Iterate List in Python
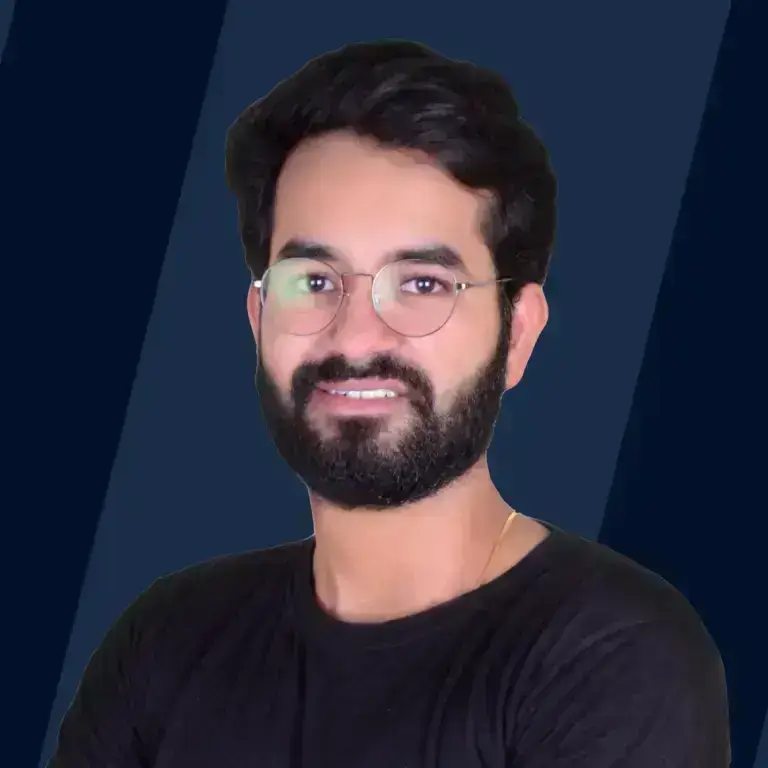
Overview
Iterating a list means going through each element of the list individually. We iterate over a list whenever we need to use its elements in some operation or perform an operation on the elements themselves. There are several ways to iterate through a list - using loops, the range method, list comprehension, enumerate function, and using the numpy module.
How to Iterate a List in Python
Before we dive into the core, let's understand the basics and go through each term.
What are Lists?
The list is an inbuilt data structure in Python used to store and use data items collectively and efficiently. Formally, a list can be defined as a group of data items referenced under a common name. Lists have the extra benefit of being dynamic in size, which means that, you don't have to specify the size of lists beforehand, size is determined by the elements created in the list. Lists show benefits like, it can hold data items with different data types, but it also has a disadvantage to it. Since lists are designed to hold data items with different data types, these items are not stored in contiguous memory locations and will be slower while computing a huge amount of data.
What are Arrays?
Since we now know about lists, let's go across a similar data structure called arrays. Arrays are also designed to serve the same purpose, but it is different from lists. Arrays store data items of similar data types. So a formal definition could be made as arrays are a group of data items of similar data type referenced under a common name. Since arrays hold data items of similar data types, these data items are stored in contiguous memory locations and are faster than lists.
What is the Principle of Iteration?
Now let's jump the second hurdle, the term iteration. What is iteration? Iteration simply refers to the technique of repeating a mathematical or computational procedure to the result of a previous application. In essence, iteration means, repeating the same task over and over again and sometimes inferring from these results. Iterations in programming are easily achieved through the use of loops. We will see to that later.
Why do We Need Iteration?
Now we know what iteration means. Now let's see why we need to use it. Iteration means simply going over the same task over and over again to achieve the result. Let's talk about the need for iteration concerning programming, after all, that is the core of this article. Suppose given a simple task to compute the multiplication table of a number. One might ask the user for the input number and ask for a limit, that is till which multiplier. For small input of the multiplier, the programmer can just display the output by computing manually, but what if the input is big, say the value of the multiplier is 100 or 1000. Computing manually is no longer an option. So what the programmer can do is repeat the same logic of computing the input number and the first multiplier over1000times in a loop, and make a change or increment to the multiplier. So in essence the same logic is run over and over again for how many times the user wants. To solve the restrains on manual computation is why we use the principle of iteration and loops.
Let's see the same program in action,
This program will illustrate the use of iteration in Python.
Output:
Here the multiplicand was 2 and the maximum multiplier was 10, and you can see how we iteratively performed the multiplication operation, saving time and effort.
We now know, what and why we need iteration. Iterating over lists and arrays is a really important task in much real-world application software. One can even say the core of algorithms is based on the principle of iterations.
Before we iterate over lists, let's see how we can create one.
Output:
Here, we created lists called items are stored values into it.
Now that we have created the list, let's go through different ways to iterate over it.
Method 1: Using For Loop
In this method, we will be using the for loop which comes under default Python functions.
Using for loops we will be iterating over lists in Python.
Syntax:
The for loop does three things, first initializes the iterator to a fixed value and second iterates through the iterable, and then third updates the iterator by the specified choice.
- iterable is the structure we want to iterate over.
- iterator is the variable used to keep track of the count of iterations over the iterable.
Output:
With the help of the in operation and the for loop we iterated over the list of items, and thus iterating over lists in Python.
Method 2: Using range() Method
In this method, we will be using a function called range which comes under default Python functions.
Using the range() function we will be iterating over lists in Python.
Syntax: range(start, stop, step)
The range() function takes 3 arguments, let's see what those arguments are.
-
start (optional): specifies the starting value of the number to be iterated, the default is 0
-
stop (mandatory): specifies the maximum value of the number, till which number to iterate up to.
-
step (optional): specifies the interval to take values.
Now let's see how to use it.
Output:
Here we accessed each element of the list items using the range() function and a for loop, and thus iterating over lists in Python.
Method 3: Using while Loop
In this method, we will be using the while loop which comes under default Python functions.
We will be iterating over lists in Python using the while loop available in Python.
Syntax:
The while loop only allows the code statements to be executed if and only if, the condition provided to the while loop returns true.
Output:
Here we accessed each element of the list items using the while loop, and thus iterating over lists in Python was done.
Click here, to learn more about While Loop in Python.
Method 4: List Comprehension
List comprehension is a technique that offers a shorter syntax when one wants to create a new list based on the values of an existing list.
Syntax:
A list comprehension works by translating values from one list into another by placing a for statement inside a pair of square brackets [], formally called a generator expression.
A generator could be called an iterable object, which gives a range of values.
This reduces the syntax as you don't have to follow the indentation of the for loop created.
We will use the list comprehension method, for iterating over lists in Python here.
Let's see how it works.
Output:
Here the statement print(i) is executed just like how it would be executed in a normal for loop.
We were succesful in iterating over lists in Python.
List comprehension is usually used where a small change is required, allowing us to shorten the syntax of iteration over loops. But one can also use list comprehension with comparison operation, nested lists or even nested for loops.
Check out this article to knoe more about List Comprehension in Python.
Method 5: Using enumerate()
When we want to convert the list into an iterable list of tuples or get the index based on a condition check, for example in a searching algorithm, we might need to save the index of the maximum or minimum element, we can make use of the enumerate() function.
Let's see how we can iterate over lists in Python using this.
Syntax:
input: iterable output: index and value of the corresponding index in the iterable.
Output:
Here, both the value of the item as well as its index can be obtained.
We have succesfully iterated over the list in Python.
The Enumerate() method works similar to a counter for an iterable data structure like lists and tuples in Python and returns the output in the form of an enumerating object, which means both index and value are coupled together.
It is mostly used, to keep track of counters in an iterable data structure.
Method 6: Python NumPy
While dealing with very large n-dimensional lists (list of lists or an image array), it is wiser to make use of an external library such as NumPy. Using functions from the NumPy package, we can iterate over lists in Python.
Let's see how.
Note: Here we make use of numpy as np as an alias for numpy, to avoid using the keyword numpy over and over again. This same time as well as makes the code look neat.
Here we make use of a function in NumPy called nditer(), which stands for n-dimensional iteration. The nditer() function allows one to iterate over an n-dimensional array, by going through each of the items in the array.
Note: The input to the nditer() function should always be an n-dimensional NumPy array. So you have to convert the said list into a NumPy array, using the functions available in the NumPy package.
Output:
And thus iterating over lists in Python was done using the nditer() function from the NumPy package.
Conclusion
- Lists are an inbuilt data structure in Python used to store a group of items with dissimilar data types.
- Arrays are a data structure used to store a group of items with similar data types.
- We have learned the principle of iteration and why we need it.
- We have also seen various methods to iterate over lists and arrays.