Global Variable in Python
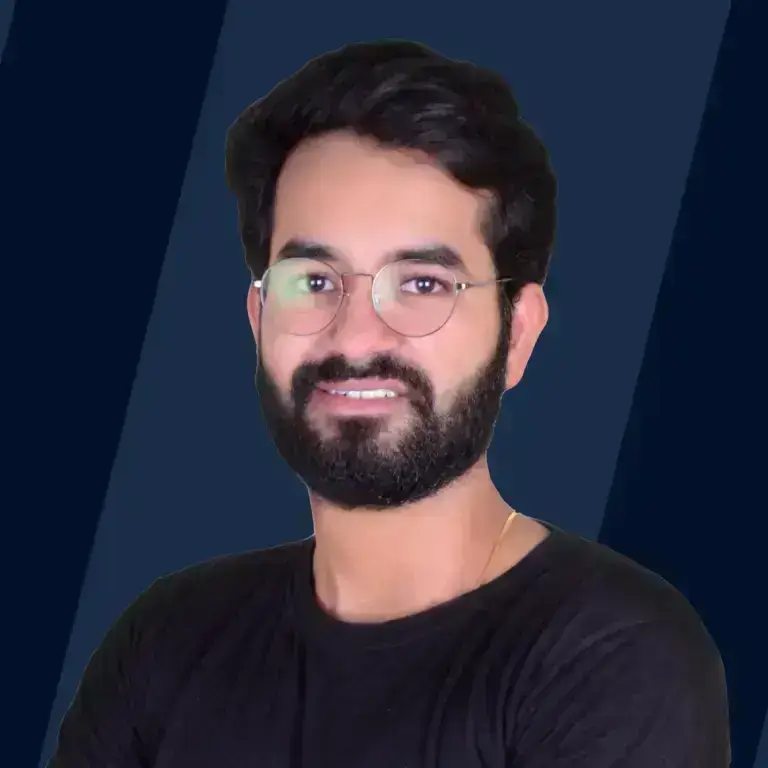
What is a Global Variable in Python?
A global variable in Python is a variable declared outside of the function or in the global scope. A global variable can be accessed from both inside and outside the function. A variable that you want to use throughout the scope of a program should be declared globally.
Create a Global Variable in Python
To make a global variable in Python, you must declare it outside of the function or in the global scope.
Output:
How to Access the Global Variable Inside and Outside of a Function?
Let's see how we can access a global variable when it is used inside and outside a function:
Output:
Using Global Variables in Python Functions
The global Keyword
The global keyword is used to declare that a variable inside the function is referencing a global variable.
This allows you to modify the global variable from within the function's scope.
In this example, the check_global() function modifies the value of the global variable num_1 by using the global keyword.
The globals() Function
The globals() function in Python returns a dictionary representing the global symbol table. This dictionary includes all the global variables in the current global scope, including built-in variables.
Here's how you can use the globals() function:
When you call my_function(), it prints out a dictionary containing all the global variables available at that point in the program.
Local Variables
- Variables that are declared within a function's scope are called as local variables.
- These variables are only accessible within the function where they are defined.
In this example, number is a local variable defined within the local_function() function. Attempting to access number outside of the function will result in a NameError because it's not defined in the global scope:
Difference Between Global and Local Variables
Here's a table summarizing the differences between local and global variables in Python:
Aspect | Local Variables | Global Variables |
---|---|---|
Definition | Defined inside a function or block. | Defined outside of all functions. |
Scope | Accessible only within the function/block where they are declared. | Accessible throughout the entire program, including inside functions. |
Lifetime | Exist only during the execution of the function/block. They are destroyed once the function/block execution is completed. | Persist throughout the life of the program. |
Declaration | Declared within the function/block. No special keyword required. | Declared outside any function. If modification is needed inside a function, the global keyword must be used. |
This table highlights the fundamental distinctions between local and global variables in terms of their scope, lifetime, declaration practices, and their use within Python programming.
Now, we'll see how global and local variables behave in the same code, through an example:
Output:
In the above code, we declare the variable x as global and y as local inside the function. Next, we perform an operation on the variable x, and leave y as it is. Then we call the function and observe that the value of x comes out to be 10, as we multiplied x by 5. We also print the value of y.
Conclusion
- Global variables are those variables that can be accessed both inside and outside functions in a program.
- Global variables should be declared outside a function.
- Local variables are declared inside a function, and their scope is limited to that function only. This is what differentiates them from global variables.
- To declare a global variable inside a function, the word global should be used to initialize the variable. Example: global x, where x is the name of the variable.