Find in Python | find() function in Python
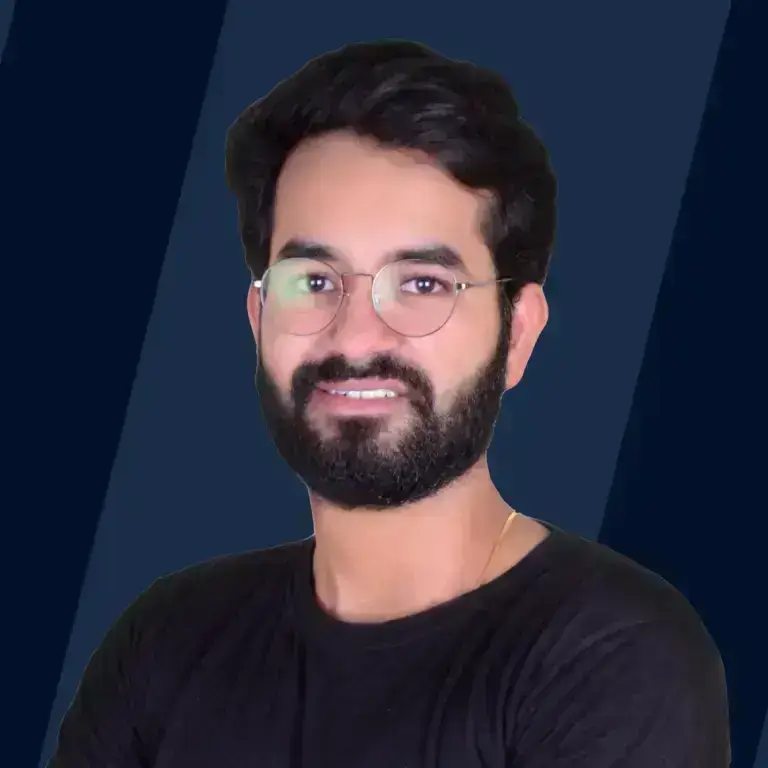
The find() function in Python is crucial for locating the index of the first occurrence of a substring in a given string, returning -1 if not found. Alongside find(), rfind() and index() are also explored. These functions prove valuable in tasks like word searching, essential for features like Find and Replace in text editors. Counting substring occurrences is illustrated using a simple Python loop.
Syntax of find() Function in Python
- string is the original sentence/word in which you want to look for the substring.
- substring is the sentence/word that you have to search.
- start and end are optional parameters. They specify the range where the substring needs to be searched.
What does find() Python Return?
- If the substring exists in the string, it will return the index of the first occurrence of substring.
- If the substring does not exist, it will return -1.
Here is an example,
Output:
In this code, we created a string variable named paragraph, and we called find() on it to find the occurrence of "I" and "q". Here, "I" comes at the very start of the string, so its index 0 is returned, but in the second case "q" is not found, so we get -1
Let's look at different types of examples:
1) No start and end arguments are given
Output :
Here we have created a variable str which contains a sentence, and then we called find() on str and gave it string python as the argument. So, find() will search for 'python' in str and return the index of its first occurrence in str
When start and end arguments are not given, then find() starts to look from the beginning and searches till the end of the string.
What if we use default arguments?
Output:
If we do not pass start and end arguments, then their default values are used. The default value of start is 0, and end is length of the string.
2) Start and End arguments are given
Output
Here, we have made a variable called str and have stored a sentence in it. Then we call find() on it and gave it 'interests', '10' and '30' as arguments. '10' tells find() that it has to start looking for the substring (i.e interests) from the 10th position and '30' tells find() to look till the 30th position and not beyond that.
So, start and end arguments set a range and find() searches for the substring only in that range.
'interests' comes at 16th position, which is between 10 and 30, so for the first print(), the output is 16, and for the second, it is -1 as 'interests' is not present between 30 and 40.
3) Only Start Argument is given
Output:
Here again, we have stored a sentence in a variable called str, and we call find() on str and pass 'interests' and '10' as arguments. '10' here tells find() that it has to start looking from the 10th index, and since there is no end argument, it will look until the end of the string.
When only start is given, the end automatically takes the default value, i.e. length of the string. So, here we define the starting range but not the ending range.
'interests' comes at 16th position, so for the first print() the output is 16 and for the second it is -1 as 'interests' is not present after 16.
Can we find the position of a whole sentence?
Yes, we can!
Output:
In the find() you can pass a whole sentence as a input. Here we have passed '*very early*' as input to find() and find() searchs for '*very early*' in str and returns 10 as position of '*very early*', which is the correct position.
What is rfind() in python?
rfind() is similar to find() but there is a small difference. rfind() returns the highest index of the substring. Similar to find() it returns -1 if the substring is not found.
Its syntax is the same as find() and takes the arguments that find() takes.
Here is an example which will make things clear.
Output:
Here, we fist created a string variable named myStr and called find() and rfind() on it. find() returned 0 and rfind() returned 9.
This is because, rfind() returned the index of the last occurence of 'py' while find() returned the index of the first occurence of 'py'.
Python String Index()
index() function is also a library function of Python. This function gives the poisition of the substring just like find() but the difference is index() will throw a ValueError if the substring is not present in the string. In such a case, find() returns -1.
Output:
Here you can see that find() returned -1 whereas index() threw an substring not found error.
How to find total occurences of a substring?
It is also a simple task, and we can do this using a loop and a find().
Output:
In this code, first, we have set the value of start and occurrences to 0, and then we used a for loop to iterate over the string repeatingStr.
Inside the loop, we call find() to check if the substring 'repeating' is inside repearingStr or not.
If it is present, we add 1 to the value of start and increase the value of occurrences by 1. The reason we add 1 to start is so that the substring is not counted twice.
When the loop is completed, the value of occurrences is printed.
Conclusion
- find() returns the first occurrence of the substring in the given string. It will return -1 if the substring is not present.
- find() can take 3 parameters: substring, start, end. The start and end are optional.
- start and end are useful when searching for a substring at some specific position inside a string.
- rfind() is similar to find() but it returns the last occurence of the substring. It also returns -1 if the substring is not present.
- index() is also a similar function, but it will throw a Value Error if the substring is not found.