File Handling in Java
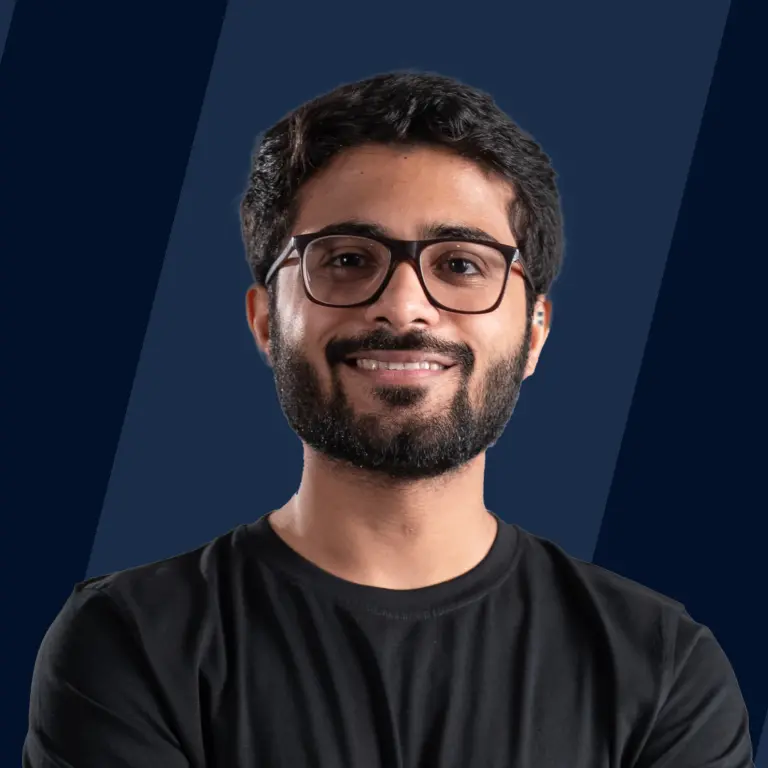
A file is a designated location where associated information is stored. Java provides Files as an abstract data type. Java provides multiple file operations to create new files, read, update, and delete. This makes file handling in Java easy.
Why File Handling is Required?
Suppose you need to append a "Happy Diwali!" message line at the end of the 1000 files that you want to print, so doing it manually will be tedious. Java's file handling operations can lessen your work as tou can append the "Happy Diwali" line in 1000 files by writing few lines of code.
Java File Handling is a way to carry out read and write operations on a file. Java's package "java.io" contains a File class, enabling us to work with different file formats.
To understand File operations, knowledge of File methods and Stream are required, so we'll cover them.
Streams in Java
In English, the stream is a continuous flow of water. Similarly, in java stream means a sequence of data. Based on the data type that a Java stream comprises, it is of two types :
- Byte Stream - Comprises of bytes.
- Character Stream - Comprises of character
Byte streams are comprised of bytes. Byte stream carries out 1 byte (8-bits) input and output operations. ByteStream classes read/write the data of 8-bits. InputStream and OutputStream are two abstract classes at the top of the hierarchy that defines a byte stream.
a. InputStream- Used to read data from a source.
b. OutputStream- Used to write data to a destination.
Input Stream
InputStream and OutputStream are superclass and have a number of concrete classes that deal with different devices like disc files, network connections, and so on.
Classes for Input and Output Streams:
InputStream and OutputStream classes are abstract classes. While InputStream is the superclass of classes representing byte stream input, the OutputStream is the superclass of all classes representing byte stream output.
- Class FileInputStream is declared as a public class FileInputStream extends InputStream
- Class BufferedInputStream is declared as a public class BufferedInputStream extends FilterInputStream Similarly, we can generate others.
InputStream classes | Description |
---|---|
FileInputStream | Reads data from a file |
ByteArrayInputStream | Data is read from a byte array |
FilterInputStream | Has subclasses BufferedInputStream, DataInputStream and PushbackInputStream |
BufferedInputStream | Used to read information from a stream. Internal buffer mechanism enhances performance. |
DataInputStream | Reads in Java's built datatype from an input stream. |
PushbackInputStream | Reads byte data from inputstream and returns it back to the stream |
PipedInputStream | It reads data bytes from one thread that are sent to PipedOutputStream for writing on another thread. |
ObjectInputStream | Deserializes the inbuilt data types and objects that are written using ObjectOutputStream. |
Basic Input Stream Operations:
The Input Stream class is an abstract class, so we can't construct objects of this type. We'll go through some of the methods. We'll look at what these input stream methods do.
Method Syntax | Description |
---|---|
int read() | Returns the next byte as int stream. Returns -1 when it approaches the end of stream. If an I/O error occurs, an IOException exception will be thrown. |
int read(byte[] array) | Used for reading byte of data from the input stream and stored in an array. The maximum number of bytes read is array.length. The data will not be returned until the stream reaches the end. The procedure will not return the end of bytes if it reaches the end. |
int read(byte[] array, int offset, int length) | It functions similarly to the other methods, with the exception of the length. |
Output Stream
- Class FileOutputStream is declared as public class FileOutputStream extends OutputStream
- Class BufferedOutputStream is declared as public class BufferedOutputStream extends FilterOutputStream
Similarly, we can generate others.
OutputStream classes | Description | Syntax |
---|---|---|
FileOutputStream | Writes data to a file | FileOutputStream fout=new FileOutputStream(<pathname_of_file>); |
ByteArrayOutputStream | Writes data to multiple files. By default, biffer size is 32 bits. | ByteArrayOutputStream bOutput = new ByteArrayOutputStream(int bufferSize); |
FilterOutputStream | Has subclasses named BufferedInputStream, DataOutputStream and PrintInputStream | FilterOutputStream filter = new FilterOutputStream(OutputStream fout); |
BufferedOutputStream | Uses internal buffer to store data. More efficient and faster than writing data into the stream. | BufferedOutputStream bout=new BufferedOutputStream(OutputStream fout, int buffer_size)); |
DataOutputStream | Enables to write Java's built datatype to output stream. It is machine-independent. | DataOutputStream data = new DataOutputStream(OutputStream fout); |
PrintStream | It has methods to write data into other stream without throwing IOException | PrintStream out=new PrintStream(OutputStream fout); |
PipedOutputStream | Used with PipedInputStream. They are connected using connect() method of PipedOutputStream. It writes data that are provided by PipedInputStream. | OutputStream output = new PipedOutputStream(PipedInputStream pipedInputStream); |
ObjectOutputStream | Used with ObjectInputStream.Writes the inbuilt data types and objects to an ObjectOutputStream. | ObjectOutputStreamDemo oot = new ObjectOutputStreamDemo(OutputStream fout); |
Basic Output Streams:
Three write methods in Output Stream are used for writing data into a stream. These methods are similar to the read() methods of the Input Stream class.
For writing to a file, use FileOutputStream.
- ObjectInputStream — This is used to write objects to a stream.
- ByteArrayOutputStream — This is used to write a byte array.
- PipeOutputStream — This is used to write data to a piped stream.
- FilterOutputStream - This filters the output of an end stream.
Standard Streams:
In most cases, the number of standard streams specified by OS is 3. System is a java.lang package member that offers system functions such as standard input, output, error, etc.
-
Standard Input Stream: It is implemented by System.in. It takes characters from the keyboard and reads them. read() function of System.in`` reads a single character by using System.in.read(). If there is nothing to read it returns -1`.
-
Standard Output Stream: It is implemented by System.out. It's a stream that outputs its data to the screen or display.
Implementation of the above two streams in a program :
Code explanation: System.in.read() takes single character as input from keyboard and System.out.println() displays the total characters inputted.
- Standard Error Output Stream: It is implemented by System.err (Syntax: System.err.println()). It is used to print error(s) occurring during the execution of a program.
If any error occurs, System.err.println() will print an error message.
Java File Class Methods
We'll go through the java file class method available for file handling. File object is created by passing in a string that represents the name of a file.
Methods | Description | Syntax |
---|---|---|
canRead() | Determines whether a file is readable or not. | boolean var = file.canRead() |
canwrite() | Determines a file is writeable or not. | boolean var = file.canWrite() |
createnewfile() | Generates a new file with no content. If the abstract file path does not exist, the method returns true, and a new file is created. If the filename already exists, it returns false. | boolean var = file.createNewFile(); |
exists() | Determines if the abstract filename denotes an existing file or directory. If the abstract file path exists, the method returns true; otherwise, it returns false. | boolean var = file.exists(); |
delete() | Deletes the directory or file denoted by the abstract pathname. The method returns true if the file or directory deleted successfully, otherwise returns false. | boolean var = file.delete(); |
getname() | The Name of the specified file object is returned by this function. A null string is returned if the abstract path does not have a name. | String var= file.getName(); |
getabsolutepath() | The absolute pathname of the specified file object is returned by this method. If the file object's pathname is absolute, it just returns the current file object's path. | String var= file.getabsolutepath(); |
length() | The length of the file specified by this abstract pathname was length is returned by this function. If the file does not exist or an exception occurs, the function returns a long value that indicates the number of bits, otherwise it returns 0L (long type). | long var = file.length(); |
list() | Returns lists the names of files and folders in a given directory | String[] paths= file.list(); |
mkdir() | Creates a new directory using the abstract pathname as its name. If a directory is created, the method returns true; otherwise, it returns false. | boolean var =file.mkdir() |
File Operations in Java
In this section, we'll go learn how to work with Java Directories. A directory is a file system structure containing files and other directories.
Fig: Child directory has files hello and project while Parent directory contains Child directory
Create and modify directory Java has different methods for creating new directories and modifying the old ones.
METHOD | DESCRIPTION | EXCEPTION THROWN | Syntax |
---|---|---|---|
mkdir() | Creates a new directory using the abstract pathname as its name. Returns true if a directory is created, otherwise false. | SecurityException when creation of directory is denied. | boolean var = file.mkdir() |
mkdirs() | Creates a new directory specified by the abstract pathname, along with all of the abstract pathname's non-existent parent directories. Returns true, if a directory is created, otherwise false. | SecurityException when creation of directory is denied. | boolean var = file.mkdirs() |
createnewfile() | Generates a new file with no content. if the abstract file path does not exist and a new file is created, it return true. If the filename already exists, it returns false. | IO Exception when input output error occurs; Security Exception when write access is denied. | boolean var = file.createNewFile(); |
setreadonly() | Restricts access to the given file or directory to read activities only. | SecurityException, when write access, is denied. | boolean var = setreadonly() |
renameTo() | Renames a File's abstract pathname to a specified pathname. Returns true if the file is renamed, otherwise false. | Security Exception when the method denies the write operation of the abstract pathnames.NullPointerException when the given filename is null. | boolean var =file.renameTo(File destination) |
delete() | This method deletes file or directory given by abstract pathname. | NoSuchFileException when the file does not exist. DirectoryNotEmptyException when a directory could not otherwise be deleted as it is not empty.IOException when an I/O error occurs.SecurityException delete access is denied. | boolean var =file.delete() |
Querying Files and Directories Java provides methods that can be used to get information about a file or a directory like if the file exists or not. This is called querying files and directories.
METHOD | DESCRIPTION | EXCEPTION THROWN | SYNTAX |
---|---|---|---|
exists() | Checks if the abstract filename denotes an existing file or directory. Returns trueIf the abstract file path exists, otherwise false. | Security Exception | boolean var = file.exists(); |
isfile() | Checks if the abstract filename represented by the abstract filename is a file or a directory. Returns true, if the abstract file path is File, otherwise false. | Security Exception | boolean var = file.isFile(); |
canread() | Checks if the file indicated by the abstract pathname can be read or not. returns true if the file path exists and the program is permitted to read it, otherwise false. | Security Exception | boolean var = file.exists(); |
canwrite() | Checks whether the program is capable of writing the file specified by the abstract pathname. Returns true, if the abstract file path exists and the program is authorized to write to it, otherwise false. | Security Exception | boolean var = file.canRead() |
canExecute() | Checks if the file indicated by the abstract pathname can be executed or not. check if the file path exists and the program is permitted to execute it, otherwise, false. | Security Exception | boolean var = file.canExecute() |
Table showing methods, descriptions, and exceptions thrown.
Conclusion
-
Java File Handling is a way to carry out read and write operations on a file using “java.io” package.
-
Stream means a sequence of data. There are two types of stream byte stream and character stream based on their composition.
-
write() operation throws 5 types of exceptions namely NonWritableChannelException, ClosedChannelException, AsynchonousCloseException ,ClosedByInterruptException and IOException.
-
In java , File Class provides various methods like canread(), canwrite(), exists(), delete() etc to for file handling.