gets() Function in C
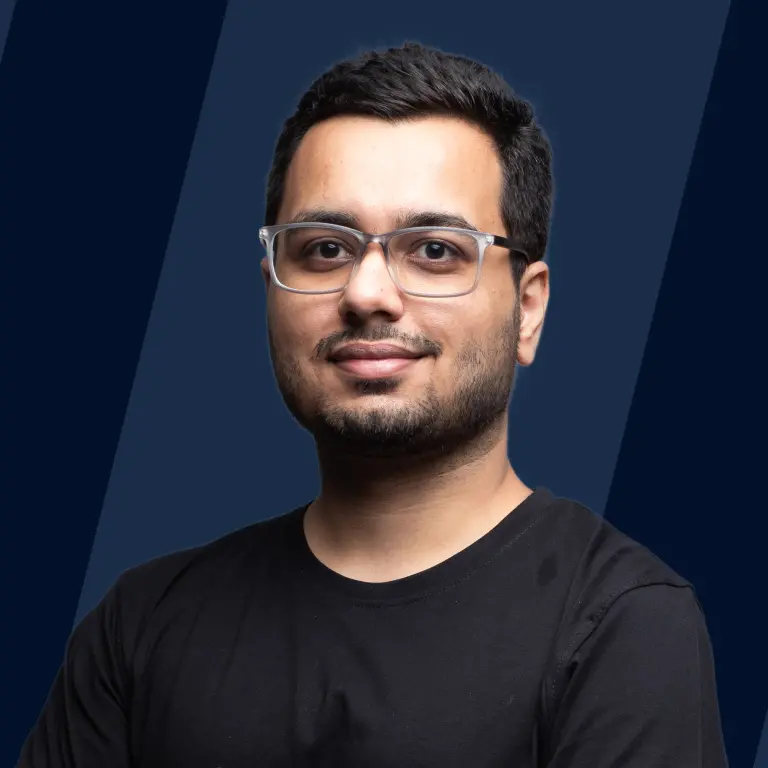
Overview
The gets() function is used to read characters from stdin and store them into the provided character array until a new line or EOF, end of the file is reached. It is declared in <stdio.h> header file.
Syntax of gets() Function in C
The syntax of gets function in C is as follows:
Parameters of gets() Function in C
Parameter: Pointer of char data type.
It accepts a pointer to the array of characters and after the read operation, the data is stored in that array.
Return value of gets() Function in C
Return Value: Pointer of char data type.
- Success: It returns the pointer to the same string/(array of characters ) where data was stored.
- Failure: Null Pointer.
Example
Input:
Enter a String: Hello World!
Output:
In the above code, we declare a character array str then take input for that using gets(). In the end, we print the value of str.
What is gets() Function in C?
The gets function in C reads characters from the stdin until a new line or end of the file is reached. It accepts a pointer to the memory where it stores those array of characters. A terminating null character(\0) is automatically appended after the successful writing of characters to the string.
This function was removed in C 11 due to its buffer overflow problem. While using the gets function in C, compiler shows warnings like this,
- warning: ‘gets’ is deprecated
- warning: the 'gets' function is dangerous and should not be used
Limitation of gets() Function in C
The function does not prevent buffer overflow at destination memory, which means the function does not have a limit check to prevent reading after the characters exceed the size of the array passed to the function. The program will terminate with a runtime error in such a scenario.
A function with the bound check was introduced in C 11 that has been named gets_s(). It is similar to gets but it accepts a size/limit and reads the characters until the size is not exceeded. By providing the limit, it fixes the problem of buffer overflows associated with the gets function. Below is the syntax of gets_s() function.
Difference between scanf() and gets() Function
scanf() | gets() |
---|---|
scanf() function takes input from stdin according to the format specifiers. By default, it considers whitespace, newline, and tab as delimiters. | gets() function takes input from stdin until a new line (\n) character or EOF is reached. |
It doesn't consider whitespace as input, although we can use a custom [^\n] delimiter to read the entire line. | It reads the entire line of string by default. |
int scanf(const char *format, Object *arg(s)); | char* gets ( char* str ); |
It can accept the input in any data type according to the format specifier. | It accepts only the string data type. |
More Examples
Buffer Overflow Example
Input:
Enter a String: This string is going to exceed the limit of destination array.
Output:
As we can see the program throws a runtime error as the size of input string exceeds the size of the character array causing a buffer overflow.
Conclusion
- We use the gets function in C to take the input of the entire line into a string.
- gets() take a pointer to the character array and store the input string into it. After this operation, it returns the same pointer.
- This function was removed in C 11 because of some limitations.
- The alternative to gets function was introduced as gets_s function which accepts a limit to prevent buffer overflow.
- The most suitable alternative to gets function is fgets function.
See Also
- scanf()
- fgets()
- gets_s()