throw and throws in Java
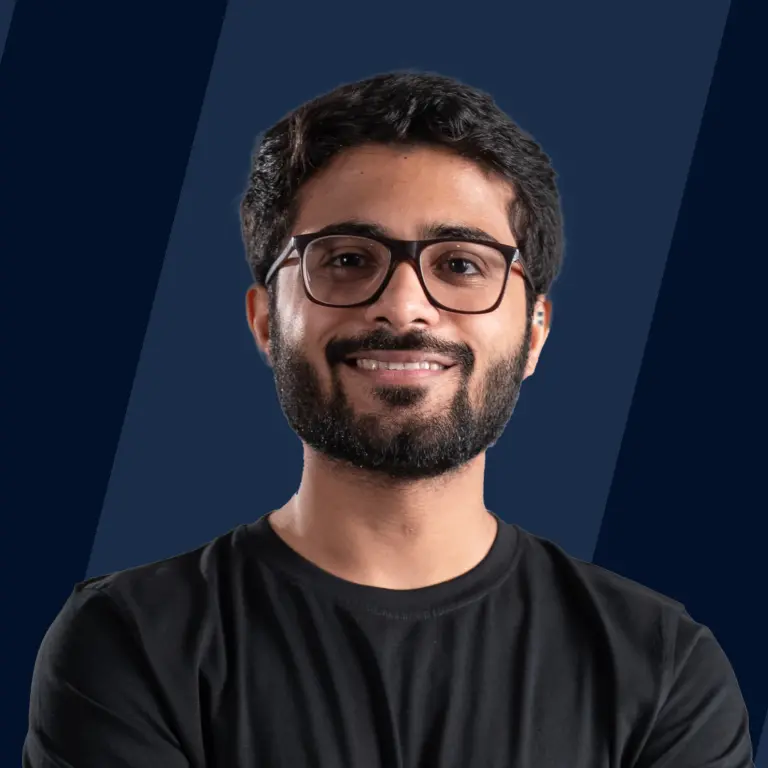
Java Exception Handling preserves the regular flow of applications by managing runtime errors like ClassNotFoundException, IOException, SQLException, and RemoteException. throw and throws in Java are used to handle exceptions. "throw" is used to manually throw an exception, while "throws" is used in method signatures to declare potential exceptions that might be thrown during execution.
Java Throw
In Java, the throw keyword is used to deliberately raise exceptions within code. It can be employed to throw both checked and unchecked exceptions, but its primary utility lies in throwing custom exceptions, aiding in better error handling and code organization.
- Checked Exception: This is an exception that occurs at the time of compilation and is also called Compile-time Exceptions. These exceptions must be handled using some exception handling mechanism in the program otherwise the program gives compile-time errors.
- Unchecked Exception: These occur at the time of execution. These are also called Runtime Exceptions. Runtime exceptions are ignored at the time of compilation. For example, invalid operations like division by zero, accessing an array with an index bigger than its size, etc are all actions that are all runtime/unchecked exceptions.
Syntax
The syntax of the throw keyword is, keyword throw followed by the instance of the exception that needs to be raised. Below is the syntax:
Java Throw Examples
Let us dive into the practical usage of throw keyword in Java language:
Here, we can understand how the keyword actually worked and understand how the flow works through examples.
Example 1: Throwing custom exception while trying to check valid driving age
In the above example, initially, declared UnableToDriveException which is the custom exception class and it just extends Exception class calling the parent constructor and returning string using super().
In CustomTestException class, we take age as input and checking with minimum age for driving and if fails, will throw UnableToDriveException using throw keyword.
Input:
Output:
Example 2: Throwing "divide by 0" Arithmetic exception
Output:
Java Throws
In Java, the throws keyword is incorporated into a method's signature to signify the potential occurrence of certain types of exceptions within that method. This declaration serves as a warning to callers, indicating that they must handle any potential exceptions that might be thrown by the method. To manage these exceptions, callers typically enclose method calls within a try-catch block, ensuring robust error handling and program stability.
Syntax
The syntax of the throws keyword is, keyword throws followed by class names of the exceptions.
Java Throws Examples
Below we have listed some common examples for which Java throws keyword is used in order to handle exceptions properly.
Example 1: Java throws Keyword
Above code will take two integers from the user and print their division. During division, if the user inputs a second integer as 0 then an exception message will be thrown by the system as division by zero is not possible.
If the user inputs a second integer apart from 0 then the code will run normally exceptions will not occur and the system will print the result of the division on the screen.
Example 2: Throwing multiple exceptions
Above code will give the division of the numbers provided by the users. It takes three values from the user,
- Dividend as a first number
- Divisor as a second number
- Length of the array as a third number
Case 1: If the user gives appropriate numbers for division and length of the array of more than 3 as input parameters, the program will run correctly and print the result of division on the output screen.
Case 2: If the user gives the second number as 0 and/or the length of the array is less than 3 then our custom method will throw exceptions and will be caught by the main() method. As all the exceptions in Java extend Throwable class e.g. ArithmeticException, ArrayIndexOutOfBoundsException will be caught by the catch block.
Example 3: Handling Multiple exceptions using throws
The above code will give the division of the numbers provided by the users. It takes three values from the user,
- Dividend as a first number
- Divisor as a second number
- Length of the array as a third number
Case 1: If the user gives appropriate numbers for division and length of the array of more than 3 as input parameters, the program will run correctly and print the result of division on the output screen.
Case 2: If the user gives the second number as 0 and the length of the array as more than 3 as input parameters, our custom method - ThrowsExample will throw an Arithmetic Exception (because division by zero is not possible mathematically) and will be caught by the main() method.
Case 3: If the user gives appropriate numbers for division but the length of the array is less than 3 as input parameters, our program will be able to do division correctly but as we are storing our result of division in the 3rd index of the array, our custom method - ThrowsExample will throw ArrayIndexOutOfBounds Exception (because our array has not enough space in order to save our division result) and will be caught by the main() method.
Important Points to Remember about Java throws Keyword
- The "throws" keyword is essential for handling checked exceptions, indicating potential errors that must be addressed during compilation.
- Its usage does not prevent unexpected program termination but rather serves as a communication tool to inform the caller of potential exceptions.
- By employing the "throws" keyword, developers can provide clarity to callers regarding the potential exceptions that may occur within a method.
Conclusion
- In this article, we explored the throw and throws in Java.
- We also learned about checked and unchecked exceptions in Java.
- Java program to handle unchecked exceptions ArrayIndexOutOfBoundsException, NumberFormatException using throw keyword.
- Java program to propagate an Unchecked Exception like NullPointerException using throw keyword.
- Java program to handle checked exception ClassNotFoundException using throws keyword.
- The combined application of throw and throws in Java contributes to the robustness and reliability of Java applications by facilitating precise error signaling and ensuring proper error handling throughout the codebase.