HashCode() in Java
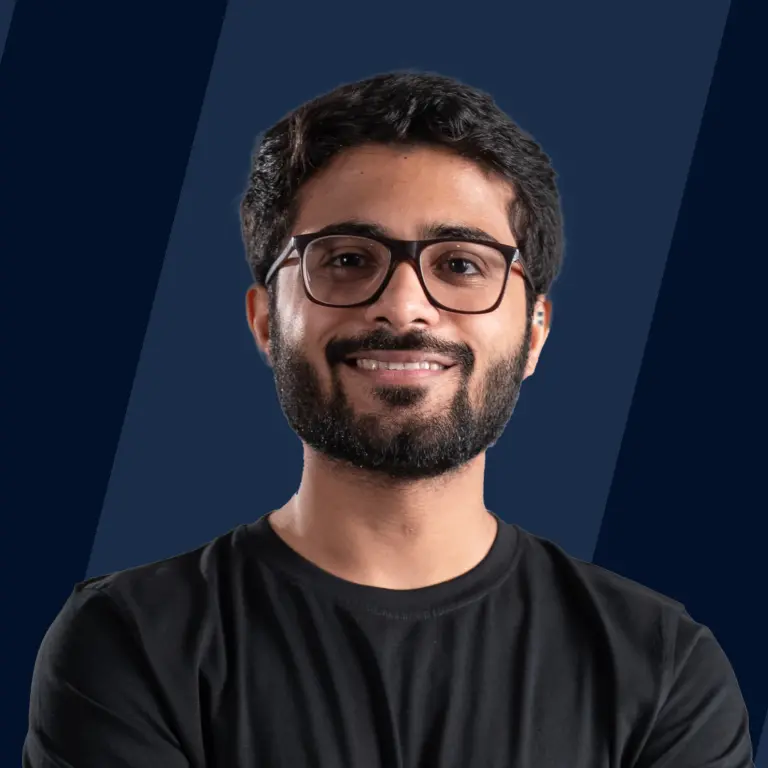
In Java, the hashCode() method determines the hash code for objects, pivotal for collections like HashMap and HashSet. The method has two variants: the general hashCode() for objects and hashCode(int value) specifically for integers. Proper implementation ensures efficient hashing for optimized collection performance.
The Java hashCode() Method
The hashCode() method is defined in the Java Object class. It computes the hash values of given input objects and returns an integer representing the hash value of the input object.
The hashCode() method is used to generate the hash values of objects. Using these hash values, these objects are stored in Java collections such as HashMap, HashSet and HashTable.
Example
Through this example, we will test the basic properties of Hashing mentioned in the previous section.
- Two objects with the same value have the same hashcodes.
- Objects with different values usually have different hashcodes.
- Hashcodes of the same object, must remain the same when computed more than once,.
Code:
Output:
Integer class hashCode Methods in Java
hashCode in Java are of two types based on their respective parameters-
- hashCode() Method—The hashCode() method belongs to the Java Integer class. It returns the hash value of a given Integer object and overrides the hashcode() method of the Object class.
- hashCode(int value) Method—This method clearly has a parameter and determines the hashcode of that parameter: value.
Declaration of hashCode in Java
The syntax of the two types of hashCode() methods in Java is as follows –
Parameters of the hashCode Method
As we can see, the first type of hashCode method does not have a parameter.
The second type of hashCode method has the following parameter-
Datatype | Parameter | Description |
---|---|---|
int | value | This “value” determines the hashcode. |
Return value of the hashCode Method in Java
The return value of the two types of hashCode method in Java are as follows-
Method | Return Value |
---|---|
hashCode() | Returns the hashcode of the calling Integer object. The hashcode is computed using the primitive int value represented by the Integer object. |
hashCode(int value) | This method returns the hashcode of its primitive int argument i.e. value. |
Implementation of hashCode in Java
Now that we have learned about the different types of hashCode() methods in Java and their syntax and parameters, let's implement them in an example.
Example of hashCode(int value)
Code:
Output:
Example of hashCode() method
Now, let’s take a look at how to compute the hashCode of Integer objects in Java.
Code:
Use of hashCode in Hashing
For our advanced learners, we can examine how hashCode in Java is employed in HashMaps to compute the hash value of the HashMap keys.
- HashMap is a part of the collections framework in Java.
- When we store a (key, value) in a HashMap, the key's hashcode is calculated. The hashcode value received is referred to as a bucket number.
- Keys with the same hash code are present in the same bucket. In the same bucket, multiple (key, value) pairs may be stored in the form of a linked list.
- However, keys with different hash codes reside in different buckets.
- When we access the value associated with a particular key, the hashcode of the key is computed again.
- This hashcode value refers to its bucket, and the corresponding value is retrieved from it.
Conclusion
- Hashing is a Computer Science concept that maps an object or entity to an integer.
- The hash value of an object is an integer value that is computed using the properties of that object.
- Every object in Java inherits the hashCode() and equals() method.
- Hash codes of two equal objects must be the same. However, the hash codes of two unequal objects need not be distinct.
- An efficient hashing algorithm generates distinct hashcodes for unequal objects.
- The hashCode method should consistently implement the equals() function.
- hashCode() and hashCode(int value) methods, defined in the Integer class, compute the hash codes of integer objects or values in Java.