getOrDefault() in Java
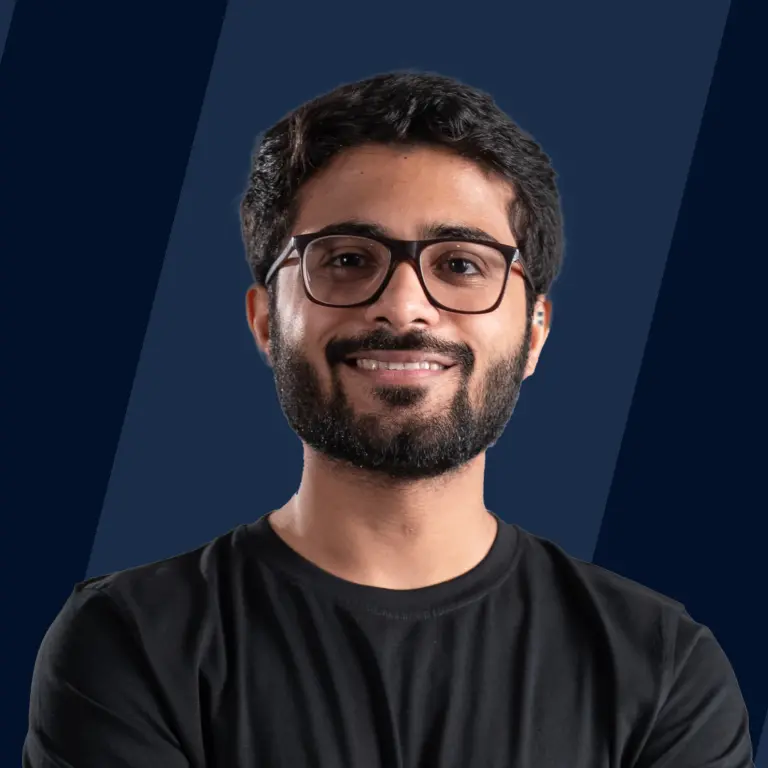
Overview
getOrDefault() in Java is a function or a method that comes with the HashMap class and is used to find the value associated or mapped to the specified key in HashMap. The syntax of getOrDefault() in Java: map.getOrDefault(Object key, Default value) Here, map is a variable object of the HashMap class, and getOrDefault() method is used to find the value associated with the corresponding key. It will return the default value if there is no mapping found for the specified key in the HashMap.
Syntax of getOrDefault() in Java
The syntax used for getOrDefault() method in Java is:
Here, map is a variable object of HashMap class.
Parameters of getOrDefault() in Java
The getOrDefault() method in Java takes in two parameters:
- Map Object Key -> It refers to the key element whose corresponding mapped value needs to be returned.
- Default Value -> It refers to the default value which is returned when there is no mapping or an associated mapped value found for a specified key in the HashMap.
Returned Value of getOrDefault() in Java
- getOrDefault() method in Java returns the value associated with the specified key in the HashMap.
- If the key doesn't exist or there is no mapping of the specified key in the HashMap, the getOrDefault() method returns the specified default value.
get() vs getOrDefault() in Java
In HashMap, get(Object key) method is used to retrieve the value of the specified object key. Example:
Output:
Here, we have a map as an object of HashMap<String,Integer> class and we have stored two key-value pairs in the map. We can easily fetch the values associated with the keys in HashMap using get(Object key) method. But have you ever wondered what if there is no mapping of the specified key in the HashMap? We will get the null value which is obviously not a good practice for writing code in Java. Instead of returning the null value, there should be some default value.
In the above example, we are avoiding the null values in the result by replacing them with the default value 0. But for this, we have to always put an additional null check for each of the values that we want to retrieve from the HashMap. So now, the question arises do we have any other alternative way to set the default value for non-existing keys in the HashMap? The answer is yes, and we have a Java method for this purpose.
We can use getOrDefault() in Java which comes with the HashMap class and is used to find the value of a key associated with the HashMap. It returns the default value (passed as a parameter in the method) if no mapping is found for the specified key in the HashMap.
Now, we will study more about getOrDefault() method in Java using example codes as we move through the course of this article :)
Modified above used Example using getOrDefault()
Output:
As we can see, in this example there is no need to put additional null checks for all the values of the map, and we are getting the same desired result.
Examples to illustrate how to use getOrDefault() in Java
Example 1.
In this Java example code, we have made a HashMap object named m1 (Key: Integer, Value: String). We then insert some key-value pairs into the HashMap. After printing the map, we then use getOrDefault() in Java to access some values based on their mappings with the specified keys. Clearly, value1 will be initialized with the value "C++" which is mapped with key 1 and since, the map doesn't contain any mapping for key 5, value2 will be initialized with the default value - "Not found!". We will then print the values at the end.
Output:
m1.getOrDefault(5,"Not Found!"); Here, 5 is the key whose mapped value is to be returned, and "Not Found!" is the default valued string that will be returned when we don't have any mapping of key 5 in the HashMap.
Example 2.
In this Java example code, we will be storing English Alphabets in the HashMap in order of their occurrence. We have made a HashMap object named m1 (Key: Character,Value: Integer). We will then insert the character-integer value pairs of English Alphabets into the m1 HashMap. For Example: 'A' will be mapped to 1, 'B' will be mapped to 2, and so on. After printing the HashMap, we will then use getOrDefault() in Java in order to target some key-value pairs to print their mapped values. Clearly, value1 will be initialized with 13, which is the mapped value of key - 'M', and value2 will be initialized with 404, which is the default value for key - '@' returned. We will print these values at the end.
Output:
m1.getOrDefault('@',404); Here, '@' is the key whose mapped value is to be returned, and 404 is the default value that will be returned when we don't have any mapping of key '@' in the HashMap.
Example 3.
In this Java example code, we are doing something unique and out-of-the-box. Extending the use-case of Example 2, we have HashMap m1, and all English Alphabets are stored in it according to their occurrence. Now, user will be prompted to enter the string str, and we will try to sum all the mapped values of all the characters of the string. getOrDefault() method will be used, which will return the mapped value of the specified key, and the default value will be set to -1. This indicates that if the string contains any character out of {"A-Z"}, then it will decrement the sum by 1. After doing this, the resultant sum value will be printed at the end.
Output:
Here assuming the entered string is "SCALER" and according to the reference with the HashMap (m1), we have {S:19, C:3, A:1, L:12, E:5, R:18} Adding all these values to the sum, we get the result 58.
Here assuming the entered string is "ABC#" and according to the reference with the HashMap (m1), we have {A:1, B:2, C:3, #:-1} Character '#' doesn't exist in the HashMap and, thus, will have its default value -1. Adding all these values to the sum, we get the result 5.
Conclusion
- getOrDefault in Java is a function or a method that comes with the HashMap class and is used to find the value mapped to the specified key in HashMap.
- The syntax used for getOrDefault() in Java: map.getOrDefault(Object key, Default value) Here, map is a variable object of the HashMap class.
- getOrDefault in Java takes in two parameters: Object Key, whose mapped value needs to be returned, and Default Value, which needs to be returned in case the key doesn't exist in the HashMap.