the current errors, the result will be executed by different statements. To understand this better, let's look at the types of javascript errors.
Types of Errors
During the execution of JavaScript code, three types of errors can occur:-
- Syntax error
- Runtime error
- Logical error
Let's understand each of them in a little more detail.
1. Syntax Error:- Syntax errors appear when the user makes a mistake in the predefined syntax of the javascript language. The interpreter cannot interpret this error raised during the code compilation.
These errors are:
- Spelling errors (wrong spelling, such as fiction instead of function).
- Ignoring essential characters, such as failing to use a semicolon to end a statement.
- Incorrect indentation or formatting
And most importantly, these errors stop the program from working until we correct the mistake.
2. Runtime Error:- Runtime Error occurs during the program's execution and gets detected only when you run the program; that's why it's known as a runtime error. And these errors suddenly crash the program or applications, and that's where exception handling became useful, and via the help of it, we can maintain the normal flow of the program.
These types of errors occur when:-
- This is caused by scenarios such as division by 0 and integer overflow.
- Runtime error is also caused by the segmentation faults such as Accessing an array out of bound.
As a developer, it's your responsibility to handle these types of errors.
3. Logical Error:- Logical Error occurs when there is any logical mistake in the program that may not produce the desired output and may terminate abnormally. These types of errors do not throw an error message or an exception.
The reason is that the code is showing the result but not doing the same thing the developer intends. Unfortunately, finding logical errors can be challenging, and you must go through manual testing to detect these types of errors.
Error Object
Whenever an error occurs during the execution or runtime of the program, the code stops unexpectedly, and JavaScript raises an exception scenario with the Error object thrown by the throw statement.
The error object is thrown by JavaScript, or the user can create a user-defined error object with the help of the throw statement.
The error object has two main properties:
- name:- error name stores or returns the name of the error.
- Message:- Whereas error message gives or describes the error message in string form in JavaScript.
Although the error is a generic constructor as it gives basic information about the condition, on the other hand, various standard built-in error types or error constructors are the foundation of exception handling in javascript as they provide detailed information about the problem.
Standard Built-in Error Types or Error Constructors
These are the built-in standard error types or error constructors, and they are helpful for exception handling in javascript.
Let's discuss each of them in more detail:-
1. EvalError:- This type of error occurs in the eval() function and indicates an issue with its arguments. Additionally, the eval() function is the global function that takes a string with javascript code and evaluates it for you.
Additionally, EvalError is not used within the current ECMAScript specification. However, the object itself remains for backward compatibility with earlier versions of the specification.
The above throw statement creates and throws an error object with the EvalError constructor.
You must avoid using the eval() function because it costs security and performance issues.
2. ReferenceError:- ReferenceError occurs when the code uses undeclared variables.
Whenever the code tries to access undeclared variables, the error occurs as a reference error.
In the above code, we have created a variable known as a number, but then we are trying to access it with the count name, which is not even defined in our code.
In this case, javascript throws an error which says:-
3. InternalError:- This error is directly thrown by the js engine when the JS engine is overwhelmed by too many recursions, or the stack grows over the limit.
The internal error occurs when too many switch cases are used, or too much recursion happens.
The Above code is an example of recursion.
4. RangeError:- This type of error occurs when a numeric value is out of a valid range.
Above, we have an array known as an arr which contains two elements, but then we try to increase the array's length by 90**99 == 2.9512665430652753e+193 elements.
The above number to increase the length of the array is way too big, and it passed the maximum size of the array, So the range error occurred.
5. SyntaxError:- This error occurs when the programmer misuses the syntax or breaks the javascript rule.
In the above code, we declared a variable, but the given name breaks the rule of variable declaration as we cannot use the space between the variable names.
The above code throws an error that says:-
6. TypeError:- It occurs when the given value is unexpected or you are trying to operate on the wrong data type.
In the above code, we are trying to make a number variable uppercase, which is possible only if we have a string data type inside that variable.
And because we are trying to perform an operation that is possible only on a string type, it throws an error.
7. URIError:- This error occurs when you pass invalid parameters to the encodeURI() or decodeURI() functions.
Whenever we give the wrong parameter to the URL function, a URL Error is thrown by Javascript.
Exception Handling Statements
An exception is an abnormal or unexpected condition that occurs during the runtime, and javascript provides various statements or a combination of statements to handle the exceptions.
It handles exceptions in try-catch-finally statements and throws statements.
Before we explore each statement, let's get a basic understanding of these terms:
- try{}:- The try block will contain the code, which may cause exceptions during the execution. If any errors occur, It stops the execution immediately and passes the control to the catch block.
- catch{}:- The catch block contains the code that will only execute when the errors occur in the corresponding try block. If the try block is executed successfully, then the catch block will be ignored.
- finally{}:- The code placed inside the "finally" block will be executed whether an error has occurred or not.
- throw:- The throw keyword or statement is used for throwing custom or user-defined errors.
Now that you know about all these let us move ahead and discuss exception handling statements.
Throw Statements
The throw keyword is used to throw custom or user-defined errors, which means you can create an error object with the message and name property. Additionally, you can use anything as an error object, maybe even a primitive, like a number or a string.
The syntax is:
Example:-
The following example demonstrates how to use the throw statement.
- In the above code, we have created a function that takes two values, x and y, and inside that function, we are dividing x by y.
- Also, we are checking the value of y. if the value of y is equal to 0, then we have to throw a custom error, or else we will do our calculation and print the output on the console.
- Whenever the value Y is equal to 0, we throw a custom error which later will be caught by the catch block, and it prints the error message.
Let's call the function with two different values and analyze the output:
Output:
- The output of the first function call is 3 because 30 divided by 10 is 3.
- The output of the second function is the custom error message thrown by the throw statement because we have passed the Y value equal to 0.
Try…catch Statements
The try block contains the code that will be executed successfully if no exceptions occur. Still, in the case of an exception or error, code execution in the try block stops immediately and passes the control to the catch block.
The catch block contains code that will only be executed when the try block throws an exception. You want to succeed in the try block, but if it does not succeed and generates an error, the catch block takes control and takes care of that situation.
In general, if the try block is executed successfully, the catch block will be ignored without causing any issues.
The syntax is:
Example:-
In the above, we have the try-and-catch blocks that contain some code.
- The execution of the code started and worked fine till line number 3, and then found a function that was not defined in the code.
- it stops the execution and passes the control to the "catch" because the try throws an exception.
- The catch block then prints the error on the console.
output:-
You can see that console.log('Not Executed'); never gets executed because the try block stops execution when it throws an error. The catch block then prints the error on the console.
try…catch…finally Statements
The try and catch block is executed based on certain things; the try block will be executed until it encounters an error, and the catch block is only executed when the try block throws an exception. But "finally block" doesn't care about both blocks.
In general, the " finally block" will be executed whether an error has occurred or not.
The syntax is:
Example:-
- The above code creates a function that takes two values, x and y.
- The try block tries to compare the y value equal to 0. If the condition meets, it throws a custom error, or else it will divide the x by y and print the answer on the console.
- The catch will print the error message thrown by the try block.
- The final block always gets executed and prints both given values on the console.
Let's call the function with two different values and analyze the output:
output:-
The Output of both functions contains the value of the "finally " block of code.
Utilizing Error Objects
An error object provides the name and message properties; depending on the error type, you can use these properties to get a more sophisticated message.
The name property provides an idea about the class of error we discussed above, and the message property provides a detailed error message in javascript string form. Whenever you throw custom exceptions, you can take advantage of these properties to identify your exception or the system-generated exception.
Conclusion
- Exception handling in Javascript is the process of handling abnormal statements that occur during the execution of a program.
- Three types of errors occur during the execution of the program Syntax error, Runtime error, and Logical error.
- whenever an error occurs, it throws an exception with the error object.
- try, catch, finally and throw are various javascript statements that are helpful for exception handling in javascript.
- You can combine javascript statements to create more accurate exception-handling statements.
- By utilizing the Error object and throw statement, you can easily create more specific exceptions and differentiate them from system-generated exceptions.