Continue Statement in Java
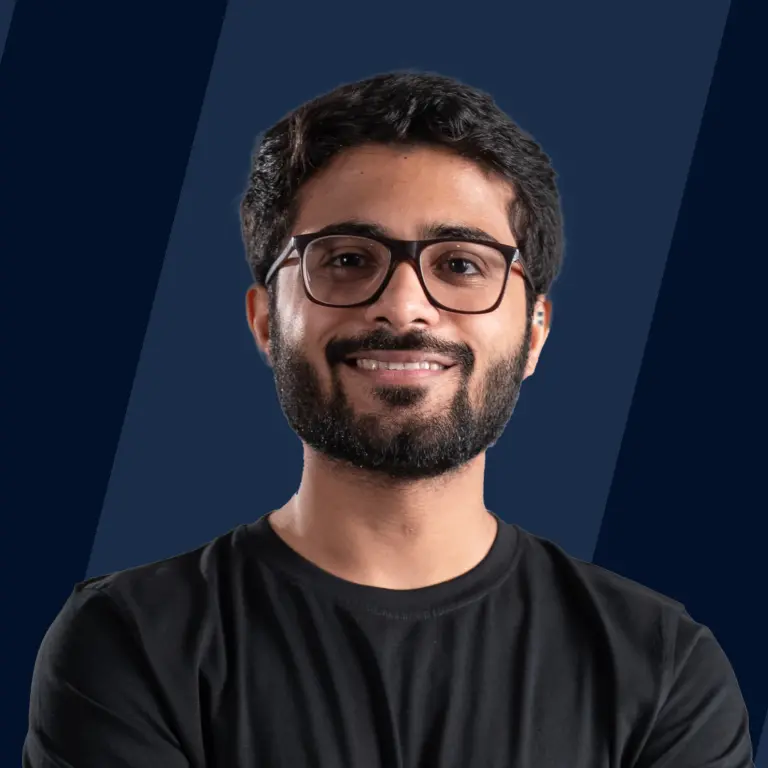
The continue statements in Java are used to control the flow of code. The continue statement in Java terminates one iteration of a loop, by skipping the instructions after it, and begins with the next iteration, i.e., “continue” allows you to skip specific iterations of a loop without completely halting it.
Introduction to Continue Statement in Java
Let’s say, we want to execute a loop in some code, but at a certain step, we want to skip the latter code and want to go to the next iteration, we can use the "continue" statement. Our goal to do this might be an overall better complexity or getting the correct flow of code. The "continue" statement acts similarly in Java, C++ and C. Example of a situation where "continue" can be handy is - printing non-prime numbers in a range of 1 to n. For every number in the loop, we can check if it is prime then we continue the loop, else we print the number.
Working of Java continue statement
The “continue” statement is generally used in loop blocks. Until the "continue" statement is encountered, all lines are executed, and after it, the program skips the rest lines of the loop and goes to the next iteration. When we wish to skip based on a condition and proceed with the rest of the execution, we utilize the continue statement. The continue statement in Java may be used in any form of a loop, although it is most commonly used in for, while, and do-while loops.
The "continue" statement forces control to go to the update statement immediately when using a for a loop. Control quickly goes to the Boolean expression in a while loop or do-while loop.
- We can also label the “continue” statement, it will be discussed later in this article.
Flow Chart of "continue" statement in Java
This is a flow chart of the "continue" statement.
The flow of the loop is such that if the condition having "continue" is false, it continues the iteration. If the condition becomes true, the iteration is terminated, and the control goes back to the next iteration.
We can see that if the "continue" statement is executed, it gives control back to the loop condition (or the update statement in case of for loop).
Syntax
continue;
Examples of using the "continue" statement in Java Loops:
Example 1: for loop
Output:
Explanation: At i = 3, the "continue" statement is skipping the print statement after it, and is giving back the control to the update statement (i++).
- Remember that in the case of for loop, the “continue” statement skips the current iteration and gives the control back to the update statement.
Example 2: while loop
Output:
Explanation: At i = 3, the "continue" statement is skipping the print statement after it, and is giving back the control to the while loop.
- Notice that updating is done before the “continue” statement, otherwise, we will end up with an infinite loop
Example 3: Do-while loop
Output:
- Again, we have incremented the value of ‘i’ before the “continue” statement, otherwise, we would end up with an infinite loop
Other Examples:
Let's try a problem. Let's say we want to compute the sum of the first 5 positive elements in an array:
Output:
Explanation:
We are checking for every i, if the array element is negative or the counter is 5, we are skipping the iteration using continue. Hence, we end up with "sum" having the sum of 2, 4, 6, 8 and 10. Upon adding 10, the counter turns 5, and in the next iterations, even if the array element is positive, the sum is not updated. This is achieved with counter == 5 condition.
- Note: continue is a pre-defined keyword in Java and therefore, it cannot be used as the name of a variable.
Real Life Scenario of using the "continue" statement:
Let’s say due to the COVID pandemic, we want to call students on alternate days and in groups. We want to divide students based on their roll numbers, even in one group and odd in the other. We have 4 working days in a week, starting from Day 0 to Day 3, and we will call students with odd roll numbered students on even days and even roll numbered students on odd days. We want to print the roll numbers of students called day wise.
Output:
Explanation: We are storing the remainder of the day number when divided by 2 in "rem". If the "rem" is equal to the remainder of j divided by 2, we know that both, roll number and day number have the same odd/even parity. And so we skip printing that roll number.
The “continue” Statement in Java with Nested Loops:
We might find ourselves using the "continue" statement in nested loops. When we have nested loops, the “continue” statement controls the next iteration of the nearest loop to which it belongs. It will be better explained with an example.
Output:
Explanation: We can observe that the inner continue (executed at j = 1) skips the current iteration of the while loop. The outer continue (executed at i = 2) skips the current iteration of the for loop, thereby skipping the while loop and print statements altogether. Hence, we can conclude that the “continue” statement skips the code of the block in which it is called.
Labelled Continue Statement in Java
Labelled continue means to give a name to the desired loop. After labelling the desired loop, we can skip iteration to it from any of its inner loops. Unlike many languages, Java does not support the "go to" statement. As the name suggests, the "go to" statement helps us to jump to a line in code forcibly. We can also use the labelled continue as an alternative to the "go to" statement. It would be very helpful if we can skip the current iteration of the outer loop from the inner loop. To tackle this, Java Development Kit 1.5 introduced the concept of the labelled continue.
Syntax:
We have to label the desired loop with a name. While using labelled continue, we have to use the name.
This continue statement will skip the current iteration of the loop labelled with the respective label.
Example of Labelled continue statement
Output:
Explanation: At j = 1 and any value of i, the current iteration of the inner loop is skipped. At j = 2 and any value of i, the current iteration of the outer loop is skipped; due to that, the value of j never proceeds 2.
When to use Continue Statement in Java?
The "continue" statement in Java is used when we want to control a loop so that we can immediately proceed to the next iteration upon satisfying a particular condition. We can use the continue statement to direct the flow of loops. It can be used in all types of loops (for, while, or do-while loops).
Conclusion
- Continue in Java is a loop control statement.
- The continue statement helps us to skip the current iteration of a loop forcibly.
- Syntax: continue <label(if any)>;
- The continue statement can also be used in nested loops. If required, we can label it.
- If not labelled, the continue Java statement skips the current iteration of the loop it is called.
- The continue can be used in any loop (for, while or do-while loops).
- The "continue" statement applies when we want to print the non-prime numbers in a range. We can continue whenever we encounter a prime number from 1 to n in the loop.