Delete a File using Java IO Streams
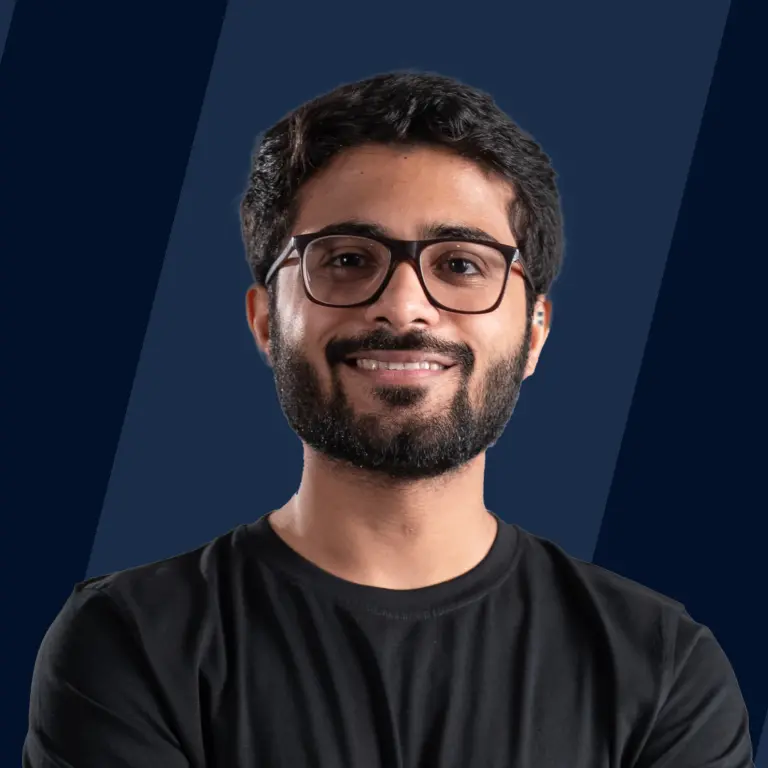
In Java, file deletion is handled by two methods: File.delete() from java.io and Files.deleteIfExists() from java.nio.file, with the latter throwing exceptions. Java's deletion permanently removes files, bypassing the recycle bin.
Method 1: Using File.delete() Method
The File class in java.io package is used to perform various operations on files such as createNewFile(), exists(), canWrite(), canRead(), etc. To delete a file using the File class, we use its delete() method. This method doesn't take any parameters, and it returns a boolean. Let us note down a few important points regarding the delete() method in the File class.
Exceptions
- SecurityException – If a security manager exists and its SecurityManager.checkDelete(java.lang.String) method denies delete access to the file
Important Points
- It is used to delete both files and empty directories. File.delete() method can delete empty directories only.
- Returns true if and only if the file or directory is successfully deleted; false otherwise.
- Throws only one exception, SecurityException, for the reasons mentioned above.
Syntax:
Let us look at an example:
Output:
Explanation:
- In the above example, we are importing the java.io package, which contains the File class.
- In the main() method of the class, we create temp_file object of the File class and pass the address of the file to be deleted.
- The file can be of any format or extension. In the if statement, we call the delete() method of the File class using the temp_file object.
- If the delete() method returns true, we print the successful custom message.
- Else, if it returns false, we print that the file was not deleted.
The File.delete() method cannot delete a file:
- If its path is wrong or corrupted
- Proper delete access is not available to the user
- Some I/O Stream error has occurred
Note:
As for deleting a file, we neither use the FileWriter class (used for writing to a file) nor the Scanner class (used for reading from a file); there's no need to close the stream using the close() method. FileWriter and Scanner classes require the closing of the file before exiting.
Method 2: Using Files.deleteIfExists(Path path) method
Files.deleteIfExists(Path path) is a boolean method defined in java.nio.file package. This method deletes a file mentioned in the path if it exists. It also deletes a directory in the path only if the mentioned directory is empty.
Syntax:
It takes a path as a parameter, the path to the file/directory to be deleted. It returns true if the file was deleted by this method; false if the file could not be deleted because it did not exist.
The file couldn't be deleted due to various reasons:
- The file is not present in the path, or the path is invalid/does not exist.
- I/O Stream error while deleting the file.
Exceptions
This method throws three types of exceptions to show various scenarios:
- DirectoryNotEmptyException - If the path is a directory and could not be deleted because the directory is not empty
- IOException - If an I/O error occurs
- SecurityException - In the case of the default provider, and a security manager is installed, the SecurityManager.checkDelete(String) method is invoked to check to delete access to the file.
Let us now see an example:
Output:
Explanation:
- In the above example, we import java.nio.file and java.io.IOException packages and create a Main named class.
- In the main() method of this class, we have put try and catch blocks as our desired method deleteIfExists() throws exceptions.
- We first try to delete the file using the method and store the result in the isDeleted variable of the boolean type.
- If the isDeleted is true, we print a successful completion message; else, we print an unsuccessful custom message.
- If the method throws an exception, we are catching them in the various catch blocks and displaying suitable information.
Conclusion
- To delete a file using Java, you can use two methods i.e. File.delete() Files.deleteIfExists(path) methods defined in java.io and java.nio.file packages respectively.
- delete() method doesn't throws SecurityException and returns a boolean. If the file is deleted successfully, it returns true else; it returns false.
- deleteIfExists() method throws an exception in case of I/O errors, or the given path's directory is non-empty. It also returns a boolean, true if the file is deleted. Otherwise, it returns false.
- Both Files.delete() and Files.deleteIfExists() can delete only empty directories.