Comparable and Comparator in Java
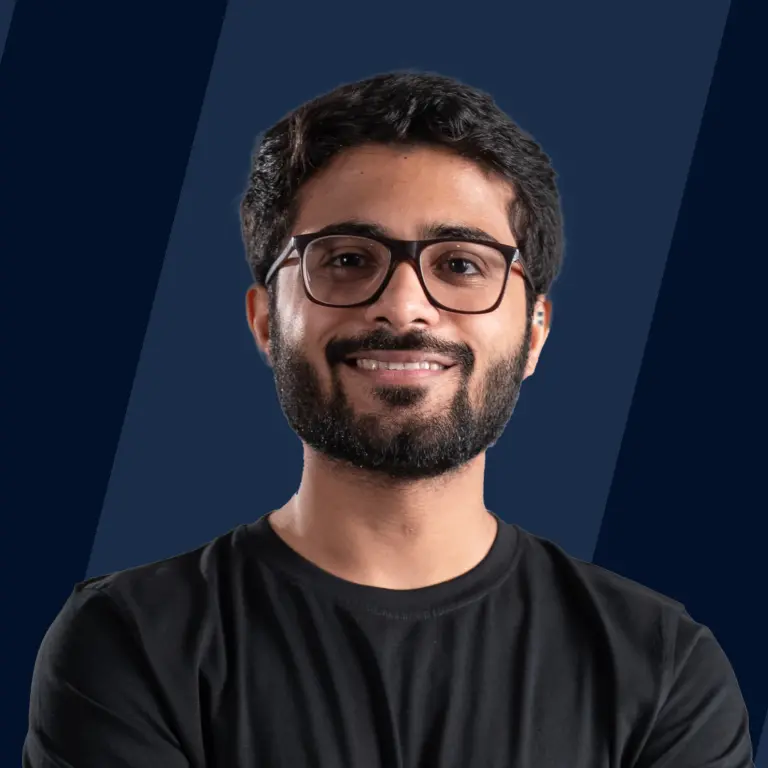
To sort custom objects like Student or Employee, we need to provide explicit sorting logic.
To achieve this, Java provides the Comparable and Comparator interfaces. Comparable and Comparator in Java allow us to define custom sorting behavior for objects, including sorting based on multiple data members.
Difference Between Comparable and Comparator in Java
The key differences between Comparable and Comparator in Java is given below. We will understand these further in the article.
Comparable | Comparator |
---|---|
Comparable is an interface in Java. | Comparator is a functional interface in Java. |
Comparable provides compareTo() method to sort objects. | Comparator provides compare() method to sort objects. |
Comparable is a part of the Java.lang package. | Comparator is a part of the java.util package. |
Comparable can be used for natural or default ordering. | Comparator can be used for custom ordering. |
Comparable provides a single sorting sequence. Ex: Sort either by id or name | Comparator provides multiple sorting sequences. Ex. Sort by both id and name. |
Comparable modifies the class that implements it. | Comparator doesn't modify any class. |
Using Comparable Interface
Comparable is an interface in Java that enables comparing an object with other objects of the same type. Comparable interface is provided by the java.lang package. Several built-in classes in Java like Integer, Double, String, etc., implement the Comparable interface.
Comparable is used for sorting objects by natural or default ordering, meaning the object itself knows how it has to be ordered. Example: The SuperHero objects should be ordered by id.
Comparable provides single sorting sequence. It means that the objects can be sorted based on a single data member. Example: The SuperHero object can be sorted based on single attribute like id, name or age.
Syntax
A class has to implement Comparable interface and implement the compareTo() method to enable comparing it with other objects of the same type.
Methods used in Comparable
compareTo()
Comparable interface has exactly one method compareTo() which accepts a single object as parameter and returns an integer value. Java determines the sorting order based on the interger value returned from the compareTo() method.
Syntax
Consider an object x is compared with y via the statement x.compareTo(y), then the value return from the method should be
- 0, if values in x and y are equal.
- Negative, if the value in x is less than in y.
- Positive, if the value in x is greater than in y.
Example of Comparable in Java
Consider creating a REST API that fetches a list of superheroes from the server.
- GET /superheroes: Returns superheroes sorted by ascending id.
- GET /superheroes?sort=name: Returns superheroes sorted by ascending name.
- GET /superheroes?sort=age: Returns superheroes sorted by ascending age.
Let's define the SuperHero class with attributes id, name, and age before proceeding.
We will take our first use case, GET /superheroes. This endpoint should return the list of superheroes ordered by id in ascending order.
We need to make the SuperHero class be sorted by id in ascending order. This can be achieved in two steps.
Implementation
- Make SuperHero class implement the Comparable interface.
- Override the compareTo() method.
Output
Explanation We have implemented the Comparable interface and overriden the compareTo() method to sort SuperHero objects by id. Collections.sort() internally calls the compareTo() method of SuperHero objects to sort them by id.
Using Comparator
Comparator is a functional interface in Java that can be used to sort objects, and it is provided by the java.util package.
A functional interface is an interface that contains exactly one abstract method. It is also called Single Abstract Method (SAM) Interfaces.
But wait, this is what the Comparable interface does. Then why do we need the Comparator interface? If a class implements the Comparable interface, then it is aware of how to sort itself because the class itself has implemented the compareTo() method.
This is called default ordering. Comparator is used for custom ordering where the class is unaware of the ordering logic.
Comparator provides multiple sorting sequence (i.e.) sorting objects based on multiple data members. Example: SuperHero objects can be sorted based on multiple attributes like id, name and age.
Syntax
Comparator can be created using a lambda that accepts two objects of the given type and should return an integer value.
Methods used in Comparator
Sure! Here is the updated table with the method descriptions and syntax:
Method Name | Description | Syntax |
---|---|---|
compare() | Compares two objects and returns an integer value indicating their ordering. | int compare(T o1, T o2); |
equals() | Checks if two comparators are equal in their ordering. | comparator1.equals(comparator2); |
comparing() | Creates a comparator based on a specified keyExtractor function. | Comparator comparing(Function keyExtractor); |
thenComparing() | Chains comparators to apply a series of sorting sequences. | Comparator thenComparing(Function keyExtractor, Comparator keyComparator); |
The descriptions are concise and focus on the primary purpose of each method, and the syntax is provided for each method as well.
Example of Comparator in Java
Consider our second and third use case, GET /superheroes?sort=name and GET /superheroes?sort=age that returns superheroes ordered by name and age in ascending order, respectively. We already overridden the compareTo() method to sort SuperHero objects by id. So, it is not possible to use it to sort by name or age. Here comes the lifesaver Comparator that allows us to create custom comparators. Example: nameComparator, ageComparator.
Output
Explanation We created nameComparator and comparator, which sort SuperHero objects by name and age, respectively. The comparators are created using lambda expressions because Comparator is a functional interface.
A functional interface is an interface that contains exactly one abstract method. It is also called Single Abstract Method (SAM) Interfaces. It can have any number of default methods. Use this Free Java Online Compiler to compile your code.
Conclusion
- Comparable is an interface in Java that enables comparing an object with other objects of the same type.
- Comparable is used for the default ordering of the objects.
- Classes implement Comparable interface and overrides the compareTo() method to enable default ordering.
- Comparator is a functional interface in Java that can sort objects. It is used for the custom ordering of objects.
- Comparator provides the compare() method that can be overridden to provide the custom comparison logic.