Hybrid Inheritance In Java
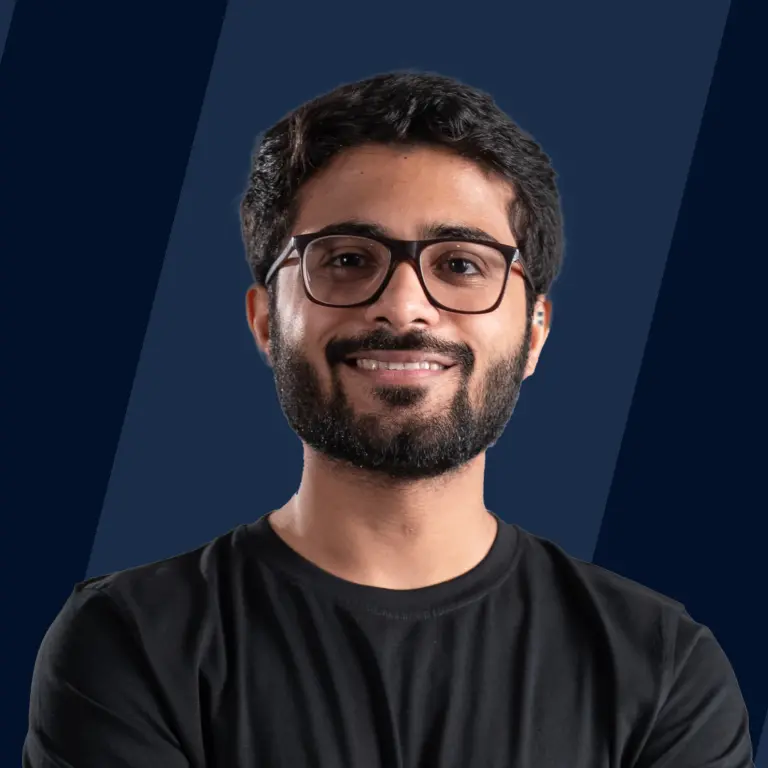
Overview
Hybrid Inheritance in Java is one of the types of inheritance where two or more inheritance types are implemented simultaneously. In fact, the word Hybrid inheritance itself suggests that it is a combination of two or more types of inheritance. In simple words, when we use many types of inheritance together, it is said to be Hybrid Inheritance in Java. The main advantages of Hybrid Inheritance in Java are Reusability and Modularity of the code. Let us dive deep into the concept of Hybrid Inheritance in Java.
Hybrid Inheritance in Java
The word "Hybrid" inheritance itself suggests that it is a combination of many types of inheritance. You might have heard about something called hybrid cars which are capable of operating on both petrol as well as electricity. The same is the case with Hybrid inheritance.
Hybrid inheritance is basically a combination of more than one type of inheritance in Java. It can be the combination of "Single and Multiple Inheritance", "Multilevel and Hierarchical Inheritance" or any other combination based on the requirement of your program. The main purpose of Hybrid Inheritance is to make it easier for the programmer to use the methods and properties already present in other classes to increase the reusability of the code. It is also helpful in making the code more modular by creating specific classes for specific entities such as Student class, Employee class, etc.
Let us now discuss how to implement Hybrid Inheritance in Java.
Implementation of Hybrid Inheritance in Java
When we combine more than one inheritance type in a single program, Hybrid inheritance comes into the picture. In this article, we are going to see two different combinations that lead to Hybrid Inheritance in Java. So without further ado, let us now see how to implement Hybrid Inheritance in Java using
- Single and Multiple Inheritance
- Multilevel and Hierarchical Inheritance
Using Single and Multiple Inheritance.
Before moving to the main example, let us quickly go through the concept of Single and Multiple Inheritance.
Single Inheritance: When a child class inherits the properties and methods of a superclass, it's called Single Inheritance, simple as that. Here's the diagrammatical representation of the same.
Multiple Inheritance: When a child class inherits the properties and methods of more than one parent at the same time, it's called Multiple Inheritance.
Note: As Java does not support Multiple inheritances using classes, we used an interface to implement multiple inheritance in Java.
Interface: In Java, an interface is a reference type which is similar to class. Basically, it is a collection of abstract methods. Classes in Java implement the interface(s) to inherit the abstract methods of the interface(s).
Code:
Output:
Explanation:
Please refer to the above-given image for reference.
In the above-given example, class B(child class) extends(or inherits) class A(Base class) which is an example of Single inheritance and as Java does not support Multiple inheritances (using classes), we used an interface to implement multiple inheritances in Java. Class D extends class B and implements interface C which is an example of Multiple inheritances. As we have used both of these types of inheritance simultaneously in our program, it is referred to as Hybrid Inheritance in Java. This is how we easily used the properties of base classes(or interfaces) in the child classes.
Let us now see another way of implementing Hybrid Inheritance in Java.
Using Multilevel and Hierarchical Inheritance.
Before moving to the main example, let us quickly go through the concept of Multilevel and Hierarchical Inheritance in Java.
Multilevel Inheritance: When a child class inherits the properties and methods of a superclass and that superclass inherits another class, it's called Multilevel Inheritance, simple as that. Here's the diagrammatical representation of the same.
Hierarchical Inheritance: When more than one child class inherits the properties and methods of the same parent class, it's called Hierarchical Inheritance. Here's the diagrammatical representation of the same.
Code:
Output:
Explanation:
Please refer to the above-given image for reference.
In the above-given example, class C extends class B and class B extends class A(Base class), this is an example of Multilevel inheritance and class D extends class B while class C also extends class B, it simply means that multiple classes(like C and D) are extending the same super class (or parent class), altogether this is an example of Hybrid Inheritance in Java as more than one types of inheritance are getting implemented in the same program. Using this way we easily used the properties of base classes in the child classes.
Conclusion
Some of the important points discussed in this article are mentioned below:
- Hybrid Inheritance in Java is one of the types of inheritance where two or more inheritance types are performed simultaneously.
- The main advantages of Hybrid Inheritance in Java are the "Reusability" and "Modularity" of the code.
- It can be the combination of “Single and Multiple Inheritance”, “Multilevel and Hierarchical Inheritance” or any other combination based on the requirement of your program.
- It is also helpful in making the code more modular by creating specific classes for specific entities such as Student class, Employee class, etc.
Also see
Java Clean Code - How to Write Clean Code in Java | Scaler Topics