floor() in C
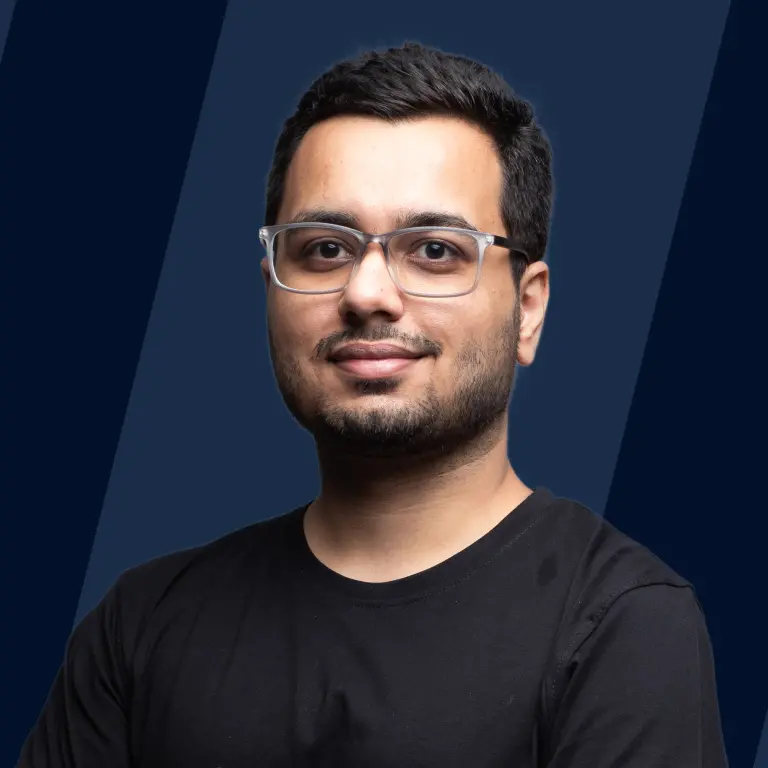
Overview
The floor() is a library function in C defined in the <math.h> header file. This function returns the nearest integer value, which is less than or equal to the floating point number (float or double) passed to it as an argument.
For example, if we pass a floating point number 5.6677 to the floor() function, the return value will be 5 (an integer).
Syntax of floor() in C
Syntax of the floor() function in C:
A floating point number (a float or a double value) is passed to the floor() function.
Parameters of floor() in C
The floor() function in C has a single parameter: a floating point number. A floating point number is a number with a decimal place, i.e., float and double in C.
Return Values of floor() in C
The floor() return value in C is an integer value immediately smaller or equal to the floating point number (float or double value) passed to it.
Example of floor() in C
Code
Output
In the above example, we are rounding off num to its immediately smaller integer using the floor() function. We are using the <math.h> header file as the floor() function is defined in it.
floor() Function in C
The floor() function in C is used to convert a floating point number to its immediately smaller integer (for eg, 3.6 to 3). This function is a part of <math.h> header file. The floor() function only accepts floating point numbers and returns an integer.
More Examples
Let's have a look at some more examples of the floor() function to have a clearer understanding:
Using a float value as the input
Code
Output
In the above example, we are rounding off 9.999 to its immediately smaller integer using the floor() function. We get 9 as the result. We are using the <math.h> header file as the floor() function is defined in it.
Using a Double Value as the Input
Code
Output
In the above example, we are rounding off the double value 5.7642753290763686574675442 to its immediately smaller integer using the floor() function. As a result, we get 5 in the output.
Integer as Input
Code:
Output:
In the above example, we are rounding off 3 to its immediately smaller integer using the floor() function. As 3 is already an integer, the floor() function returns the input as it is.
Scientific Notation (float) as Input
Scientific Notation is the way of expressing a number that is too large or small to represent in decimal form.
For example: 0.00000000043 in scientific notation is . This notation is mostly used for floating point numbers (float or a double value)
Code
Output
In the above example, we are rounding off the scientific notation to its immediately smaller integer using the floor() function. As a result, we get 6376 in the output.
Finding the fractional part of a number using the floor() function
The fractional part of a number (float or double) is the digits beyond the decimal point of the number. It is equal to the difference between the original number and the greatest integer of that number.
In Mathematics, the fractional part of is denoted by , and its greatest integer (floor value) is denoted by . Therefore, .
Code
Output
In the above example, we find the fractional part of the number 6.376 using the floor() function, as a fractional part of a number equals the difference between that number and its floor value. Here, the fractional part of 6.376 is equal to 6.376 - 6, i.e., 0.376.
Conclusion
- The floor() function is defined in the <math.h> header file.
- This function rounds off the floating point numbers (float and double values) into their immediately smaller or equal integer.
- We can also use integers with the floor() function, but it will return the input as it is.