Java String Interpolation
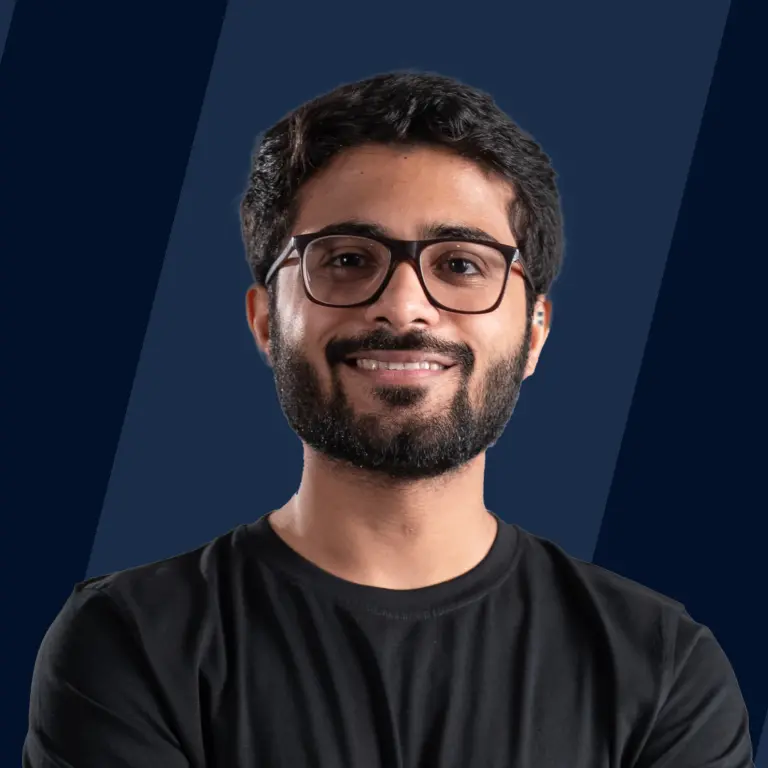
Overview
Java String Interpolation is the process of replacing the placeholders in a string in Java with variable values. Java string interpolation is used to implement dynamic strings in Java.
Introduction to Java String Interpolation
Java string interpolation refers to the process of replacing certain placeholders in strings in Java with variable values. A string in Java is provided with placeholders and these placeholders are replaced by variable values.
String Interpolation makes the code more compact and avoids the repetition of using variables to print the output. String Interpolation replaces the placeholder with the mentioned variable names assigned to strings and hence makes it efficient to write large variable names or text.
For example:
Suppose we have a string to print the name of a person which goes as follows:
Now suppose we have to print the name of another person Bruce, then the output will be:
Here we can observe that the "My name is " part of the string is repetitive and the part that is changing is the last word.
Thus the changing portion of the string (the last word in this case) can be replaced by a placeholder that will contain values according to the input.
Thus in Java, we can write the string in the form:
Now the placeholder can be replaced with either Bruce or Peter.
Note: The syntax for java string interpolation has been discussed further and the above expression is just a symbolic representation.
Need for String Interpolation
Following are the needs of Java string interpolation:
- It helps us to avoid repetition in code.
- It helps in improving the efficiency of the code.
- Makes code less cluttered and more readable.
Ways to Implement String Interpolation
Now that we have an overview of java string interpolation, let us learn how to implement java string interpolation.
There are primarily four-way to implement String Interpolation in Java:
- String interpolation using the plus (+) Operator
- String interpolation using String.format() Method
- String interpolation using MessageFormat Class
- String interpolation using StringBuilder Class
All of the above methods have been further discussed in the article.
String Interpolation Using the plus (+) Operator
In this section, we will learn how to achieve java string interpolation using the + operator. The + operator can be used to concatenate the variables and string values together. The + operator is placed outside the double quotes and when the string is interpolated in Java then the variables are replaced with their respective values.
Code:
Output:
Explanation::
- The main() method declares and initializes two string variables, "str1" and "str2", with the values "Java" and "programming", respectively.
- Then, a string interpolation is performed using the concatenation operator "+" to combine the values of the variables and a string literal to create a new string.
- This interpolated string is then printed to the console using the "System.out.println" statement.
Using String.format() Method
In this section, we will learn how to achieve java string interpolation using the String.format() method. The String.format() method is used to separate the input string and the variables. Within the string, the placeholders (%) are used as placeholders for the variables. The String.format() method accepts this string as the first parameter followed by the variables as the parameters. These variables then replace the % in the string in a sequential manner.
Code:
Output:
Explanation of the example:
- In this code, we are using string interpolation using the String.format() method to create a string that combines the values of two variables str1 and str2 with a fixed string in between.
- The String.format() method takes a format string as its first argument and a variable number of arguments as subsequent arguments. In this case, the format string contains two placeholders %s, which indicate that two string arguments will be substituted into the string.
- The first %s is replaced with the value of str1, and the second %s is replaced with the value of str2.
Using MessageFormat Class
In this section, we will learn how to achieve java string interpolation using the MessageFormat Class. To use the format method we need to import MessageFormat class.
The format method in this case behaves similarly to the String.format() method provided by Java. It is used to separate the input string and the variables. Within the string, the placeholders are used for the variables. The placeholder is in the form '{0}, {1}..' within the input string. The format() method accepts the input string as the first parameter followed by the variables as the parameters. These variables then replace the placeholder in the string in a sequential manner.
Note: The sequence here means the placeholder {0} will be replaced by the first variable, {1} will be replaced by the second variable, and so on.
Code:
Output:
Explanation of the example:
- In the above example, we have defined two strings and stored them in variables str1 and str2.
- Now when the "{0} is a high-level, class-based, object-oriented {1} language" part is interpolated then String.format() would replace the first placeholder {0} with the value of str1 and the second placeholder {1} with the value of str2.
Using StringBuilder Class
In this section, we will learn java string interpolation using the StringBuilder Class.
Code:
Output:
Explanation of the example:
- First, two strings are declared and assigned values: "Java" and "programming".
- Then, a StringBuilder object is created and initialized with the value of str1 (which is "Java").
- The append() method is then called on the StringBuilder object twice: once to add the string " is a high-level, class-based, object-oriented " and another time to add the value of str2 (which is "programming").
Conclusion
- Java String Interpolation is a process of replacing placeholders in strings with variable values in Java. This makes the code more efficient, less repetitive, and more readable.
- There are primarily five ways to implement Java String Interpolation, which includes using plus (+) operator, String.format() method, MessageFormat class, and StringBuilder class.
- Java string interpolation can be achieved by using + operators by concatenating strings with variables.
- Java string interpolation can also be achieved by using inbuilt methods like String.format().
- StringBuilder class, MessageFormat class can also be imported into the Java program to implement interpolation in Java.