How to Input a String in C++
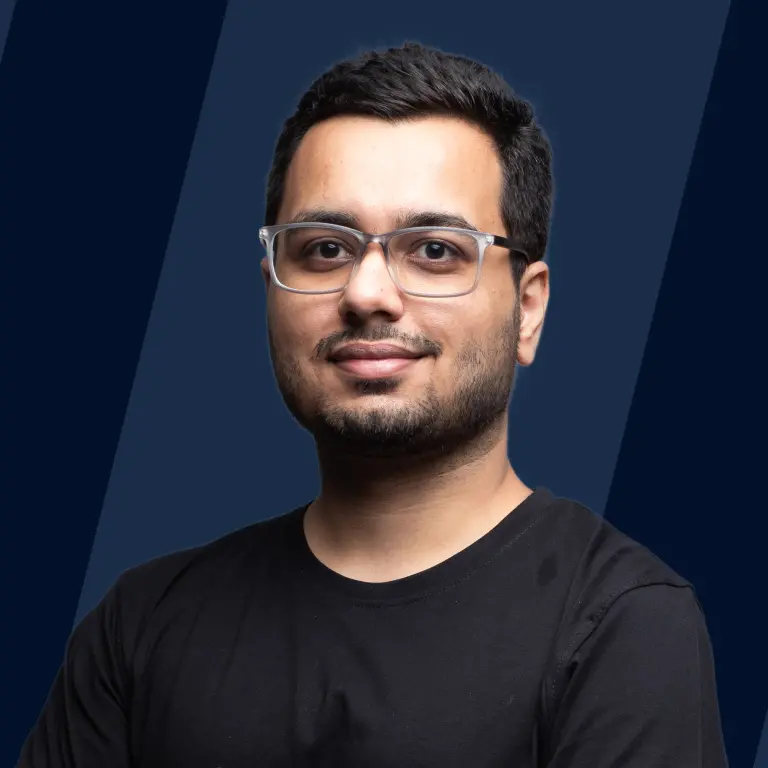
Overview
A string is a linear data structure defined as a collection or sequence of characters. We can declare a string by declaring a one-dimensional array of characters. The only difference between a character array and a string is that a string is terminated with the null character \0.
In the upcoming sections of this article, we will look at how to input a string in C++. We have 4 different methods of taking input from the user depending on the requirement and the form of the input string.
Introduction
The string data structure is an array or a group of characters. C++ has an inbuilt string class that can be used directly, or we can implement the arrays of characters to use a string data structure.
We can declare a string by declaring a one-dimensional array of characters. The only difference between a character array and a string is that a string is terminated with the null character \0.
We can declare the string in the same way we do for arrays, here the data type will always be the char of the elements stored in the string:
Here, char is the data type of the elements stored in the array, and stringArray is the array's name.
To assign values in the array, we can write:
We also have different ways to declare C-style strings:
Or
Alternatively, we can also do:
or
Utilizing all the space allocated to a character array is unnecessary while declaring its size. Still, it's suggested to allocate only THE space that the string requires to avoid any extra memory allocation loss, as we can see in the below stringArray declaration:
To return the value present at index 3, we can write:
The output of the above code would be 'l', as the value stored at index 3 is 'l'.
The character array will look like this:
Various Methods to Input a String
Now, we will see how to input a string in C++. By taking input of strings, we mean accepting a string from the user input. There are different types of taking input from the user depending on the different forms of a string. For example, the string can be a single word like "Hello," or multiple words separated by a space delimiter like "I am here to learn various methods to input a string".
In this article, we will closely examine four methods to input a string according to the requirement or the type of string that needs to be taken as input.
1. cin()
To provide our program input from the user, we most commonly use the cin keyword along with the extraction operator (>>) in C++. The iostream header file is imported using our program's cin() method.
The "c" in cin refers to "character," and "in" means "input". Hence, cin means "character input".
The cin object is used with the extraction operator >> to receive a stream of characters.
By default, the extraction operator considers white space (such as space, tab, or newline) as the terminating character. So, if the user wanted to take the string "hello" as the input, then cin() method will take the input as "hello", but on the other hand, suppose the user enters "I am Nobita" as the input string. Then, in that case, only the first word, "I" will be considered as the input, and the " am Nobita" string will be discarded because the cin() method will consider the white space after "I" as the terminating character.
So, while taking input with the cin() method, it will start reading when it comes to the first non-whitespace character and then stops reading when it comes to the next white space.
Syntax
The syntax of taking user input by using the cin() method is:
Here, we can write the cin keyword along with the extraction operator (>>), then mention the name of the string to be taken as the user input.
Example
Let us take a simple example to understand this:
The output of the above program is:
Explanation
As we can see from the output above, the string "hello" was taken as the input from the user and was displayed as the output.
Now, let's take another example: our input string will consist of more than one word to see the working of cin() method.
The output of the above program is:
Explanation As we can see from the output above, the string "I am Nobita" was taken as the input from the user, but only the first word, "I" was displayed as the output. As " " is the terminating character, anything written after " " was discarded. Hence, we only got "I" as the output.
Thus, the cin() method can only extract a single word, not a phrase or an entire sentence.
This is one of the significant limitations of the cin() method, as we cannot take input of the string consisting of multiple words separated by space, tab, or a new line character. This limitation is caused because we are using an extraction operator, which is the limitation of the extraction operator >>. To counter this limitation, we will look at other methods of taking input from the user.
Conclusion
- A string is a linear data structure defined as the collection or a sequence of characters. We can declare a string by declaring a one-dimensional array of characters. The only difference between a character array and a string is that a string is terminated with the null character \0.
- In this article, we looked at how to input a string in C++ and discussed different methods and examples.
- The cin object and the extraction operator >> are used to receive a stream of characters as a user input string.
- While taking input with the cin() method, it will start reading when it comes to the first non-whitespace character and then stops reading when it comes to the next white space.
- So, the cin() method can only extract a single word, not a phrase or an entire sentence.
- The gets() method in c reads characters until a new line occurs or you press enter or a return key.
- fgets() method is used to avoid buffer overflows as it checks the array bound, so it is safer to use than the gets() method.
- stringstream() method associates a string object with a stream allowing you to read from the string as if it were a stream. It is mainly used to "split" the string into different words treating the given string as a stream.
- Using the <sstream> header file, you can convert a given string into a StringStream object. For this, you need to use the type stringstream to create a constructor and pass the given string as input.
- getline() method takes an input of a sentence from the input stream until the delimiting character is encountered. If we do not add or specify any delimiting character in a getline() function, it will read characters until a new line occurs or you press enter or a return key.