Difference between Abstract Class and Interface in Java
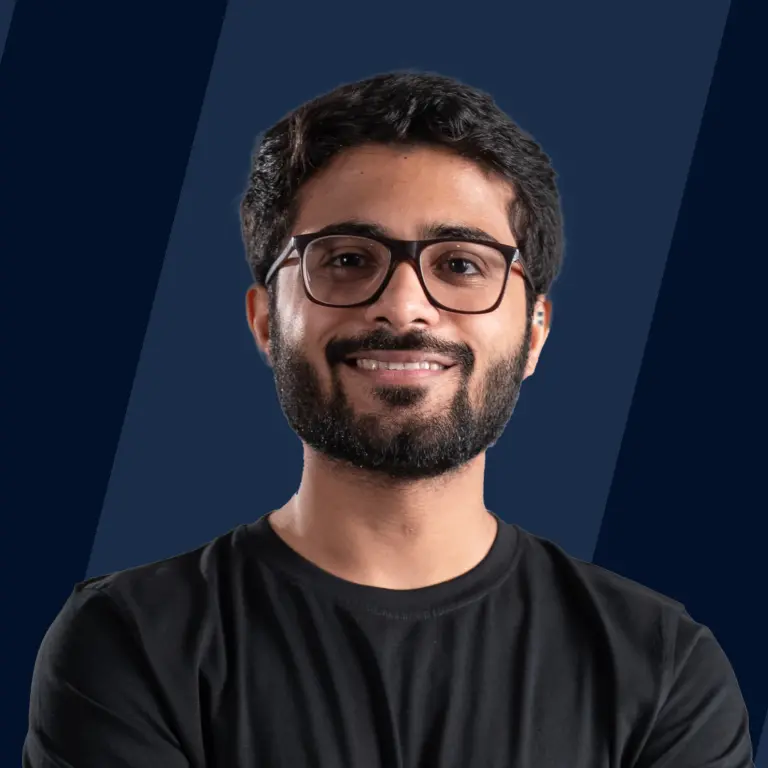
The main difference between Abstract Class and Interface in Java is that an abstract class can have both abstract and concrete methods, while an interface can only have abstract methods.
Additionally, abstract classes can have instance variables, constructors, and static methods, whereas interfaces cannot contain any implementation details. Furthermore, a class can extend only one abstract class, but it can implement multiple interfaces.
The difference between abstract class and interface lies in their implementation details and usage scenarios.
An abstract class in Java is a class that cannot be instantiated and may contain abstract methods, which are declared but not implemented. It serves as a template for other classes to extend and provides common functionality to its subclasses.
On the other hand, an interface in Java is a reference type that contains only abstract methods, constants, and nested types. It defines a contract for classes to implement, specifying a set of methods that must be implemented by implementing classes. Interfaces allow for multiple inheritance in Java, as a class can implement multiple interfaces.
Abstract class vs interface:
Abstract class | Interface |
---|---|
Limits the implementing class to extend atmost one abstract class | Allows a class to implement multiple interfaces that simulate the effect of multiple inheritance |
Abstract classes can have abstract and non-abstract methods. | Interfaces can only have abstract, static and default methods. |
Supports non-final, final, non-static, and static methods and attributes | Supports only final and static methods and attributes |
Abstract classes can extend one abstract class and implement multiple interfaces. | Interfaces can only extend other interfaces. |
Can be inherited by other classes. | Can be implemented by classes and other interfaces. |
Can have constructors. | Cannot have constructors. |
An abstract class can provide the implementation of an interface. | An interface cannot provide the implementation of an abstract class. |
Cannot be instantiated. | Can be instantiated. |
Subclasses extend an abstract class using the extends keyword. | Classes implement interfaces using the implements keyword. |
[public] abstract class AbstractClass{} | [public] interface InterfaceName{} |
[public] class Concrete extends AbstractClass{} | [pubilc] class Concrete implements Interface1, Interface2, ..., InterfaceN {} |
Example of Abstract Class and Interface in Java
Let's see an example where we will be using both Abstract Class and interface in Java.
Output:
Conclusion
- Abstract classes and interfaces both serve as blueprints for creating classes in Java.
- The difference between them lies in their implementation details and usage scenarios.
- Abstract classes can have both abstract and concrete methods, while interfaces can only have abstract methods.
- Abstract classes are used to provide a common base for related classes, while interfaces define a contract for classes to implement.
- Choosing between abstract classes and interfaces depends on the specific requirements of the design and the level of abstraction needed in the application.