Difference Between Data Hiding and Abstraction
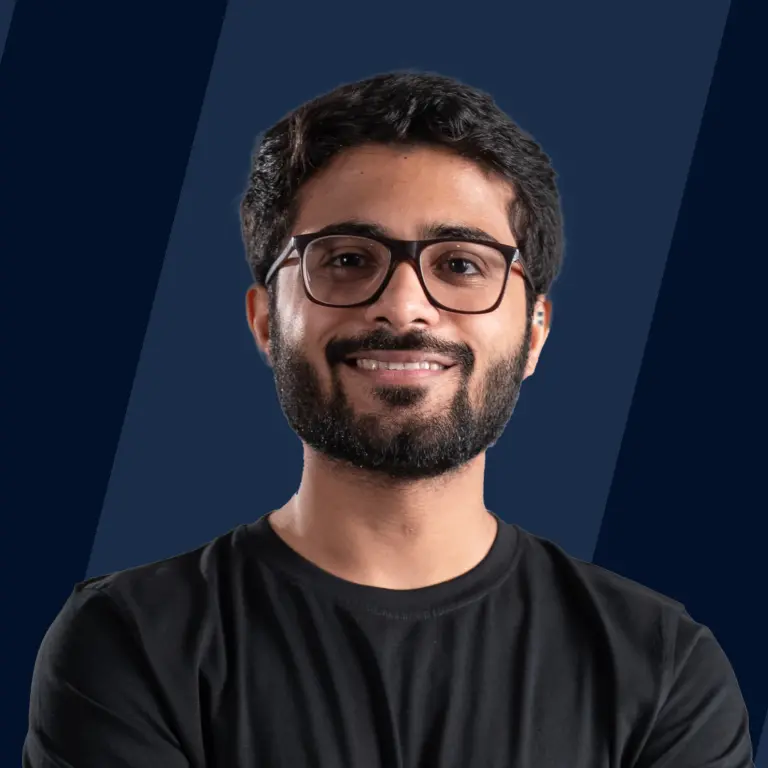
Overview
Data Abstraction and Data Hiding concepts in OOP are used to show only the required information to the end-user and hide the unnecessary details but for distinct purposes such as reducing the system's complexity to make it more user-friendly.
Abstraction is used to display only the important set of services or functionalities to the users and hide the internal implementation details of the code or software. Data Hiding, on the other hand, is the process that hides the internal data and restricts it from directly getting accessed by the program for unauthorized access. It is achieved by using private and protected access specifiers.
Introduction
Java is an object-oriented programming language. Object-Oriented Programming (OOP) is a paradigm that helps to create solutions to real-world scenarios easily. Data Hiding and Data Abstraction are two important concepts of object-oriented programming. However, both seem very similar but are totally different in terms of concept and implementation, which we will cover in detail in this article.
Abstraction hides the internal implementation details and displays only the important services or functionalities to the outside world while Data Hiding is used to hide internal data from the outside world so that it can not be directly accessed by the outside world/classes.
What is Abstraction?
Abstraction is a process of hiding all the unnecessary implementation details and exposing only the required functionalities. Like we all use a laptop for creating documents, internet browsing, playing games, etc., in our daily life. But we don’t know what things are happening inside the laptop when we turn it on to perform any kind of operation.
Also, when we need to send SMS from our mobile, we only type the text and send the message. We don’t know the internal process of the message delivery. That's how Abstraction works in daily life, as all the unnecessary details are hidden.
In Java, Data Abstraction is defined as the process of reducing the object to its essence so that only the necessary characteristics are exposed to the users. Abstraction defines an object in terms of its properties which are the attributes, their behavior which is defined as methods, and interfaces which acts as means of communicating with other objects.
Data Abstraction is achieved by using the abstract classes and interfaces in Java programming.
For better understanding, let us understand abstract classes and interfaces as well.
Abstract Class
An abstract class in Java is a restricted class that cannot be used to create objects as it cannot be instantiated. To access it, it must be inherited or implemented by another class. An abstract class is created using the abstract keyword. It may or may not contain abstract methods.
Abstract Methods
An abstract method is a method that is declared without an implementation (without braces) and it is followed by a semicolon, as given below:
If a class includes abstract methods, then the class itself must be declared abstract, as in:
When an abstract class is inherited, the inherited class usually provides implementations for all of the abstract methods in its parent class. However, if it does not implement all of them, then the inherited class must also be declared abstract.
Interfaces
An interface is a reference type, similar to a class, that can only contain constants, method signatures, default methods, static methods, and nested types. Method bodies exist only for default methods and static methods. Interfaces cannot be instantiated — they can only be implemented by classes or extended by other interfaces.
Methods in an interface that are not declared as default or static are implicitly abstract, which means the abstract modifier is not used with the methods declared in interfaces.
Defining an interface as below:
Note:
Abstract method signatures have no braces and are terminated with a semicolon.
To use an interface, we write a class that implements the interface.
As we have seen in the above examples, the abstract method signalTurn or turn is exposed in the interface. But its method implementation is hidden in the other class.
Likewise, in real life, we only turn on/off the indicator, but its working and implementation are hidden.
Abstraction Types
There are basically three types of abstraction:
Procedural Abstraction
It is a model of what we want a subprogram to do (but not how to do it). It provides mechanisms for calling well-defined procedures or operations as entities.
It is when we write code sections (static methods) that are generalized by having variable parameters. The idea is that we have code that can cope with various situations, depending on how its parameters are set when it is called. It makes a program shorter and easier to understand and helps to debug since when we correct a procedure, we correct the program for all the cases where the procedure is called.
So we achieve abstraction through classes by a series of procedures in form of functions followed by one after another in sequence.
Data Abstraction
It is the type of abstraction in which we create complex data types and hide their implementation details from the users. The benefit of this approach is that it solves the performance issues and improves the implementation over time. If we make any changes to improve the performance, it does not reflect on the code present on the client side.
In data structures such as HashMap or HashSet, we hide their implementation details from the users and display only the meaningful operations to interact with the data. So abstraction is achieved from a set of data that describes an object.
Control Abstraction
It is the software part of abstraction where the program is simplified and unnecessary execution details are removed.
Control abstraction is the process of determining all such statements which are similar and repeated many times and exposing them as a single unit of work. It follows the basic rule of DRY code, which means Don’t Repeat Yourself, and using functions in a program is the best example of control abstraction.
What is Data Hiding?
Suppose you have an account in the bank. If your balance variable is declared as public in the bank software, your account balance will be known publically. In this case, anyone can know your account balance which obviously you don't want.
So, the balance variable is declared as private to make your account safe so that anyone cannot see your account balance. The person who has to see his account balance will have to access private members through methods defined inside that class, and these methods will ask for the account holder's name, user id, and password for authentication. Thus, we can achieve security by utilizing the concept of data hiding.
So, Data Hiding is the process that hides the data from the components of the program that don't need to be retrieved. It ensures exclusive data access to class members and provides object integrity by preventing unintended or intended changes.
Access Modifiers in Java
In the Java programming language, we have access modifiers like public, private, and protected. The other class objects can access the public methods and data members. The protected members are accessible by the object of the same class and its subclasses. However, the private members are accessible within the class. Hence, the members are secured with the help of private access modifiers. The scope of member variables to be accessed outside a class is as follows:
Modifier | Same Class | SubClass | Package | Global |
---|---|---|---|---|
public | Yes | Yes | Yes | Yes |
protected | Yes | Yes | Yes | No |
private | Yes | No | No | No |
default | Yes | No | Yes | No |
Getters and Setters:
When it is necessary to restrict objects of other classes from accessing the members of another class, we can use the private access modifier. Then, the data members are only accessible within the class. To make the data members private and also accessible outside by the other classes, we use getters and setters methods.
How to Achieve/Implement Data hiding?
As we have discussed, Data Hiding is achieved using the concepts of getters and setters methods. So members are private, and getters and setters are public functions to access and update private attributes of the objects. Here, we will discuss in detail about the implementation.
When the class fields are declared private, then getters and setters are used to access and manipulate their values of them. Public access specifiers are used with the getter and setter methods for accessibility.
Let's see with an example:
Output:
Explanation:
- In the above example, we have created a Student class in which all the private member variables are declared and can not be accessed directly outside the Student class.
- For accessing and modifying the values, getters and setters are created with public access modifiers. By this, we are hiding the data and only using the getter-setter methods, which enhances the security as the private member variables will not be accessed directly.
- We can also put some conditions for setting the values as required. In this example, we are checking if the name is null or its length is 0. If either of the conditions is true it will throw an exception.
Difference Between Data Hiding and Abstraction
Abstraction | Data Hiding |
---|---|
It is the process of hiding the internal implementation and keeping the complicated procedures hidden from the user. Only the required services or parts are displayed. | It is the process that ensures exclusive data access to class members and hiding the internal data from outsiders. |
Focuses on hiding the complexity of the system. | Focuses on protecting the data by achieving encapsulation (a mechanism to wrap up variables and methods together as a single unit). |
This is usually achieved using abstract class concept, or by implementing interfaces. | This can be achieved using access specifiers, such as private, and protected. |
It helps to secure the software as it hides the internal implementation and exposes only required functions. | This acts as a security layer. It keeps the data and code safe from external inheritance as we use setter and getter methods to set and get the data in it. |
It doesn’t affect end users, since the developers can perform changes internally in implementation classes without changing the abstract method in the interfaces. | This ensures that users can’t access internal data without authentication. |
It can be implemented by creating a class that only represents important attributes without including background detail. | Getters and setters can be used to access the data or to modify it. |
Key Differences between Abstraction and Data Hiding
- Abstraction shows only the essential information and hides the non-essential details. On the other hand, data hiding is used to hide the data from the components of the program by ensuring exclusive data access to class members.
- The abstraction's purpose is to hide the complex implementation details of the program or software. On the other hand, data hiding is implemented to achieve encapsulation.
- Abstraction is used in class to create a new user-defined data type. On the contrary, in classes, data hiding is used to make the data private.
- In abstraction, abstract classes and interfaces are used, whereas in data hiding, getters and setters are used for the implementation.
- Abstraction focuses on the observable behavior of the data, whereas data hiding restricts or allows the use of data within a capsule.
Conclusion
- Data hiding and abstraction are two concepts in OOP which help in hiding unnecessary details and expose only required information to the end users.
- Abstraction is the concept of hiding internal implementation details and expose only the necessary functionalities or apis to the end users.
- Data Hiding is the concept of restricting access to class and instance variables to improve the security aspect of the applications.
- Data hiding secures the data members. On the other hand, abstraction helps to reduce the complexity of the system.
- Data hiding is used for attaining Encapsulation whereas Abstraction limits access to the internal details and show the essential features.
- Abstraction is achieved by using the abstract classes and interfaces. And Data Hiding is achieved by using private and protected access specifiers.