How to End Program in Python
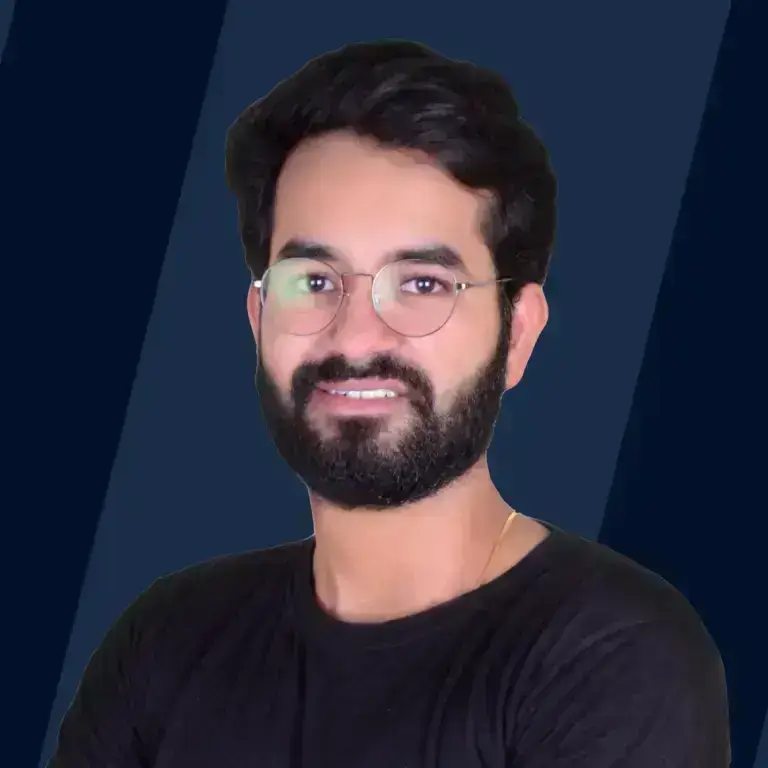
Overview
Giving a computer instructions on how to carry out a task is referred to as programming. A programming language was used to create these instructions. A script is a set of such instructions that have been organized.
The primary function of a programmer is to create scripts (i.e. programs). You must be aware of how scripts can end or terminate, though. We shall discuss many ways of "How to End Program in Python" in this article. This article doesn't require any prior knowledge, but it would help if you were familiar with Python basics.
How to End Program in Python?
You must be already aware that as a programmer we are supposed to create programming scripts. Scripts are created to carry out specific tasks, and they are meant to finish when those tasks are finished. We have a major issue if a script never ends. For instance, if the script contains an infinite while loop, the code theoretically never finishes and may need to be interrupted externally.
Sometimes, we intentionally write a script that is supposed to run infinitely. There is no issue with the infinite loop in this situation because it is intentional. Hence, if the script executes successfully and achieves its intended goals, it is great. But on the other hand, it would create a blunder if the script ends by throwing an error or raising an exception.
When we write a Python script, sometimes we realize that there is a need to stop the execution of the script at a certain point during execution. This can be achieved by Python exit commands.
Different Ways to End a Program in Python
We have got several ways through which we can end a program in Python. They are as stated below:
- Using KeyboardInterrupt
- Using sys.exit()
- Using exit() function
- Using quit() command
- Through os._exit() function
- By handling Uncaught Exceptions
- Through raise SystemExit
Now, let us cover all of these ways to end a program in Python in detail.
Ending Program in Python Using KeyboardInterrupt
One of the approaches to ending the program in Python is to manually stop it with keyboard interruption. Python scripts can be stopped using Ctrl + C on Windows, and Ctrl + Z on Unix will pause (freeze) the Python script's execution.
While a script is executing on the terminal, pressing CTRL + C causes the script to terminate and raise an exception. Let us look into the example below to understand it in further detail.
FYI: KeyboardInterrupt is a Python exception that is thrown when a user or programmer interrupts a program's usual execution. While executing the program, the Python interpreter checks for any interrupts on a regular basis.
Code:
Output:
Explanation: In the above example, we have written a code that will run infinitely. In simple words, we have written the code for an infinite while loop.
Now, midway through the code, we manually interrupt and stop its execution by pressing the CTRL + C on our keyboard. Hence, we are then prompted with the KeyboardInterrupt exception and our program ends abruptly. So we prevented this code from running infinitely by interrupting it through a keyboard and hence ended the program.
However, this is not the most efficient way since you can see, we got the KeyboardInterrupt exception while doing so, and the code exited abnormally.
If a KeyboardInterrupt exception occurs, we may use a try-except block in the script to perform a system exit.
Code:
Output:
Explanation: In the above code, we have again ended our Python program manually through keyboard interruption. But here we have smoothly handled the exception. The try-except block of code catches the KeyboardInterrupt exception and performs a system exit without throwing any error.
Ending Program in Python Using sys.exit()
One of the approaches to ending the program in Python is using the sys.exit(). The Python standard library contains the sys module. It offers functions and parameters that are system-specific.
exit is a sys module function that simply terminates the Python code. The output that we get from the sys.exit() might be different depending upon the environment we have written the code. Let us look into the syntax of sys.exit().
Syntax: Please note that we need to import the sys module before using the sys.exit().
Parameters: The sys.exit() function takes an optional argument that can be any string, integer, or any other object.
Now, let us first code and see how do we end the program in Python using the sys.exit().
Code:
Output:
Explanation: In the above code, we first imported the sys module to use the sys.exit() function. Then, we tried to check whether or not our variable satisfies the given condition. And, since in our code, the variable actually satisfies the condition, we end our Python program by using the sys.exit() function.
This is a very simple, yet convenient way to handle the exiting of the Python code when we are aware beforehand of when to stop our code.
Ready to take your Python skills to new heights? Enroll in Scaler Topics Python certification course and become a certified Python pro!
Ending Program in Python Using exit() Command:
The exit() function is in-built in Python and is defined in the site.py module. It can be considered as an alias for the quit() command (we will learn ahead in the article). The exit() command works only after the site modules are loaded, and hence it should not be used in the production-level codes.
The SystemExit exception is raised by the exit() command. In this case, exit(0) denotes a clean exit without any problems, whereas exit(1) denotes that a problem was caused during the program's termination.
Syntax: The syntax for exit() is very straightforward. We do not need to install or import any extra modules or packages because it comes in-built in Python. And it does not takes any parameters.
Code:
Output:
Explanation: In the above code, we tried to end our Python code by using the in-build function exit(). Hence, our for-loop ran only once and then exited. Even the next print statements were also not executed because the program terminated earlier due to the exit() function.
Ending Program in Python by using quit() Command
The quit() function can be considered an alternative to the exit() function in Python. It is also an in-built Python function that is used to terminate the Python codes.
When the system comes across the quit() function, it exits the Python program by closing the Python file itself. The quit() command also requires the site.py module to be imported. The SystemExit exception is raised by the quit() command in the background.
Syntax: The syntax for quit() is very straightforward. We do not need to install or import any extra modules or packages because it comes in-built in Python. And it does not takes any parameters.
Code:
Output:
Explanation: In the above code, we tried to end our Python code by using the in-build function quit(). Hence, our while loop ran only once and then exited. Even the next print statements were also not executed because the program terminated earlier due to the exit function.
Which to use -- sys.exit() or exit() or quit() at Production Level?
The exit() or quit() function also raises the SystemExit exception, but it is not handled like in the case of sys.exit(). As a result, it is preferable to end Python scripts in production code using the sys.exit() function.
Apart from that, the site.py module must be loaded before using the exit() or quit() commands. Hence, it is better to use the sys.exit() command at the production level.
Ending Program in Python Through os._exit() Function
In some circumstances, the os._exit() command can be used to terminate a Python program with a specific status, without invoking any of the cleaning handlers, flushing stdio buffers, etc. This function requires importing the os module before exiting the program with os.exit().
The os.exit() is generally used in the child processes after the os.fork() system call. Please note that it is a non-standard way to exit a process.
Code:
Output:
Explanation: In the above code, we terminated our Python program by using the os._exit() command.
Ending Program in Python by Handling Uncaught Exceptions
One of the approaches to ending the program successfully in Python is through handling the uncaught exceptions.
It is uncommon to build a script that works flawlessly on the first try; it typically requires multiple revisions. For the same reason, most of the scripts terminate with some uncaught exception, which eventually breaks our code and indicates that our script contains some bugs.
Hence, while writing code, we can insert try-except blocks in the areas which are prone to throw errors. It will then handle the execution of our code smoothly and end the code successfully, even if it contains some bug. This can be better understood with some examples. Hence, let us consider a scenario where our code can throw some errors.
Code:
Output:
Explanation: In the above code, we have tried to demonstrate how can an uncaught exception exist in our code, which will break our code if not handled carefully. So, we have created a fruitsdictionary and filled it with some data. While fetching the data from the dictionary using the dictionary key, there comes a point where the key does not exist in the dictionary. At that point, our code throws the KeyError.
Let us now see, how can we handle this exception and ensure a smooth ending of our Python code.
Code:
Output:
Explanation: In the above code, you can see how smoothly we handled the issue that was caused due to the missing key. We simply wrapped our code into a try-except block which prevented our code from breaking and performed the action we have defined in the except block.
This was just an example of how can we handle uncaught exceptions, but there are ample ways which you can use in your code to prevent any issue.
Ending Program in Python Through Raise SystemExit
SystemExit is an exception in Python which is raised by the sys.exit() method. The SystemExit exception is inherited from the BaseException. When the SystemExit exception is raised and is left unhandled, then the Python interpreter exits.
Interestingly, the SystemExit exception is raised in most of the methods of exiting Python programs (such as sys.exit(), exit(), and quit()) we discussed above, but we can also raise this exception directly. Let us look into the code for the same.
Code:
Output:
Explanation: In the above code, we have manually tried to demonstrate how the SystemExit exception is raised. Once this exception is raised, our except block handles this exception by performing the instructions we have given to our code.
In Python, the SystemExit Exception is raised when the sys.exit() function is called.
Code:
Output:
Explanation: In the above code, we have demonstrated the working of sys.exit. We see that once the limit is 100, it raises the SystemExit exception and the program is terminated, printing the message we have passed to the print statement -- 'Limit reached 100!!'. Use this Python Compiler to compile your code.
Related Programs in Python
Now that you have got a clear and crisp idea about "How to End Program in Python", I encourage you to go ahead and pick any of the below scaler articles to further enhance your knowledge in Python –
Conclusion
In this article, we learned about " How to End Program in Python ". Let us now go through the topics we covered and summarise them.
- When we write a Python script, sometimes we realize that there is a need to stop the execution of the script at a certain point during execution. This can be achieved by Python exit commands.
- We can stop the execution of a Python program by the sys.exit() function, quit() command, exit() command, and through raising systemExit. We can also handle uncaught exceptions for the smooth ending of Python programs.
- KeyboardInterrupt is a Python exception that is thrown when a user or programmer interrupts a program's usual execution. While executing the program, the Python interpreter checks for any interrupts on a regular basis.
- quit() function is inbuilt into Python and exits by closing the Python file. Since we have to load the site module to use it, it is not used in production codes.
- exit() command is also similar to the quit() command.
- Python’s in-built quit() function exits a Python program by closing the Python file.
- The sys.exit([arg]) command raises the SystemExit exception and has the in-built function to exit the program. It can be used in production-level codes.
- The os._exit() command is a non-standard way to terminate a Python program with a specific status.
- The SystemExit exception is raised whenever a program needs to terminate. We can also raise it directly.