How to Print String in C
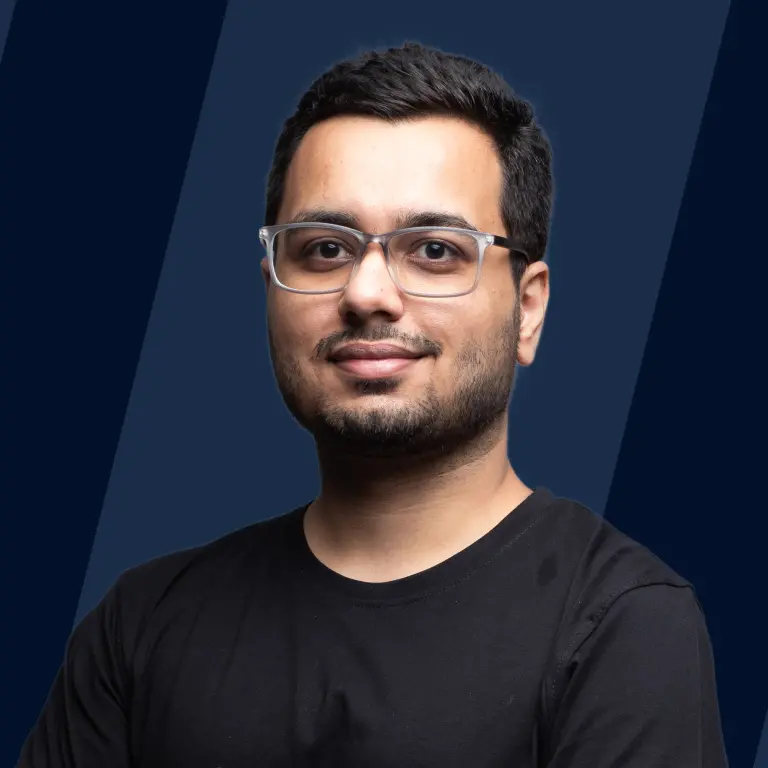
An array of characters that ends with a Null character (\0) is known as a string in C language. By default, the compiler adds the Null character (\0) at the end of a string of characters that are wrapped in double quotes (" "). A string of characters can be printed using puts(), printf(), loops, and the recursion method.
What are strings in C?
A sequence of characters in an array is known as a string in the C language. All the strings in the C language end with a null character (represented by \0).
Declaration of a string: A string in C can be declared very easily, similar to defining a one-dimensional array. The fundamental syntax for defining a string is shown below:
In the syntax, <size> represents the string's length or the number of characters the array can hold. string_name is any name supplied to the array name. Please be aware that strings differ from standard character arrays, in that they have an additional terminating character, the Null character (\0), which indicates the end of the string.
Initializing a string: Let's look at different ways to initialize a string in C language. There are several ways to initialize a string. We'll provide examples to demonstrate this:
1. char str[] = "String";
2. char str[12] = "Programming";
3. char str[] = {'S', 't', 'r', 'i', 'n', 'g', '\0'};
4. char str[13] = {'P', 'r', 'o', 'g', 'r', 'a', 'm', 'm', 'i', 'n', 'g', '\0'};
Diagrammatic Representation: Below is the representation of how the string literal string is stored in the memory. string value is stored in the memory in a sequence of characters concerning an index value starting from 0. Every string literal stored in the character array is terminated by a null character (\0), i.e., present at index 6 in the below representation.
How to print string in C?
Let's see how to print a string in C. There are many ways to print strings in the C language. As we know, a string is a character array, so we can loop over the array or use some built-in functionalities of the C language to print a string.
In the sections below, let's look at the methods used to print a string.
puts() function for printing a string in C
puts(<str_name>): puts() function takes a string character array name as an argument to print the string in the console.
Example C Program:
Output:
Explanation: We have declared a char array with size 20 and initialized it with a string literal "Have a good day!". puts() is used to print string in the C console output window.
printf() function to print a string in C
We can use the printf() function to print a string in C language. It takes the string as an argument in double quotes (" "). The functions scanf() and printf() can be used to read input and display the string, respectively.
printf("%s", <str_name>): We can use the %s format specifier in the printf() function to print the character array in the console.
Example C Program:
Output:
Explanation: We have declared a char array with a str name and 15 size. str is initialized with the "learning" string literal. %s format specifier is used in the printf() function to print the string literal in the output.
Printing a string in C using loops
Loops can be used to print an array of characters (string). We can iterate over every character in the array and simultaneously print the character in the output.
Example C Program:
Output:
Explanation: We have initialized a char array with string literal, the array name is str with size 7. We have used a while loop statement to iterate over the array till the null character is encountered and simultaneously printed every element of the character array.
Printing a string in C using recursion
The recursive approach can also be used to print a string in C. We can define a function and repeatedly call the function itself to print a single character in a single call till the termination condition is reached, i.e., when a null character is encountered.
Example C Program:
Output:
Explanation: We have declared a char array with str name and 15 size. str is initialized with the "Recursed" string literal. We have passed the address of the first element of the char array as an argument in the printString() function. The printString() function calls itself by printing one element with one call till the terminating condition is reached, i.e., when a null character is encountered.
Examples for understanding
Input string containing spaces:
Let's see how we can take an input and print a string containing spaces. We use the gets() function to take a string with spaces, as the enter key is the only delimiter in the gets() function. However, in the scanf() function, space is a delimiter. So, we can't use scanf() with strings having spaces.
Input:
Output:
Explanation: We have used the gets() function to take an input string from the user containing spaces, as spaces are not considered a delimiter in the gets() functionality. We have printed the string using the printf() function.
Conclusion
- An array of characters that ends with a Null character (\0) is known as a string in C language.
- We can print a string in C using puts(), printf(), for loop, and recursion method.
- To print the string in the console, we have to pass a string character array name as an argument to the puts() function.
- We can use the %s format specifier in the printf() function to print the character array in the console.
- gets() function does not consider space as a delimiter. However, the scanf() function considers space as a delimiter when taking input strings from the user.