How to Create a CSV File in Python?
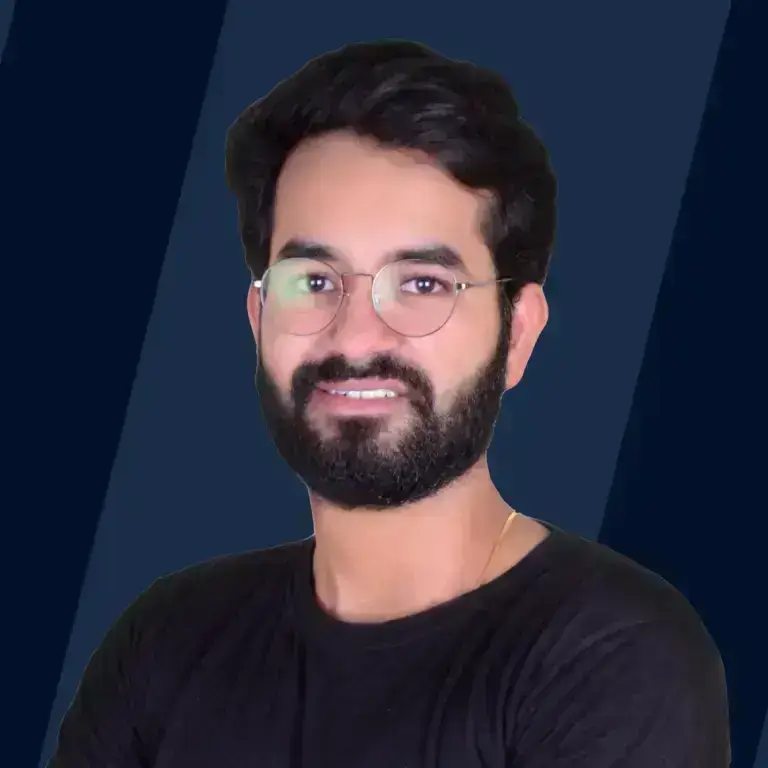
Overview
CSV stands for comma-separated values, which is a simple file format to store data in a structured layout. A CSV file stores and arranges tabular data such as a spreadsheet or database in the form of plain text. Each row in a CSV file is referred to as a data record. Each data record has one or more fields (columns) separated by commas. Therefore, the comma acts as a delimiter (character that identifies the beginning or the end of a data value) here.
How to Create CSV File in Python?
To create a CSV file and write data into it using Python, we have to follow a certain set of instructions:
- Open the CSV file in writing (w mode) with the help of open() function
- Create a CSV writer object by calling the writer() function of the csv module
- Write data to CSV file by calling either the writerow() or writerows() method of the CSV writer object
- Finally, close the CSV file
CSV Module Functions in Python
CSV module is used to read/ write CSV files in Python. To use the csv module in our program we have to first import using the following statement:
Before learning about different types of functions let us study in brief about dialects, Dialects are used to group multiple formatting patterns like delimiter, skipinitialspace, quoting, and escapechar into a single dialect name, thus removing redundancy when working with multiple files. We will learn more about dialects later in this article.
Different types of functions in the csv module are as follows:
- csv.field_size_limit - This function returns the current maximum field size allowed by the parser.
- csv.get_dialect - It returns the dialect associated with a name.
- csv.list_dialects - It returns the names of all registered dialects.
- csv.reader - This function reads the data from a CSV file.
- csv.register_dialect - It associates dialect with a name, and the name must be a string or a Unicode object.
- csv.writer - This function writes the data to a CSV file.
- csv.unregister_dialect - It deletes the dialect, which is associated with the name from the dialect registry. If a name is not a registered dialect name, then an error is raised.
- csv.QUOTE_ALL - It instructs the writer objects to quoting all fields.
- csv.QUOTE_MINIMAL - It instructs the writer to quote only those fields containing special characters such as quote char, delimiter, etc.
- csv.QUOTE_NONNUMERIC - It instructs the writer objects to quote all the non-numeric fields.
- csv.QUOTE_NONE - It instructs the writer object never to quote the fields.
How to Open a CSV File in Python?
There are two common methods of opening a CSV file, one is using the csv module and other is using the pandas library.
-
csv module
In the above program, we have imported the csv library and used the .reader() function to read the csv file. This with keyword allows us to both open and close the file without having to explicitly close it.
The open() method takes two arguments, the first is the name of the file, and the second is the mode argument. Here, we are using r for reading, however r is also the default mode.
Learn in-depth about file handling and various modes to open a file from this article.
-
pandas library
In the above program, we are importing the Pandas library, which is used to implement data manipulation and analysis. It contains the .read_csv() method we is used to read our students.csv file.
How to Close a CSV File in Python?
If we are working with the file using the with keyword, it allows us to both open and close the file without having to explicitly close it.
In case we have opened the file using the open(filename.csv) method, we have to explicitly close it using the .close() method. The .close() method is used to close the opened file. Once a file is closed, we cannot perform any operations on it.
Level up your programming game with our free Python certification course - the ultimate path to mastering Python!
Methods to Create CSV File in Python
Python's in-built csv module helps us to deal with CSV files. There are various classes provided by this module for writing to CSV:
- Using csv.writer class
- Using csv.DictWriter class
Method 1: Using csv.writer Class
The csv.writer class is used to insert data in CSV files. It returns a writer object which is responsible for converting the user’s data into a delimited string. A CSV file object should be opened with newline='' otherwise newline characters inside the quoted fields will not be interpreted correctly
Syntax for csv.writer Class
Parameters
- csvfile - file object having write() method
- dialect - It is an optional parameter that specifies the name of the dialect to be used
- fmtparams - Certain optional formatting parameters that are used to overwrite the parameters specified in the dialect
csv.writer class provides two methods for writing data in a CSV file, which include the following
-
writerow(): This method is used when we want to write only a single row at a time in our CSV file. writerow() can be used to write field rows. Syntax
Let us see the implementation of writerow using the following example:
Output
In the above example, a students.csv file will be created in the current working directory with the given entries. Here, we have opened the students.csv file in writing mode using the open() function, and then the csv.writer() function is used to create the writer object.
-
writerows(): This method is used to write multiple rows at a time. writerows() can be used to write a list of rows. Syntax
Let us see the implementation of writerows using the following example:
Output
In the above example, a students.csv file will be created in the current working directory with the given entries. Here, we have opened the students.csv file in writing mode using open() function, and then the csv.writer() function is used to create the writer object. Then, the 2D list is passed to the writer.writerows() function to write the data of the list to the CSV file. Use this Online Python Compiler to compile your code.
Method 2: Using csv.DictWriter Class
The objects created using the csv.DictWriter() class are used to write to a CSV file from a Python dictionary.
Syntax for "csv.DictWriter" class
Parameters
- csvfile - file object having write() method
- fieldnames - this parameter includes a sequence of keys that identify the order in which values in the dictionary should be passed
- restval (optional) - This parameter specifies the value to be written if the dictionary is missing a key in fieldnames
- extrasaction (optional) - this parameter is used to indicate what action to take if a key is not found in fieldnames, if it is set to raise a ValueError will be raised
- dialect (optional) - it is the name of the dialect to be used
csv.DictWriter class provides two methods for writing data in a CSV file, which include the following
-
writeheader(): This method is used to simply write the first row in our CSV file using pre-defined field names.
Syntax
Let us see the implementation of writeheader() using the following example:
Output
In the above example, a students.csv file will be created in the current working directory with the given entries. Here, we have opened the students.csv file in writing mode using open() function, and then the csv.DictWriter() function is used to create the CSV dictionary writer object. Then, the list of dictionaries is passed to the writer.writeheader() function to write the pre-defined field names.
-
writerows(): This method is used to simply write all the rows where in each row, only the values(not keys) are written to the CSV file.
Syntax
Let us see the implementation of writerows() using the following example:
Output
In the above example, a students.csv file will be created in the current working directory with the given entries. Here, we have opened the students.csv file in writing mode using the open() function, and then the csv.DictWriter() function is used to create the CSV dictionary writer object. Then, the list of dictionaries is passed to the writer.writeheader() function to write the pre-defined field names and the writer.writerows() function to write the data of the list to the CSV file. .
Extra Examples of Working with CSV File in Python
-
Creating CSV file with custom delimiters
A CSV file uses comma , as the default delimiter, but we can also use custom delimiters such as | or \t etc. In the example below we will see the implementation of how to create a CSV file having pipe | delimiter:
Output
In the above program, we have specified the optional parameter delimiter = '|' which is mainly used to specify the writer object that the created CSV file must have | as a delimiter.
-
CSV files having Quotes Let us consider a situation where we want our comma-separated values to be within quotation marks (""). The below example shows the implementation of how to create such a CSV file in Python:
Output
In the above program, we have specified the csv.QUOTE_NONNUMERIC as a value to the quoting parameter, here csv.QUOTE_NONNUMERIC is a constant which specifies the writer object that quotes should be added around each non-numeric entry. In the same example, we can also write CSV files with our custom quoting characters. For that, we will have to use an optional parameter called quote char.
-
Using Dialects Dialects are used to group multiple formatting patterns like delimiter, skipinitialspace, quoting, and escapechar into a single dialect name, thus removing redundancy when working with multiple files. A dialect is passed as a parameter to multiple writer or reader instances. The following example involves the use of a dialect:
Output
In the above program, we have used the csv.register_dialect() function to define a custom dialect that requires a name in string format. This created dialect is passed to the writer() object to specify that the writer instance, thus increasing the modularity of our program.
Conclusion
- CSV stands for comma-separated values, which is a simple file format to store data in a structured format.
- Python’s in-built CSV module helps us to deal with CSV files. There are various classes provided by this module for writing to CSV:
- Using csv.writer class
- Using csv.DictWriter class
- The with keyword allows us to both open and close the file without having to explicitly close it.
- The objects created using csv.DictWriter() class are used to write to a CSV file from a Python dictionary.
- Dialects are used to group multiple formatting patterns into a single dialect name, thus removing redundancy when working with multiple files.