Java For Loop
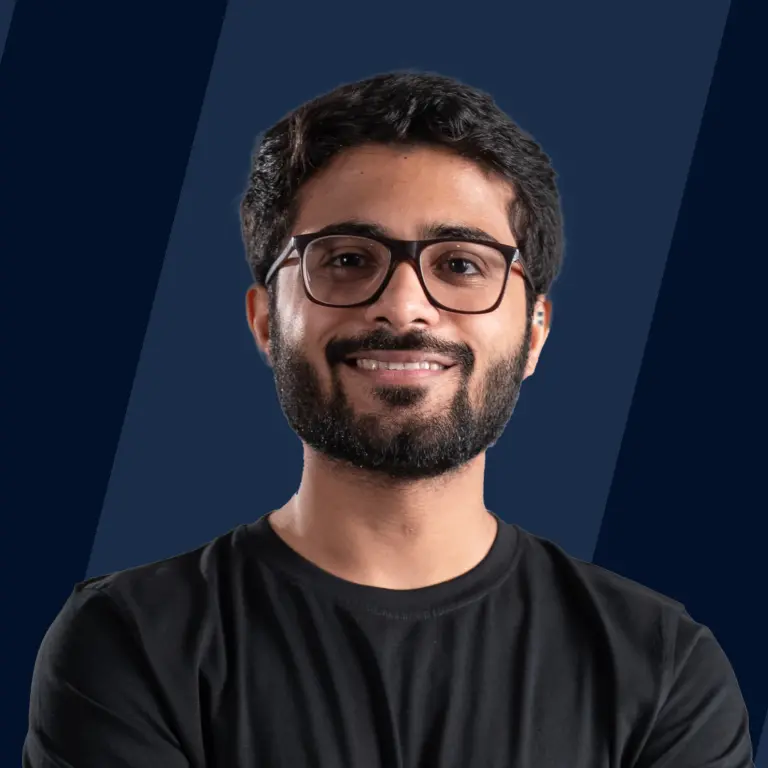
Java for loop is used to run a block of code multiple times. There are many types of for loop in Java:
- Simple For loop
- Nested For loop
- for each loop
- labeled for loop
- Infinitive for Loop
Using a for loop, which is a repetition control structure, you can quickly create a loop that has to run a certain number of times. To learn more about the loop in Java.
Java Simple for Loop
The for loop in Java is a control flow statement that allows you to execute a block of code repeatedly for a fixed number of times, or over a range of values. It consists of three parts:
1.Initialization Expression(s)
The loop in Java starts with an initial condition, and this is executed only once when the loop starts.
2.Test Expression It is the second condition that is executed every time to check/test the condition of the loop.
3.Update Expression(s)
The update expression(s) modifies the loop variables' values. At the conclusion of the loop, after the body of the loop has been completed, the update expression is used. For instance, increment or decrement commands can be used as update expressions.
Syntax
Example
In this example, we are going to use simple for loop to print numbers from 1-5
Output
Java Nested for Loop
A nested for loop in Java is when it contains another loop within its body. The inner loop, however, must come to an end before the outer loop in a nested loop. An illustration of a "nested" for loop in java is the following:
Syntax
Example
In this example, we will print the * pattern using nested for loop.
Output
Code Explanation
In Above code first for i=1 inner loop will run 1 time and print single *.Then for i=2 inner loop will run 2 time and print * * for i=3 * * * and so on.
Java for-each Loop
In Java 5, the for-each loop was first introduced. It's also known as an Enhanced for a loop.
It's a different traversing method created specifically to go across collections or arrays. Notably, the for is also used as a keyword. However, we assign a variable with the same type as an array or collection in place of utilizing a loop counter variable.
For-each refers to the process of iteratively traversing each element of an array or collection.
Example
In this example, we are going to print each element of array num using for each loop.
Output
Code Explanation
In the Above Code, the loop will traverse through an array num, and the number will store each of its elements in every iteration.
Java Labeled for Loop
When we wish to stop or continue a certain for loop in response to a need, labeling a for loop is helpful. A break statement inside an inner for loop will cause the compiler to exit the inner loop and restart the outer loop.
It can be used with break and continue. Labels allow you to shift control from the break and continue. In general, a break goes outside a loop, but if you have more than one loop, you can specify which loop you want to break by providing labels in the break statement.
Syntax
Example
In the example below, we are going to use the label for loop with nested for loop in Java.
Output
Code Explanation In the above code, the innermost loop prints 5 when j2=5, and when j2=6 breaks, the loop exits with the outermost label.
Java Infinitive for Loop
If a condition is always true, an infinite loop will result.
Example
In this example, we will use an infinite loop to print "Hello World" endlessly.
Code Explanation
This will print Hello World endlessly. Because the termination Condition is not specified
Code Explanation
The code above will start printing numbers at zero and never stop because a termination condition is not given.
Java for Loop vs while Loop vs do-while Loop
Aspect | for loop | while loop | do-while loop |
---|---|---|---|
Initialization | Initialization is done within the loop declaration. | Initialization is done outside the loop. | Initialization is done outside the loop. |
Condition Checking | Condition is checked before each iteration. | Condition is checked before entering the loop. | Condition is checked after the first iteration. |
Control Variable Update | Control variable update is done within the loop. | Control variable update is done manually within the loop. | Control variable update is done within the loop. |
Execution Control | Executes a block of code a fixed number of times. | Runs a block of code as long as the condition holds true. | Executes a block of code at least once, then repeats. |
Usability | Preferred when the number of iterations is known. | Useful when the condition depends on an external factor. | Useful when you need to ensure at least one execution. |
Readability | Can be less readable for complex conditions. | Straightforward, especially for simple conditions. | Clear control flow, especially for mandatory execution. |
Conclusion
- Using a for loop in Java, which is a repetition control structure, you can quickly create a loop that has to run a certain number of times.
- for loop in Java are of different types
- Simple For loop
- Nested For loop
- for each loop
- labeled for loop
- Java Infinitive for Loop
- The for loop is ideal for situations where the number of iterations is known
- while loop continues executing as long as the condition remains true.
- The do-while loop ensures that the loop body executes at least once before checking the termination condition.