JavaScript Form Validation
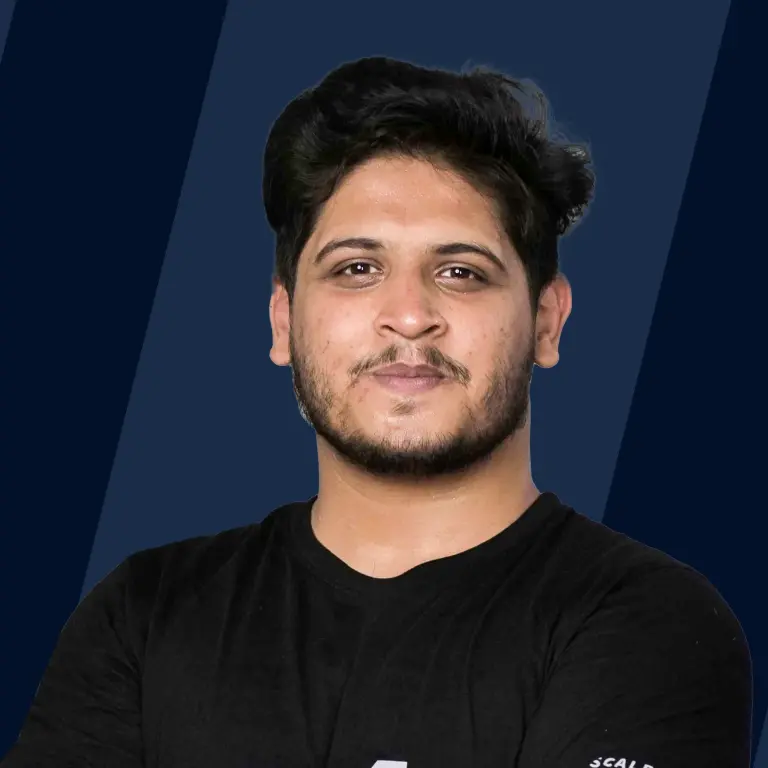
Ensuring the integrity of user-submitted data is imperative, prompting the need for form validation. JavaScript's client-side validation is favored by developers for its efficiency, allowing faster data processing than server-side methods. This versatile language validates diverse fields such as names, passwords, emails, and more. Forms, integral to webpages, gather user details for server processing, finding application in e-commerce, online banking, and surveys. Prerequisites involve HTML for form creation, CSS for layout design, and JavaScript for validation.
Building the Form Validation Script
Scripts are small pieces of code written in Javascript either in a script tag or in a separate file for some short automated process. With the Javascript form validation script, we can validate several input fields to ensure that user data is authentic. The script specifies when a request is to be sent to the server, obviously after the validations of user data.
JavaScript Form Validation Examples
In this example, we will check for several form input fields like,
- Name should neither be null or blank nor consists of less than 3 characters.
- Password should neither be null or blank nor consists of less than 6 characters. Also, both passwords must match.
- Image must be of gif, png, or jpeg extension.
- Phone Number must contain all numeric values.
- Email must be correct(Further explanation of provided regex is given below).
JavaScript Name Validation
This javascript function will accept a name variable and will check whether it is null or blank, subsequently, it will check for the length of the name, which should not be less than 3.
JavaScript Re-Type Password Validation
This javascript function will compare the two provided passwords.
JavaScript Number Validation
This function will check whether the given input is a number or not. In javascript, we have an inbuilt function isNaN() which returns true if the provided argument is a number.
JavaScript Validation for Image
This function will accept the path of the image file, which is provided by the value attribute of HTML. In this function, we are splitting the path at the \ character, and the pop function is returning the last element of the array after the split. In this way, we are somehow getting the file name. Later we have a Regex pattern which will be used to check whether there is .jpg, .jpeg, or .png in the file name, exec() method is being used for the same purpose.
Example:
If we combine all the functions described above with our HTML form, it will look something like this.
Note : We are not showing the CSS file just to avoid the length of code because this article is not concerned with styling.
Explanation:
- We are performing the execution of validateForm() function with the submit button of the form.
- Inside the validateForm() we are accessing the node of the document by their name attribute to ultimately fetch their value.
- Example : document.signup.fname.value, It will look for the signup named node which is a form, and then it will look for the fname named child which is an input element and finally it will access the value.
- We are maintaining a boolean variable namedisValid according to return values of validation functions.
- At the end, if our isValid variable contains false then the form is not valid to be submitted.
JavaScript Email Validation
This function will accept an email string as a parameter and test it against a regex pattern to ensure that provided string is a valid email. Below is the description of RegExp Pattern,
- ^ denotes the starting of the string.
- [a-zA-Z0-9_.] denotes that characters from a-z, A-Z, 0-9, and _ are allowed.
- + denotes that the previous pattern could occur one or several times.
- @ will check for an exact match.
- [a-zA-Z0-9.] again it will check for a-z, A-Z, 0-9, and ..
- + denotes that the previous pattern could occur one or several times and subsequent $ denotes the end of the Pattern.
Understanding Client-Side Validation
Client-side validation protects our server against inappropriate data before a request is sent to the server.
This initial validation check provides a better user experience by avoiding the lengthy process of validation at the server only.
We can validate the data entered by the user, like ensuring the input format is correct, the mandatory fields are filled in, etc. By implementing client-side validation, we can determine when a request should be sent to the server.
Creating the HTML Form
We can create an HTML form by using the HTML tags related to forms, like, form, input, etc.
This is what a basic form looks like in javascript.
Let's try to transform this into a dummy sign-up form.
Output:
When we open this HTML file in our browser we would be able to see this form as output,
Adding Style Sheet to Beautify the Form
CSS File:
Output:
Our styled form will look something like this,
Conclusion
- Client-side validations are performed in order to protect our server from invalid data.
- A server cannot respond correctly if the incoming data is not well-formatted; it is, therefore, necessary to validate the data.
- In Javascript, we can validate a form by writing a script and then attaching the script to the submit button's on-click event.
- If the script can determine the validity of the data, then only the server requests will be executed.