Format in Python
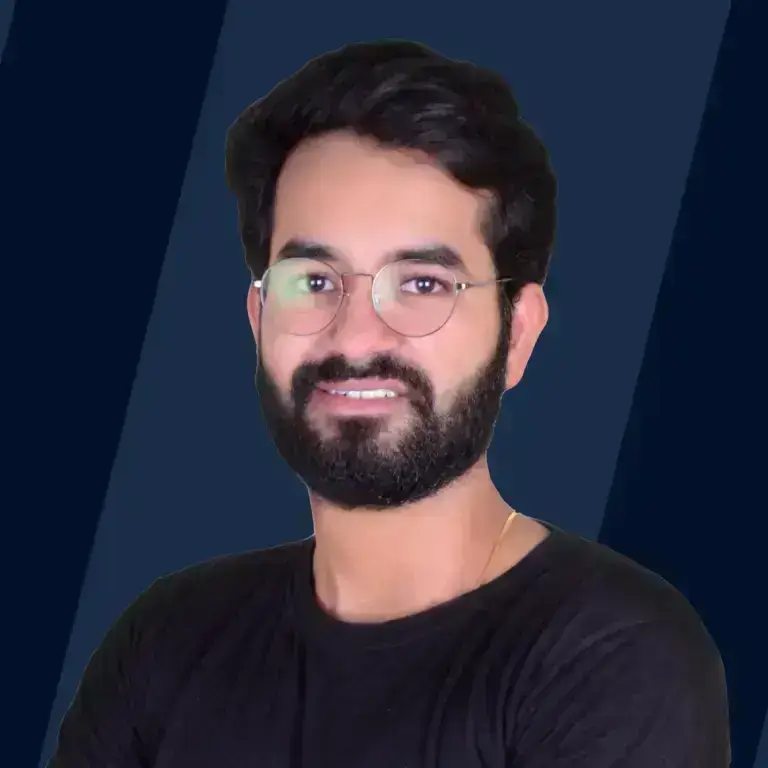
Overview
Format is the arrangement and representation of something. In Python, we use the format() functions to format our data.
There are two types of format functions in Python, one is the format() function which is used to convert a value to a formatted representation, and the other is the str.format() method used to insert variables in a string without having to concatenate different strings.
Introduction to format() function in Python
Let us assume, for instance, that you are given some numbers in decimal format and have to convert them into binary, octal, and hexadecimal numbers. Though there are many ways to convert decimal numbers into these formats, the format() function comes in handy here.
The format() is a built-in function in Python that converts a value into the required format/representation (shown in the example below). Code:
Output:
In the above example, we have used the format() function to convert a decimal value into a binary value.
Now we will discuss the syntax and parameters of the format() function.
Syntax of format() in Python
The format() function has two parameters (value and format_spec).
The syntax of the format() function is:
Read the next section to know more about these parameters in detail.
Parameters of format() in Python
The format() function has two parameters:
- value: the value which has to be formatted (value can be a number or a string).
- format_spec (optional): This is a string for inputting the specification of the formatting requirements.
The second parameter (format_spec) is optional; in its absence, the value will be formatted into a string by default.
Format of format specifier (format_spec)
The second parameter of the format() function called format_spec follows a certain format.
At first glance, this may look very intimidating, but this is not as hard as it looks.
Here's an overview of what the various specifiers mean:
-
fill: (any character)
It is a character that fills up the empty spaces after formatting. It is also called a padding character.
-
align: It is an option to specify the alignment of the output string.
"<": left-alignment specifier
">": right-alignment specifier
"^": center-alignment specifier
"=": justified specifier -
sign: This determines the use of signs for the output.
"+": Positive numbers have a "+" sign, and negative numbers have a "-" sign."-": Negative numbers have a "-" sign.
" " (space): Positive numbers are preceded by a space, and negative numbers are preceded by a "-" sign.
-
"#": This specifies that the return value should have a type indication for numbers. For example, hexadecimal numbers should have a "0x" prefix added to them.
-
"0": This specifies that the output should be sign aware and padded with 0's for consistent output.
-
width: Specifies the full width of the return value.
-
",": Specifies that the return value should have commas as a thousand separator.
-
.precision: Determines the number of characters after the decimal point.
-
type: This Specifies the output type. The types are three types:
- String: Use an "s" or nothing at all to specify a string.
- Integer: d (decimal), b (binary), o (octal), x (hexadecimal with lowercase characters), X (hexadecimal with uppercase characters), c (character)
- Floating point: f (lowercase fixed point), F (uppercase fixed point), e (exponent using "e" as separator), E (exponent using "E" as separator), g (lowercase general format), G (uppercase general format), % (percentage)
This format is discussed in a detailed manner in the examples section below ("Example 1: Number formatting with format()" and "Example 2: Number formatting with fill, align, sign, width, precision, and type").
Return type of format()
The format() function returns a formatted representation of the given value as per the format specifier. The return value is always a string, irrespective of the input.
Example 1: Number Formatting with format()
Code:
Output:
In the above example, The decimal value is being converted into binary, octal, and hexadecimal values using type specifiers (discussed in the section above - "format of format specifier")
Example 2: Number formatting with fill, align, sign, width, precision, and type
Example: Formatting an Integer Code:
Output:
*+4,453
In the above example, we formatted the integer "4453"; the format_spec is *>+7,d. Let's understand the significance of each symbol in *>+7,d:
- * - The fill character fills up the empty spaces after formatting.
- > - The right align option aligns the output string to the right.
- + - It is a sign option forcing the number to have a sign on its left.
- 7 - It is the width option forcing the number to have a minimum width of 7; empty spaces get filled by fill character.
- , - It is the thousands operator, which puts a comma between all thousands.
- d - It is the type specifier option specifying the number into an integer.
Example: Formatting a floating-point number Code:
Output:
0933.3630
In the above example, we are formatting the floating-point number "933.3629", the format_spec is ^-09.3f. Let's understand the significance of each symbol in ^-09.3f:
- ^ - It is the center align option aligning the output string to the center.
- - - It is a sign option forcing only negative numbers to have a sign.
- 0 - It is a character placed in empty spaces.
- 9 - It is the width option forcing the number to have a minimum width of 9.
- .3 - It is the precision operator specifying the precision of a given decimal number to 3 places.
- f - The type specifier option specifies the number into a floating-point number.
Example 3: Using format() by Overriding __format__()
Code:
Output:
In this example, we have overridden the __format__() method of the class Car.
It now accepts a format parameter and returns Red if it is equal to colour, else None is returned.
The format() function internally runs Car().__format__("colour") to return Red.
Introduction to String format() Method in Python
There will be multiple instances when we have to insert values in a string, str.format() method is used for this.
Python's str.format() method allows us to format the specified values and insert them in place of placeholders into the string.
The placeholders in the str.format() method are defined using curly brackets "{}". Read more about placeholders in the "Formatters using Positional and Keyword arguments" section below.
Here's an example showing the application of the string format method: Code:
Output:
In the above example, we have used the string format method to insert a value inside a string.
This was just for demonstration; read along to know how this works.
Syntax of String format() method in Python
The str.format() method is required to have one or more than one parameter (values to be formatted and inserted into the string).
Syntax of str.format() method is:
Read the next section to learn about its parameters.
Parameters of String format() method in Python
The str.format() method can have one or more than one parameters, all of which are values that have to be formatted and inserted in the input string.
The values can be of any data type. Parameters can be a list of values separated by commas, a key-value list, or a combination of both.
There are two types of use cases for formatting strings; one is when we have to format/insert only one value into the string, which is called a Single formatter, the other case is when we have to deal with multiple values, called Multiple formatter.
Single Formatter
This means we can pass only one value into the str.format() method, which after getting formatted, takes the placeholder's position inside the string.
Since we are passing only one parameter to the string format method, it is called a single formatter.
Let's see how single formatters work:
Code:
Output:
In the above example, we have inserted a single value into the string using the str.format() method where "Everyone likes {}" was the string and "pizza" was the value to be inserted in it in place of the placeholder.
Multiple Formatter
In this case, the string format() method will have more than one parameter.
Multiple formatters are used when we want to insert more than one value in a string. The values substitute the placeholders in order (from left to right). We can use positional arguments to change this order, which we will learn about in the next section.
Here's an example for Multiple formatters:
Code:
Output:
In the above example, we have inserted multiple values into the string using the str.format() method where "People who like {} also like {} with {}" was the string and "pizza", "pasta", and "garlic bread" are values to be inserted in it in place of the placeholder. The three values are inserted into the string in the same order they were passed into the function.
The number of placeholders and values in the format() should be equal. Otherwise, there will be an error.
:::
Formatters Using Positional and Keyword Arguments
The values in the str.format() method are primarily tuple (tuples are a sequence of immutable Python objects) data types. Every value in the tuple is referred to by its indexes starting from 0. These index numbers are then passed into the placeholders, and then they get substituted with the values.
Positional arguments are arguments that refer to the values at a specific index number while calling a function. They can be used to get a particular value in place of the placeholder while formatting strings.
Explained with an example below:
Code:
Output:
In the above example, we are using index numbers in placeholders to refer to the values inside the str.format() method and insert them in the string.
The order of the values inside the sentence can also be changed using indexes.
Explained with an example below:
Code:
Output:
In the above example, we are using index numbers in placeholders to refer to the values inside the str.format() method to change the order of the values being inserted into the string.
Keyword arguments are arguments that can be called by their keys, and by referring to the key, the argument's value can be accessed.
In the String format method, keyword arguments can be used to access the value by putting the key inside the placeholders.
Here is an example of Keyword arguments in the str.format() method:
Code:
Output:
In the above example, we are using keys in placeholders to refer to the keyword arguments in order to access their values and insert them into the string.
Both Keyword arguments and Positional arguments can also be used together.
In this example, we are using both positional and keyword arguments for passing values into the string. The key "food1" passes the value "pizza" into the string in place of the first placeholder, whereas the index number "0" in the second placeholder refers to the first positional argument, which passes the value "pasta" into the string in place of the second placeholder.
Type Specification in format()
Some parameters apart from the index or key can also be enclosed among the curly brackets (placeholders) by using format code syntax. These parameters are used for converting data type, spacing, or aligning, and here we are going to discuss how to convert the data type of values in string format().
Some important conversion types are:
Converting decimal into binary in string format: Code:
Output:
In the above example, we have converted the decimal number (22) into a binary number using a type specifier ("b").
Limiting the number of decimal points in a floating integer: Code:
Output:
In the above example, we are specifying the precision of the floating-point number (43.542130) using the .precision specifier (".3").
Spacing and Alignment Using Formatter
Spacing, aligning or even signs follow the same format of the format_spec discussed above in the section "format of the format specifier".
For alignment, the operators are:
Below are some examples for applying spaces and alignment using str.format(): Code:
Output:
In the above example, we are specifying the width of the first formatted value to be "10", due to which we can see that the string "Delhi" is aligned left to the spaces in the output.
Code:
Output:
In the above example, we are specifying the width of the second formatted value to be "10", due to which we can see that the integer "450" is aligned right to the spaces.
As you can see, the strings are left-justified, and the numbers are right-justified, let's see how to change this behavior using alignment options. Code:
Output:
In the above example, we are specifying the width of the first formatted value to be "10" and left-aligning it using the "<" operator, due to which we can see that the string "Delhi" is aligned left to the spaces.
Code:
Output:
In the above example, we are specifying the width of the second formatted value to be "10" and right-aligning it using the ">" operator, due to which we can see that the string "Delhi" is aligned right to the spaces.
Organizing Data
When handling and displaying data, organizing the data for easy reading, accessing, and management is always preferable. Formatters can help organize the data using align, sign, and width specifiers.
Let's look at a set of unorganized data: Code:
Output:
In the above example, we displayed a data set without organizing it. It's not readable, and numbers are overflowing into each other's columns, making it very hard to distinguish between them.
Now, let's use formatters to organize the same set of data: Code:
Output
In the above example, we have displayed a set of data using align and width specifiers. The alignment is left-align, as seen in the placeholders, this aligns all the sets of columns to the left, and the width is "5", making each column of width "5" to store big values in an organized way.
This is how formatters make data more readable, manageable, accessible, and organized.
Built-in format() vs String format()
The built-in format() function is a low-level implementation for formatting an object using __format()__ internally, whereas str.format() is a high-level implementation of the same, able to perform complex formatting operations on multiple values and insert them into the string.
The format() is a function, and str.format() is a method.
Conclusion
- The format() function is used for formatting representations of a value.
- The values are formatted using a set of operations in a defined order.
- The string format() method is used for passing multiple values and their formatting in a string.
- The format() functions can be used to convert data types, change their representation and help in organizing them.
- Both format() function and string format() method call the __format__() method internally.