Infinite Loop in Java
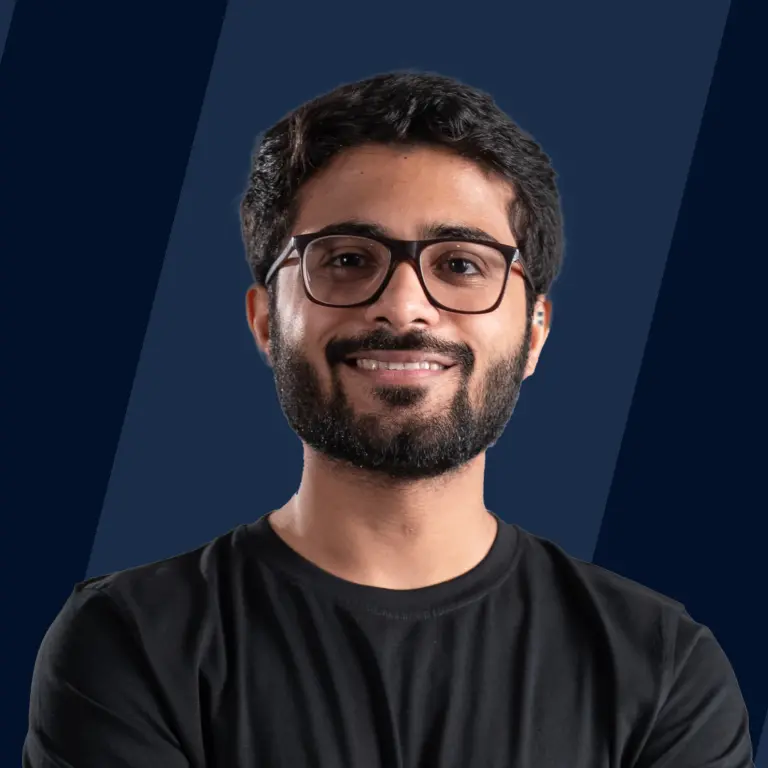
In Java, an infinite loop endlessly executes without a stopping condition, potentially causing resource overuse or application crashes. While it can result from programming errors, it might serve intentional purposes based on specific application needs. Recognizing Java's loop types and behaviors is vital for effectively handling and preventing unintended infinite loops.
Using While Loop
Syntax of While Loop
The code part inside the body of while loop is executed if the condition is true else the while loop is terminated. So we have to provide a condition that will always evaluate to true and this can be achieved by passing true itself as the condition in the above loop.
Code:
Output:
Explanation:
If we try to run the above code, the code will print Hello World as the while loop keeps on executing for infinite time.
IMPORTANT: If there is a time limit on the period of execution, TimeLimitExceeded error will be thrown.
Using For Loop
Syntax of for loop
Let's have a brief recap of for loop in Java, the initialization expression is used to initialize the counter, and the condition expression tests whether we have to execute the body of the for loop or we have to terminate the loop. The updation expression is executed after each execution of the for loop.
Let's have an example of for loop in Java,
Output:
Explanation:
- In the initialization part, the variable i is assigned the value , and if i is less than the body of the for loop is executed.
- After each execution of the for loop, i is incremented by . After executing the for loop for , i becomes , and the condition evaluates to false thus resulting in the termination of the for loop.
So in the case of for loop in Java, we can also pass the condition expression as true so that the for loop executes for infinite time.
Output:
Explanation:
- Here, the body of the loop is executed till i is less than 3.
- However, instead of incrementing the value of i, we are decreasing it in the updation statement.
- As a result, the condition is always true and the loop executes infinitely.
But here is a twist, in the case of for loop the expressions initialization, condition, and updation are optional whereas in the case of while loop the condition expression was mandatory. So if we leave this expression as empty, the above for loop will execute for infinite time.
Output:
Using a do-While Loop
Syntax of do-while loop
The do-while loop is similar to the while loop in Java, but with one minor change. The do-while loop executes the do block of the loop and then checks the condition. So the do-while loop is executed atleast once even if the condition is false.
Let's have an example of this:
Output:
And just like the while loop, the condition expression is mandatory.
Like we did in the while loop, we will pass true as the condition and the above loop will execute for infinite time.
Output:
Now we have learned the infinite loops in Java, let's move on to the intentional infinite loops in Java.
Intentional Infinite Loops
Sometimes we intentionally want an infinite loop for example in a web server. A typical web server accepts a request for a web page, such as a communicative message, and returns a web page while waiting for the next request.
Thus the web server needs to be active always to read and process upcoming requests. This can be achieved by using infinite loops as,
or,
Conclusion
- An infinite loop is a sequence of instructions that loops indefinitely unless the program is terminated or the system crashes.
- In the case of a while loop in java we can pass true as the condition to execute the while loop for infinite time, in the case of a for loop in java we can leave the expression empty to execute the for loop for infinite time, in the case of a do-while loop in java we can pass true as the condition to execute the do-while loop for infinite time.
- Most of the time infinite loops in Java are considered a programming error, but these can be intentional such as in the case of a web server.
- If there is a limit on the period of execution of a program, TimeLimitExceeded (TLE) error is thrown.