How can We Call the Garbage Collector Explicitly?
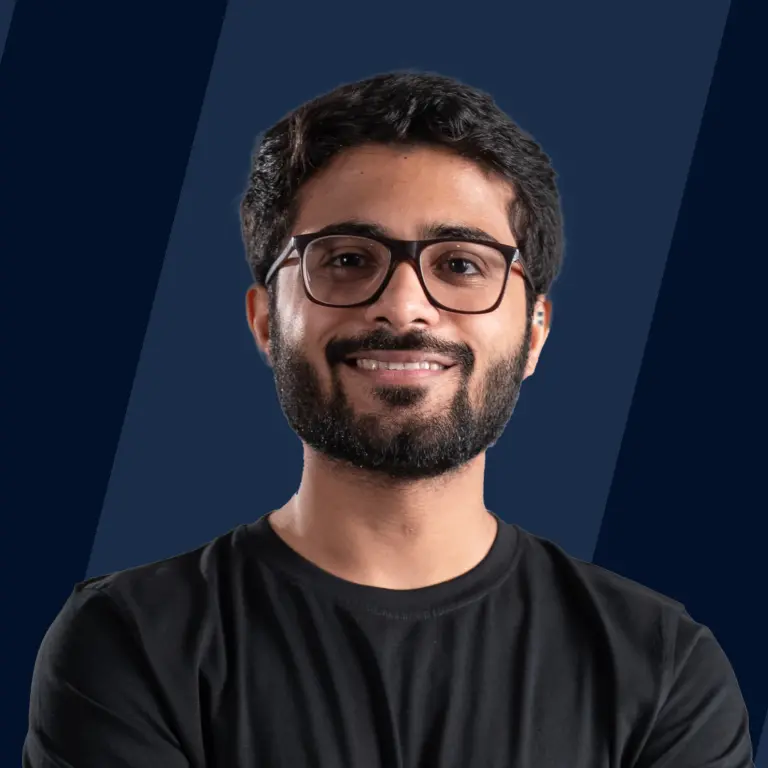
Objects that are no longer reachable, meaning that there are no references to them from any live threads or static fields are eligible for garbage collection. This is not necessarily related to how long they have been "idle" or "unused." The JVM's garbage collector runs automatically, but it does not run at specific intervals. It runs continuously and dynamically based on the available memory and the number of unreachable objects.
The next question that generally arises in a programmer's mind is:
Can we call the Garbage Collector Explicitly ?
The answer is yes, the garbage collector can be called explicitly. But the point here is that it does not run instantly every time; it's unpredictable whether the GC (garbage collector) will run immediately or not.
How can we call the Garbage Collector Explicitly ?
There are two ways to call the garbage collector explicitly and trigger the garbage collection process:
- Using the System Class method
- Using the Runtime Class method
Call the Garbage Collector using the System Class method
The System class is a core class in Java that belongs to the java.lang package. It is a final class and does not provide any public constructors, which makes all members and methods in the System class static.
There is a method named gc() in the System class that is used to call the Garbage Collector explicitly.
Syntax:
Code
Output(s)
Explanation
Here, we have created 100,000 different objects of the List class with Integer values in memory. These objects have not been used anywhere. We have printed the memory after creating these objects, which is 9828336. Then, we printed the memory after invoking the Garbage Collector using the gc() method of the System class. After the Garbage Collector gets triggered, it trashes out the unnecessary variables and objects from memory, due to which the updated and increased free memory printed is 124820368.
Note: The output of the code will be the memory usage before and after the garbage collector is invoked. The exact output will vary depending on the system's available memory and the current state of the JVM. However, as mentioned earlier, there are more reliable ways to measure the garbage collector than printing the memory before and after calling the garbage collector's performance. It is just an example to show the use of gc() method.
Call the Garbage Collector Using the Runtime Class Method
Every Java application has a single instance of the Runtime class, which is a Singleton class. The Runtime class allows the application to interface with the environment (JRE) in which it is running. The current runtime of a Java application can be extracted using the get runtime() method. An application cannot create its own individual instance of the Runtime class.
The Runtime class has a method named gc() that calls the Garbage Collector explicitly.
Syntax:
Code
Output(s)
Explanation
The program creates an instance of the Runtime class, which allows the program to access information about the runtime environment. Then, the program creates 100000 instances of the List class and adds some integers. Then it prints the memory usage before and after invoking the Garbage Collector using the gc() method of the Runtime class. The program is designed to show how the Garbage Collector can be used to free up the memory used by unneeded objects in a Java program.
Note: An important point to note is that there is no difference in the gc() methods of System Class and Runtime Class. Both are used to request the JVM to invoke the garbage collector to perform the garbage collection of the unreferenced objects. Still, we already agreed above that gc() is non-deterministic, as it cannot be predicted whether it will run immediately or not.
Learn More
To learn more about Garbage Collection in Java, please visit the following article link
Conclusion
- We can call the Garbage Collector in 2 ways:
- Using gc() method of the System class
- Using gc() method of the Runtime class
- All methods in the System class are static, so gc() must be called using the class name.
- Runtime is a Singleton class. So there will be just a single instance of Runtime for every Java application.
- There is no such difference between the GC () method of the System class and the GC () method of the Runtime class. However, comparatively, using the gc() method of the System class is easier, as it needs no object to access it.
- Garbage Collection in Java is unpredictable and non-deterministic.