How to Sort a String in C++?
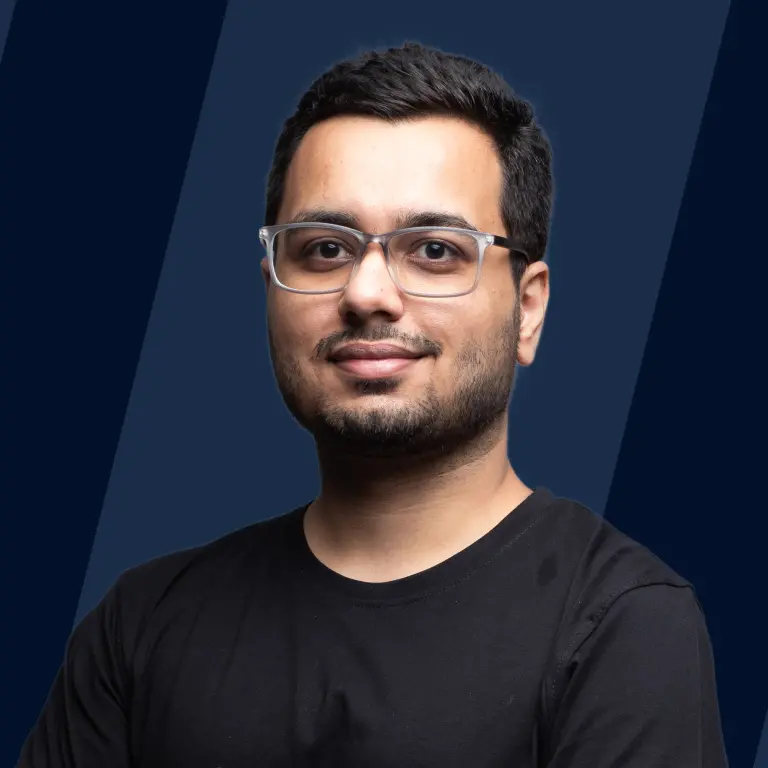
Overview
Different sorting techniques are introduced to sort numbers, strings, or arrays in C++. We will be looking at different sorting techniques with different time complexities to sort a string.
Introduction
Sorting algorithms use comparison operators to decide whether the array should be rearranged in ascending or descending order. Heap sort, fast sort or merge sort, bubble sort, and insertion sort are several techniques.
We'll sort strings in this article using a variety of techniques. We shall use the STL library function <algorithm> to sort the names by string length.
Methods to Sort String in C++
Using Sorting Techniques :
1. By Bubble Sort :
Let us sort a string using the Bubble sort technique. But first, understand what bubble sort is and how bubble sort works. In the bubble sort technique, the adjacent strings(a[i] and a[i+1]) are swapped when the successive string is smaller (a[i] > a[i+1]).
Algorithm :
- Step - 1 :
Get array input from the user. - Step - 2 :
Use strcpy to swap the name of the strings. - Step - 3 :
Nested for loop is used to compare two strings and traverse through them. - Step - 4 :
If the ASCII(letters, numbers and characters assigned to the 8-bit codes) value of y is greater than y+1, then the values are swapped. - Step - 5 :
Swapping is done until the condition returns false.
Example :
We will use a character array as user input in this code which will take 10 character strings as input. We have created a nested loop where the “strcpy” function is used for swapping the name of the strings. The "strcmp" function is used for comparing two strings in the if statement.
Output :
As a result, we get sorted alphabets in ascending order.
The worst-case time complexity is , and the best-case time complexity of bubble sort is .
2. By Insertion Sort :
In Insertion sort, we divide the array into sorted and unsorted sublists.
We compare the values in the unsorted part, and after sorting them, we put them in the sorted sublist.
We consider the first element of the sorted array as a sorted sublist. All the elements of the unsorted sublist are compared with the element in the sorted sublist.
All the greater elements are shifted to the right.
Example :
Output :
The elements are arranged in ascending order.
The worst-case time complexity is , and the best-case time complexity of insertion sort is .
3. By Quick Sort :
In Quick sorting, we assign a pivot value that can take any place in the array. Suppose we pick x as the pivot value for the current array. Then the values greater than x are arranged after x, and the smaller values than x are arranged before x.
Example :
We will declare two variables' start and end points to the character string. We will divide the array in half, and using the do-while loop, we will swap the elements of the array and repeat the process until the string is sorted.
Output :
If the string includes capital letters, the capital alphabet will be sorted first, and then the small letters.
The time complexity for the above sorting technique is where the value of n is the length of the string.
The worst case time complexity is and best case time complexity of quick sort is .
Using Hashing :
As we know, there are only 26 alphabets. Therefore, we will create a hashed array to store alphabets from a-z. Indexing of hashed array starts from a and goes to z.
Example :
Output :
If any capital letter is included in the string, it doesn't get sorted and is also not visible in the output. The sorting is done in ascending order.
Using Library Function :
C++ has an inbuilt library function <algorithm> that gives us access to the sort method.
We will create an array of name strings where the inbuilt sort function will take the name of the array and its size as an argument to sort the strings.
Syntax :
Example :
Output :
In this program, only the first word gets sorted because if the sort function encounters a null value, it stops the execution.
C++ Pprogram to Sort String in Ascending Order
We have already seen quite a few techniques to sort strings in ascending order, like the bubble sort, hashing, etc.
Let us sort strings using the bubble sort technique, as we have already seen the bubble sort algorithm, where we sorted multiple strings alphabetically.
Here, we will sort a single string by getting user input using gets that reads the characters from stdin and stores that in the str, and we will also use strlen to return the length of the string.
Code :
Output :
In the result, the string positive is sorted in ascending order because we are swapping the positions of the bigger position alphabet with the smaller alphabet here using bubble sort.
C++ Program to Sort String in Descending Order
To sort the string in descending order, we will use the above program only, but we will change the if loop slightly.
Code :
Output :
The string is sorted in descending order because now, while we traverse through the array, we swap the values where smaller alphabets are replaced with the large value alphabets using bubble sort.
C++ Program to Sort String (Names) in Alphabetical Order
Let's sort strings in alphabetical order using the vector of strings and STL library function std::sort from library <algorithm>.
Code :
We are using const auto &element in for loop because it does not make copies, and also, using const here means that it won't allow us to make any modifications and also signify your intent.
Output :
C++ Program to Sort String by Length
To sort the string according to the length, we will use the above code and make a slight change in the sort function, where we will return the string according to the element's size.
- We are creating an array using a vector to be sorted according to the length.
- In the next step, we print all the array elements using for loop where const auto &element syntax is used.
- Next, the sort function is used as part of the library <algorithm>, where we will compare consecutive string lengths using the inbuilt size function.
- In the next step, all the sorted elements according to the length are printed.
Output :
Conclusion
- There are different techniques introduced to sort strings in different ways like bubble sort(where we swap the adjacent elements), insertion sort(array is divided into sorted and unsorted arrays and then solved), hashing, quick/merge sort(it is a divide and conquer algorithm).
- Quick sort is a type of divide and conquer algorithm.
- To sort strings using hashing, a hashed array is created of all 26 alphabets.
- The time complexity with bubble sort to sort the string is .
- The sort function is a part of the <algorithm> library.